Question
Write a program that will read in a list of positive integers (including zero) and display some statistics regarding the integers. The user is assumed
Write a program that will read in a list of positive integers (including zero) and display some statistics regarding the integers.
The user is assumed to enter the list in sorted order, starting with the smallest number and ending with the largest number.
Your program must store the all of the integers in an array. The maximum number of integers that you can expect is 100, however, there may not necessarily be that many. Your program should allow the user to continue entering numbers until they enter a negative number or until the array is filled - whichever occurs first. If the user enters 100 numbers, you should output a message stating that the maximum size for the list has been reached, then proceed with the calculations.
After you have placed all of the integers into an array, your program should perform the following tasks, in this order:
Display the count of how many numbers were read
Display the smallest number in the array
Display the largest number in the array
Display the median (the median is the integer that is stored in the middle position of the array)
Display the average of all the numbers
Allow the user to search the array for a specified value.
First, ask the user to enter an integer to search for.
Next, search the array to determine if the given integer is in the array.
If the integer is not found, display a message stating that the integer is not on the list.
If the integer is found, then display the position number of where you found the integer. If the integer happens to be in the array more than once, then you only need to tell the first position number where you found it.
After performing the search, ask the user if he/she wants to search for more integers. Continue searching for numbers until the user answers "N".
Technical notes and restrictions:
You should copy the methods created in the Assignment (parts A-D) and use them to create this project program. DO NOT CHANGE THE METHODS! They should be EXACTLY as they were written for the assignment. (METHODS A-D ARE FOUND AT THE END OF THIS POST).
Absolutely NO other global variables (class variables) are allowed (except for the keyboard object).
Create a MAXSIZE constant and set it to 100. You are only allowed to use the number 100 one time in your program - which is to set the MAXSIZE constant. You are not allowed to use the numbers 101, 99, 98, or any other number that is logically related to 100 anywhere in your program. If you need to make reference to the array size then, use the length variable, or the MAXSIZE constant to reference this rather than hard-coding numbers into your program.
The main program is responsible for all of the printing. There should be no screen printing in any of the four methods that came from your assignment parts A-D.
Remember that at this stage, COMMENTING IS A REQUIREMENT! Make sure you FULLY comment your program. You must include comments that explain what sections of code is doing. Notice the key word "sections"! This means that you need not comment every line in your program. Write comments that describe a few lines of code rather than "over-commenting" your program.
You MUST write comments about every method that you create. Your comments must describe the purpose of the method, the purpose of every parameter the method needs, and the purpose of the return value (if the method has one).
Build incrementally. Don't tackle the entire program at once. Write a little section of the program, then test it AND get it working BEFORE you go any further. Once you know that this section works, then you can add a little more to your program. Stop and test the new code. Once you get it working, then add a little bit more.
Make sure you FULLY test your program! Make sure to run your program multiple times, inputting combinations of values that will test all possible conditions for your IF statements and loops. Also be sure to test border-line cases.
The following is an example of what your MIGHT see on the screen when your program runs. The exact output depends on what values that the user types in while the program runs. The user's values are shown below in italics:
Enter a list of up to 100 positive integers. Please enter the values in ascending order. Enter a negative value to stop. 2 42 53 53 767 -1 The count of items entered is: 5 The smallest item in the list is: 2 The largest item in the list is: 767 The median of the list is: 53.0 The average of the list is: 183.4 Enter an integer to search for: 42 Item was found at position 1 Do you want to search for more numbers (Y or N)? Y Enter an integer to search for: 54 Item was not found. Do you want to search for more numbers (Y or N)? N
grading
Program must use arrays
Program must use your four UNCHANGED methods from the assignment
Program cannot use 'break' statements to exit loops
EVERY METHOD must have comments before the method which describe:
the purpose of the method
the purpose of each parameter the method needs (if any)
the purpose of the return value (if not void)
Use good variable names (Do not variable names which consist only of a single letter)
A: Missing, create your own method Write a method named getNums:
import java.util.*;
public class GetNumbers { public static Scanner kbd; public static final int MAXSIZE = 8; public static void main(String[] args) { kbd = new Scanner(System.in); int[]list = new int[MAXSIZE]; int numberOfElements = getNums(list); System.out.println(" The count of items entered is: "+ numberOfElements); kbd.close(); } /** * Gets a list of positive integers (including zero) from the user, and places them * into an array which has been previously initialized. The method stops when the * user enters a negative integer, or when the array is full. * @param list The array into which the numbers will be placed. * @return The count of numbers which were placed into the array. */ public static int getNums(int[]list) { int counter = 0; int value = 0; System.out.println("Enter a list of up to "+ MAXSIZE +" positive integers."); System.out.println("Enter a negative value to stop."); while(counter=0){ list[counter]=value; counter++; } if(counter==MAXSIZE){ System.out.println("The maximum size of the list has been reached."); } return counter; } }
B: Used to find the Median
public class CalculateMedian {
/** * Calculates the median of the integers in array 'list' * @param list The array containing the integers for which to calculate the median * @param size The number of integers in array 'list' * @return The median of the integers in the array */ public static double median(int [] list, int size) { double medianValue = 0.0; if (size%2!=0) medianValue=list[size/2]; else medianValue = (double)(list[(size-1)/2] + list[size/2])/2.0; return medianValue; } }
C: Method to find the average (missing create your own)
public class CalculateAverage {
/** * Calculates the average of the integers in array 'list' * @param list The array containing the integers for which to calculate the average * @param size The number of integers in array 'list' * @return The average of the integers in the array */ public static double average(int [] list, int size) { return 0; } }
D: method named search which searches for a specified integer in an array of integer
public class SearchArray { /** * Searches for an integer in an array * @param list The array containing the list of integers to search * @param size The number of integers in the array * @param key The integer to search for * @return The first position number of where key is found in the array, * or -1 if key is not found in the array. */ public static int search(int [] list, int size, int key) { int i; for(i=0;i if(list[i]==key) return i; } return -1; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
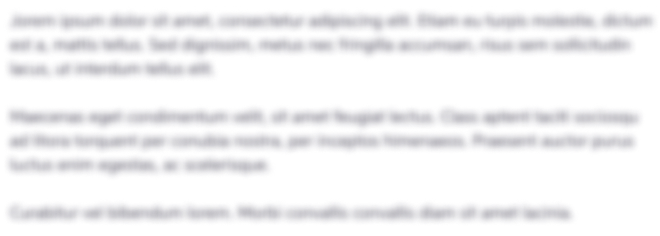
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started