Question
Write a Program to do the following: Step 1: Create a vending machine object o Contains a tower of cars (2D array) with the number
Write a Program to do the following: Step 1: Create a vending machine object o Contains a tower of cars (2D array) with the number of slots & columns read from file. Step 2: Load vending machine with all cars in the file o For each car in the file: o Read details for the car from the file. o Create a car object using the details. o Add car to vending machines tower in slot and column specified in file o Use the addCarToTower method in the VendingMachine class
Step 4: Display the loaded vending machine. o Display a nicely formatted version of the vending machine o Use the displayCars() method in the VendingMachine class Step 3: Print an inventory report for the vending machine. Write a method to print report: public static void printInventory (VendingMachine vendingMachine) This method must:
Be placed in your Assignment4 class Create one VehicleReport for each car and place all reports into an ArrayList Sort all VehicleReports in the ArrayList using the Collections.sort() method Print all VehicleReports in the ArrayList (which are now sorted by price) o Call the print() method on VehicleReport (see 2 nd output example)
4. Test file information a. Run your code on the provided file VendingMachineCars.txt This file is an example so DO NOT assume that your code should only work for a car tower with 6 slots and 5 columns and/or these cars in this specific order. The grader file will be different. 6 Number of slots in the tower (array row index) 5 Number of columns in tower (array column index) 1 1 29400.00 2015 Toyota Tacoma 1 2 31700.00 2012 BMW 6Series 1 5 41700.00 2016 Ford Mustang Details for each car 2 1 31000.00 2017 BMW X1 2 3 14300.00 2015 Nissan Altima 2 5 17900.00 2016 Dodge Challenger 3 2 26000.00 2017 Mini Convertible 3 3 39600.00 2017 Chevrolet Tahoe Note: 4 1 15100.00 2014 Chevy Volt - Column 0 and Slot 0 are NOT used 4 2 24400.00 2017 Toyota Rav4 - Column 1: slots 3 & 4 are empty 4 3 30400.00 2017 Subaru Outback - Column 2: slots 2 & 4 are empty 4 4 72900.00 2017 Tesla Model-S - Column 3: slots 1,4 & 5 are empty 4 5 43500.00 2016 Porsche Cayenne - Column 4: no empty slots
b. 1st line is the number of slots that each column contains c. 2nd line is the number of columns in the vending machines tower d. Remaining lines contain details for each car. The format is as follows: Column# Slot# Price Year Manufacturer Model 1 1 29400.00 2015 Toyota Tacoma
Interfaces and Classes Printable Interface Description o This interface represents an objects ability to print details about itself Public Methods o print() - returns string with details object wants to display in a nice format
VendingMachine Class Description o Represents the vending machine of cars. o The vending machine contains one car tower that is modeled by a 2-dimensional array. If you flatten the car tower it can be viewed as a 2D array of car objects. Im using the word slot instead of row since slot makes more sense when talking about placing a car in the tower, so slot = row in this assignment. Slot 0 and column 0 are not used
They are still part of 2D array, but we wont be placing cars into them. In the test file, the tower has 6 slots (0-5) and 5 columns (0-4). BUT the car tower is represented ONLY by slots 1-5 and columns 1-4 in the array as shown: Column 0 Column 1 Column 2 Column 3 Column 4 Slot 0 Slot 1 Toyota BMW -------- Chevy Slot 2 BMW -------- Mini Toyota Slot 3 -------- Nissan Chevy Subaru Slot 4 -------- -------- -------- Tesla Slot 5 Ford Dodge -------- Porsche
Private Data Fields o numberSlots number of slots in each column (i.e. row index ) o numberColumns number of columns in the vending machine (i.e. column index) o carTower array of Car objects use a 2D array NOT ArrayList see FAQ for help with 2D arrays
Public Methods o Constructor: public VendingMachine (int numberSlots, int numberColumns) Initializes numberSlots and numberColumns to incoming values Initializes size of car tower (2D array) to numberSlots and numberColumns carTower = new Car[numberSlots][numberColumns];
o Getters: For data fields numberSlots and numberColumns o Setters: None o addCarToTower (int slotNumber, int columnNumber, Car car) Places incoming car object in 2D array at a specified location (slot/column) Simulates loading a car into the vending machine in a specific location o getCar (int slot, int column) Returns the car stored in the tower (2D array) at the specific slot/column If there is no car in slot/column location (i.e. empty array location), returns null o displayCars() Displays a nicely formatted version of the vending machines tower (2D array) Print slot #, column #, and car manufacturer (see 1 st example output below)
Car Class Description o Represents one car Private Data Fields o price double value for the cars sticker price o year integer value for the cars year o manufacturer string value for the cars manufacturer o model string value for the cars model Public Methods
o Constructor public Car (double price, int year, String manufacturer, String model) initializes all private data fields with incoming values o Getter: One for each data field o Setters: None VehicleReport Class Description o Represents the report for one vehicle. o Contains slot number, column number, year, manufacturer, model, and price. o Class must implement Printable and Comparable
initializes all private data fields with incoming values o Getters and Setter: None o public String print() overrides method in Printable interface returns string with column#, slot#, year, manufacture, model, and price o public int compareTo(VehicleReport otherReport) overrides method in Comparable returns integer value (-1, 0, 1) based on result of comparing two vehicle reports Compares two vehicle reports based on the price
Mut Do and Tips Must Do Use a regular 2D array to model the vending machines car tower (see FAQ on 2D arrays) Use an ArrayList to store vehicle reports Use Javas pre-defined Comparable interface. Do not create your own Comparable interface! Tip: Reading Cars from File The number of cars is not part of the file as on previous assignments. On this assignment, use a while loop that reads until the end of the file is reached using the scanners hasNext method. Tip: Slots and Columns Slot zero and column zero is not used in the 2D array. They are part of the array but not used. Not all locations in the vending machines tower contain a car. o Empty locations in the array contain the value null
Tip: VehicleReport Sorting and Comparable In printInventory method, use the sort method on the Collections class. Heres how this works: o Because VehicleReport implements Comparable, the class must contain a compareTo method. The compareTo will compare two reports based on vehicle price.
o The connection to Collections.sort() method: Collections.sort uses an objects compareTo method to compare and sort objects! By sending the ArrayList of VehicleReports to Collections.sort, the ArrayList is sorted based on vehicle price. o See Listing 13.9 p. 515 in Liang for compareTo example
Tip: General One way to return nicely formatted strings in the print methods use string format method. o For example: return String.format(%d\t%d\t%-4d\t%-10s\t%-15s\t%-1.2f,
columnNumber, slotNumber, year, manufacturer, model, price);
o See chapter 4 in your book for details on format specifiers
Output Your output will look like the following when running against the test file VendingMachineCars.txt Loading cars into vending machine...
Column 1 Column 2 Column 3 Column 4 Slot 1 Toyota BMW ------ Chevy Slot 2 BMW ------ Mini Toyota Slot 3 ------ Nissan Chevy Subaru Slot 4 ------ ------ ------ Tesla Slot 5 Ford Dodge ------ Porsche
Dashes represent an empty slot in tower Produced using print method on VehicleReport ************************************************************************
VENDING MACHING INVENTORY (From Low to High Price)
************************************************************************ Column Slot Year Manufacturer Model Price ------------------------------------------------------------------------ 2 3 2015 Nissan Altima 14300.00 4 1 2014 Chevy 2014 Volt 15100.00 2 5 2016 Dodge Challenger 17900.00 4 2 2017 Toyota Rav4 24400.00 3 2 2017 Mini Convertible 26000.00 1 1 2015 Toyota Tacoma 29400.00 4 3 2017 Subaru Outback 30400.00 2 1 2017 BMW X1 31000.00 1 2 2012 BMW 6Series 31700.00 3 3 2017 Chevy Tahoe 39600.00 1 5 2016 Ford Mustang 41700.00 4 5 2016 Porsche Cayenne 43500.00 4 4 2017 Tesla Model-S 72900.00
Step by Step Solution
There are 3 Steps involved in it
Step: 1
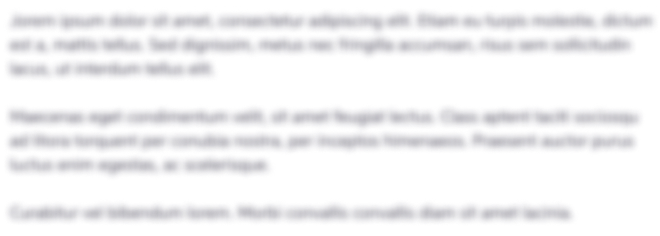
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started