Question
Write a program that reads in a sequence of characters entered by the user and terminated by a period ('.'). Your program should allow the
Write a program that reads in a sequence of characters entered by the user and terminated by a period ('.'). Your program should allow the user to enter multiple lines of input by pressing the enter key at the end of each line. The program should print out a frequency table, sorted in decreasing order by number of occurences, listing each letter that ocurred along with the number of times it occured. All non-alphabetic characters must be ignored. Any characters entered after the period ('.') should be left in the input stream unprocessed. No limit may be placed on the length of the input. Use an array with a struct type as its base type so that each array element can hold both a letter and an integer. (In other words, use an array whose elements are structs with 2 fields.) The integer in each of these structs will be a count of the number of times the letter occurs in the user's input. Let me say this another way in case this makes more sense. You will need to declare a struct with two fields, an int and a char. (This should be declared above main, so that it is global; that is, so it can be used from anywhere in your program.) Then you need to declare an array (not global!) whose elements are those structs. The int in each struct will represent the number of times that the char in the same struct has occurred in the input. This means the count of each int should start at 0 and each time you see a letter that matches the char field, you increment the int field. Don't forget that limiting the length of the input is prohibited. If you understand the above paragraph you'll understand why it is not necessary to limit the length of the input. The table should not be case sensitive -- for example, lower case 'a' and upper case 'A' should be counted as the same letter. Here is a sample run: Enter a sequence of characters (end with '.'): do be Do bo. xyz Letter: Number of Occurrences o 3 d 2 b 2 e 1 Note: Your program must sort the array by descending number of occurrences. You may use any sort algorithm, but I would recommend using the selection sort from lesson 9.6. Be sure that you don't just sort the output. The array itself needs to be sorted. Don't use C++'s sort algorithm. Submit your source code and some output to show that your code works. Hints: You will want to be familiar with various C++ functions for dealing with characters such as isupper, islower, isalpha, toupper, and tolower. You should assume for this assignment that the ASCII character set is being used. (This will simplify your code somewhat.) Note that your struct should be declared above main(), and also above your prototypes, so that your parameter lists can include variables that use the struct as their base type. It should be declared below any global consts. You will not need to read the user's input into an array or a c-string or a string. The only variables you will need are the array of structs described above, one single char variable to store each single character as it is read, and perhaps a couple of loop counters. For some students this may require a deeper understanding of how the extraction operator works. For example, if you put the extraction operator in a loop, you can read a sequence of characters. There doesn't have to be a separate prompt or a separate press of the "enter" key for each use of the extraction operator. Consider this code: #include using namespace std; int main() { char ch; int count = 0; cout << "Enter a bunch of text, ending with a ?" << endl; cin >> ch; while (ch != '?') { count++; cin >> ch; } cout << "There were " << count << " characters." << endl; if (cin.peek() != ' ') { cout << "The following characters were entered after the '?': "; cin.get(ch); while (ch != ' ') { cout << ch; cin >> ch; } cout << endl; } } This code will count the number of characters the user enters before typing "?". The user can hit the enter button as many times as they want, and the loop will keep reading characters until it encounters a '?'. Furthermore, if there are additional characters typed AFTER the ?, they will be left in the input stream (cin) for the next time it is used. The above program demonstrates this by reading those remaining characters and printing them. I strongly suggest that you copy this code and try it out. Try typing a few lines of text before entering a question mark, and type a few characters after the question mark (on the same line) before hitting enter. More hints about how this will work: The user enters a bunch of characters then hits the enter button. When the user hits the enter button, those characters go into the input stream. THEN cin >> ch; reads the first character from the input stream, then it is counted, then the second time through the loop the second character is read from the input stream and counted, then the third time through the loop the third character is read from the input stream and counted, and so on until the loop condition becomes false or all of the characters that the user entered have been read. At that point, if all of the characters have been read, then the cin.get(ch) causes the program to stop and wait for more characters to be entered. If the loop has exited, then any remaining characters that the user entered will stay in the input stream, so that next time you do a cin.get(ch) or cin >> ch, those characters will be read. One last way of saying this: If the user enters several characters, but does not enter '.', and then hits the enter key, the loop should process all the characters entered, and then just wait for more characters, until it finally sees a '.'
Step by Step Solution
There are 3 Steps involved in it
Step: 1
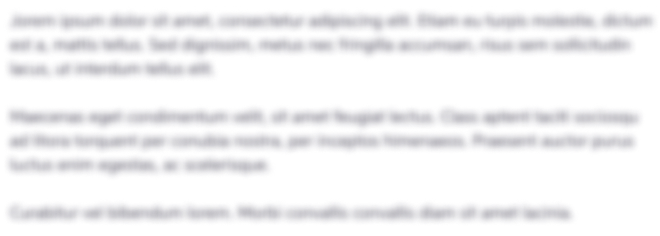
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started