Question
Write a program to test the class LinkedBag below For example create a bag by asking the user for few names then 1.Put those names
Write a program to test the class LinkedBag below
For example create a bag by asking the user for few names then
1.Put those names in the bag.
2. ask the user for a name and delete it from the bag.
3. ask for a name and check whether it is in the bag or not
4. print all names in the bag.
import java.util.*;
/**A class of bags whose entries are stored in a chain of linked nodes. */ public class LinkedBag
public LinkedBag(T[]items, int numberOfItems) { this(); for(int index = 0; index public boolean isFull() { return false; } /** Retrieves all entries that are in this bag. @return A newly allocated array of all the entries in the bag. */ public T[] toArray() { // The cast is safe because the new array contains null entries @SuppressWarnings("unchecked") T[] result = (T[])new Object[numberOfEntries]; // Unchecked cast int index = 0; Node currentNode = firstNode; while ((index < numberOfEntries) && (currentNode != null)) { result[index] = currentNode.data; index++; currentNode = currentNode.next; } // end while return result; } // end toArray public boolean isEmpty() { return numberOfEntries == 0; } public int getCurrentSize() { return numberOfEntries; } public int getFrequencyOf(T anEntry) { int frequency = 0; int loopCounter = 0; Node currentNode = firstNode; while ((loopCounter < numberOfEntries) && (currentNode != null)) { if (anEntry.equals(currentNode.data)) frequency++; loopCounter++; currentNode = currentNode.next; } // end while return frequency; } // end getFrequencyOf public boolean contains(T anEntry) { boolean found = false; Node currentNode = firstNode; while (!found && (currentNode != null)) { if (anEntry.equals(currentNode.data)) found = true; else currentNode = currentNode.next; } // end while return found; } // end contains private Node getReferenceTo(T anEntry) { boolean found = false; Node currentNode = firstNode; while (!found && (currentNode != null)) { if (anEntry.equals(currentNode.data)) found = true; else currentNode = currentNode.next; } return currentNode; } public void clear() { while (!isEmpty()) remove(); } // end clear public T remove() { T result = null; if (firstNode != null) { result = firstNode.data; firstNode = firstNode.next; // Remove first node from chain numberOfEntries--; } // end if return result; } // end remove public boolean remove(T anEntry) { boolean result = false; Node nodeN = getReferenceTo(anEntry); if (nodeN != null) { nodeN.data = firstNode.data; // Replace located entry with entry // in first node firstNode = firstNode.next; // Remove first node numberOfEntries--; result = true; } // end if return result; } // end remove private class Node { private T data; // Entry in bag private Node next; // Link to next node private Node(T dataPortion) { this(dataPortion, null); } // end constructor private Node(T dataPortion, Node nextNode) { data = dataPortion; next = nextNode; } // end constructor } }// end Node // end LinkedBag public interface BagInterface
Step by Step Solution
There are 3 Steps involved in it
Step: 1
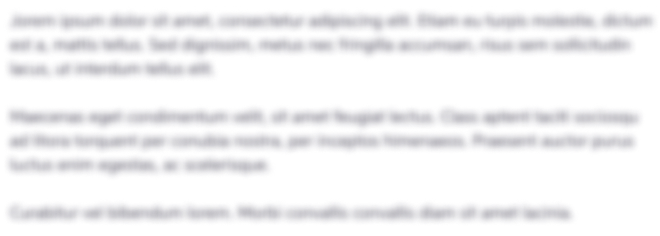
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started