Question
Write a program that will calculate a person's weekly gross pay (gross pay is pay before taxes). A person who is paid by the hour
Write a program that will calculate a person's weekly gross pay (gross pay is pay before taxes).
A person who is paid by the hour receives weekly gross pay based on their pay rate ($/hr.) multiplied by the number of hours worked. In addition, if the person works more than 40 hours, they are paid "time-and-a-half" (1.5x) for hours in excess of 40 hours.
The program will ask the user to enter the pay rate of the person (as a double), followed by the number of hours worked (as a double), and will display the weekly gross pay.
(1) Using a loop, continue to ask for additional pay rates (and hours) until a pay rate of zero is entered. (3 pts)
Ex:
Enter the pay rate (0 to quit): $10.55 Enter the number of hours worked: 12.5 Pay: $131.88 Enter the pay rate (0 to quit): $0
Ex:
Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 12 Pay: $120.00 Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 41 Pay: $415.00 Enter the pay rate (0 to quit): $0
Ex:
Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 12 Pay: $120.00 Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 41 Pay: $415.00 Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 40 Pay: $400.00 Enter the pay rate (0 to quit): $0
(2) Using loops, validate the pay rate is not negative. The validate messages are indented 3 spaces. (3 pts)
Ex:
Enter the pay rate (0 to quit): $-1 Pay rate must be positive. Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 12 Pay: $120.00 Enter the pay rate (0 to quit): $0
Ex:
Enter the pay rate (0 to quit): $-1 Pay rate must be positive. Enter the pay rate (0 to quit): $-5 Pay rate must be positive. Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 12 Pay: $120.00 Enter the pay rate (0 to quit): $0
Ex:
Enter the pay rate (0 to quit): $-1 Pay rate must be positive. Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 12 Pay: $120.00 Enter the pay rate (0 to quit): $-5 Pay rate must be positive. Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 40 Pay: $400.00 Enter the pay rate (0 to quit): $0
(3) Using loops, validate the number of hours worked is greater than zero. The validation messages are indented 3 spaces. (2 pts)
Ex:
Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 0 Hours worked must be positive. Enter the number of hours worked: 12 Pay: $120.00 Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 41 Pay: $415.00 Enter the pay rate (0 to quit): $0
Ex:
Enter the pay rate (0 to quit): $10 Enter the number of hours worked: -1 Hours worked must be positive. Enter the number of hours worked: -5 Hours worked must be positive. Enter the number of hours worked: 12 Pay: $120.00 Enter the pay rate (0 to quit): $10 Enter the number of hours worked: 41 Pay: $415.00 Enter the pay rate (0 to quit): $0
I have already written most of the code but a I am getting the error: Program generated too much output. Output restricted to 50000 characters. Check program for any unterminated loops generating output.
Please help fix.
My code:
#include
int main(void) {
float hours, wage, overtime = 0, regularHours; while (1) { overtime = 0; printf("Enter the pay rate (0 to quit): $"); scanf("%f", &wage); if (wage == 0.0f) { break; } printf(" Enter the number of hours worked: "); scanf("%f", &hours); if (hours <= 40) { regularHours = hours; } else { overtime = hours - 40; regularHours = 40; } printf("Pay: $%.2f ", (regularHours * wage + overtime * wage * 1.5)); }
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
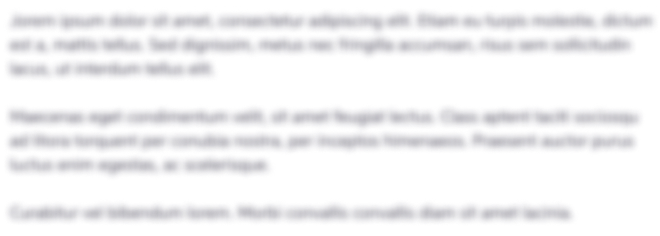
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started