Question
Write a program that uses the Coin class. The program should have three instances of the Coin class: one representing a quarter, one representing a
Write a program that uses the Coin class. The program should have three instances of the Coin class: one representing a quarter, one representing a dime, and one representing a nickel. When the game begins, your starting balance is $0. During each round of the game, the program will toss each of the simulated coins at one time. When a tossed coin lands heads-up, the value of the coin is added to your balance. For example, if the quarter lands heads-up, 25 cents is added to your balance. Nothing is added to your balance for coins that land tails-up. The game is over when your balance reaches one dollar or more. If your balance is exactly one dollar, you win the game. If your balance exceeds one dollar, you lose.
I already have the code for this program (posted below). I need the following additions:
In the coin class I need to add the following:
- A private static double named balance. The balance will hold the balance, or total value, of all the coins tossed.
- A void member function named addBalance that will add the value field to the balance field.
- A static double member function getBalance that will return the balance field.
The Coin class should act as the base class. Three child classes will be created using the Coin class as the base class. The classes will be Quarter, Dime, and Nickel. These child classes will contain a constructor that will be used to set the value field to the value of their amount (Quarter = 0.25, Dime=0.10, Nickel=0.05)
Instead of using the separate counter variable to keep the balance of the coins, the static field balance should keep track of the balance of the coin tosses.
In the main, create a function called evaluateToss that will take a Coin reference pointer parameter. Use this method to toss the coin, decide if the coin is showing heads or tails, and add the value to the balance if needed. Pass the Quarter, Dime, and Nickel objects to this function each time to do the work.
The main goal is to use inheritance. The program should include as many methods of inheritance as possible.
Here are the program files:
Source.cpp
#include "Coin.h"
int main() { srand(time(0)); Coin quarter(25), dime(10), nickel(5); double balance = 0; double add_value;
while (balance < 1) { quarter.toss(); cout << quarter.getSideUp() << ": "; add_value = quarter.getValues() * quarter.getHeads(); balance += add_value; cout << "Balance = " << balance << " " << endl;
if (balance >= 1) { break; }
dime.toss(); cout << dime.getSideUp() << ": "; add_value = dime.getValues() * dime.getHeads(); balance += add_value; cout << "Balance = " << balance << " " << endl;
if (balance >= 1) { break; }
nickel.toss(); cout << nickel.getSideUp() << ": "; add_value = nickel.getValues() * nickel.getHeads(); balance += add_value; cout << "Balance = " << balance << " " << endl;
if (balance >= 1) { break; } }
if (balance > 1) { cout << "You lost the game. Balance = " << balance << endl; } else { cout << "You won the game! Balance = " << balance << endl; }
return 0; }
Coin.h
#ifndef DICE_H #define DICE_H #include
using namespace std;
class Coin { private: string sideUp; bool heads; double value;
public: Coin(double _cents = 5, bool _heads = true);
void toss();
bool getHeads();
double getValues();
string getSideUp();
}; #endif
Coin.cpp
#include "Coin.h"
Coin::Coin(double _cents, bool _heads) { if (_heads) { sideUp = "HEADS"; } else { sideUp = "TAILS"; } heads = _heads; value = _cents * 0.01; }
void Coin::toss() { int random = rand() % 2; heads = random;
if (heads == 1) { sideUp = "HEADS"; } else { sideUp = "TAILS"; } }
bool Coin::getHeads(){ return heads; }
double Coin::getValues(){ return value; }
string Coin::getSideUp(){ return sideUp; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
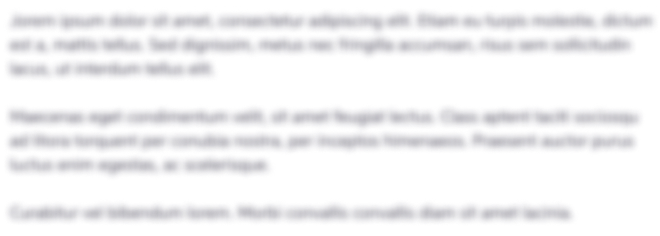
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started