Question
Write a program that will collect from the user a list of temperature samples taken throughout asingle day. Each temperature sample consists of a temperature
Write a program that will collect from the user a list of temperature samples taken throughout asingle day. Each temperature sample consists of a temperature (degrees Fahrenheit) and a time(military time in hours and minutes only). The hours in military time is an integer between 0 and23 and the minutes is an integer between 0 and 59. Your program will display military time inthe format HH:MM, where HH is the hour and MM is the minutes. For example, midnight is00:00 in military time, noon is 12:00, 9:10am in standard time is 09:10 in military time, and 3:15pm in standard time is 15:15 in military time.
The procedure main() (see the code template temps_template.cpp) has been written foryou (DO NOT change anything in the main() procedure) as well as the members (attributes andfunctions) of the Classes Miltime and Fahrenheit. You will write code for the member functions of both classes and also helper functions as indicated by the comments /* INSERTCODE HERE */.Run temps_solution.exe to see an example of the program. A sample run of the program would look like this (bold indicates what the user will enter):
> temps.exe Enter the output file name: out.txtEnter the number of samples: 2 Enter degrees(Fahrenheit): 212
Enter length: 4
Enter height: 27
Enter degrees(Fahrenheit): 32.5
Enter length: 0
Enter height: 1
> cat out.txt
-------------------------------------------------------------------------------------------------
Sample #1: 212 degrees F (or 100 degrees C ) at 04:27
Sample #2: 32.5 degrees F (or 0.277778 degrees C ) at 00:01
------------------------------------------------------------------------------------------------
The average temp is 122.25 degrees F
The coldest temp is 32.5 degrees F
The last sample was taken at time 04:27 To view the contents of the file, type cat out.txt. Your program must work for any file names besides out.txt.
1. The function prototypes are provided for you and must NOT be changed in any way.
2. The procedure main() is provided for you and must NOT be changed in any way.
3. Understand the class definitions for MilTime and Fahrenheit.
4. Understand the algorithm in the procedure main().
5. Do NOT add more functions/procedures to the solution.
6. Each function should have a comment explaining what it does.
7. Each function parameter should have a comment explaining the parameter.
8. Write code by replacing every place /* INSERT CODE HERE */ appears with your solution.
9. Implement the member functions for the class MilTime. Use the appropriate class attributesof the class MilTime in your solution.? Use the provided class attributes in your solution of the class MilTime.10. The member function write_out() of the class MilTime writes the military time in the formatHH:MM to a given file output stream. Write code to ensure this format.11. You may assume that the user will enter only valid values for hours (0-23) and minutes (0-59).12. Implement the member functions for the class Fahrenheit. Use the appropriate classattributes of the class Fahrenheit in your solution.
? Use the provided class attributes in your solution of the class MilTime.? Include the C++ command #include
13. The member function getTemp() of the class Fahrenheit retrieves the degrees stored inFahrenheit.
14. The member function getTime() of the class Fahrenheit retrieves the military time (anobject).
15. The member function getCelsius() of the class Fahrenheit converts the stored degrees inFahrenheit to Celsius and returns this conversion.
16. The member function write_out() of the class Fahrenheit writes a list of the samples andother information to an output file given a file name.? Use the file name to open the file for output. Make sure to pass a C style string, not the C++ string, to the open routine. Be sure to use the type ofstream, not ifstream, for your output file streams. Check if the file was successfully opened for output. If not, print an error message (see sample executable) and exit. 17. These functions are called from the main() function.
? Implement the function read_filename() that prompts, reads, and returns a file nameas a string. Include the C++ command #include
temperature over all of the samples.o Implement the function coldest_temp() that returns the lowest temperaturevalue over all of the samples.
o Implement the function last_sample() that returns the last temperaturesample taken during the day.
PLEASE FOLLOW THIS TEMPLATE:
#include
using namespace std;
class MilTime { private: int hour; int minutes;
public: int getHour() const; int getMin() const; void setHour(const int h); void setMin(const int m); void write_out(ofstream & fout); };
class Fahrenheit { private: double degree; MilTime time;
public: // member functions double getTemp() const; MilTime getTime() const; double getCelsius() const; void setTemp(const double temp); void setTime(const int h, const int m); void write_out(const string fname) const; };
// FUNCTION PROTOTYPES GO HERE: string read_filename(const string prompt); int read_num_samples(const string prompt); Fahrenheit read_sample(const int k); void write_to_file(const string filename, const vector
int main() { // Define local variables string fname; // Output file name int n; // Number of temperature samples vector
int MilTime::getMin() const { /* INSERT YOUR CODE */ }
void MilTime::setHour(const int h) { /* INSERT YOUR CODE */ }
void MilTime::setMin(const int m) { /* INSERT YOUR CODE */ }
void MilTime::write_out(ofstream & fout) { /* INSERT YOUR CODE */ }
double Fahrenheit::getTemp() const { /* INSERT YOUR CODE */ }
MilTime Fahrenheit::getTime() const { /* INSERT YOUR CODE */ }
double Fahrenheit::getCelsius() const { /* INSERT YOUR CODE */ } void Fahrenheit::setTemp(const double temp) { /* INSERT YOUR CODE */ }
void Fahrenheit::setTime(const int h, const int m) { /* INSERT YOUR CODE */ }
string read_filename(const string prompt) { /* INSERT YOUR CODE */ }
int read_num_samples(const string prompt) { /* INSERT YOUR CODE */ }
Fahrenheit read_sample(const int k) { /* INSERT YOUR CODE */ }
void write_to_file(const string filename, const vector
double coldest_temp(const vector
MilTime last_sample(const vector
Step by Step Solution
There are 3 Steps involved in it
Step: 1
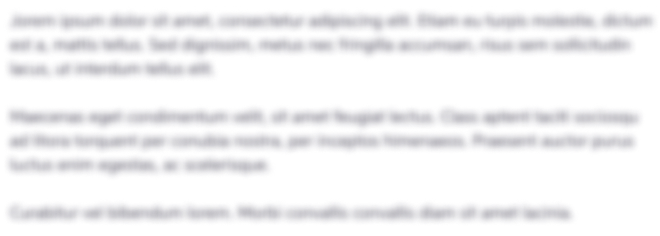
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started