Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a program using functions, arrays, and structures to calculate and display the total expenses for a business person on a trip. The following is
Write a program using functions, arrays, and structures to calculate and display the total expenses for a business person on a trip. The following is input from the user: total number of days spent on the trip; time of departure on the first day of the trip; time of arrival back home on the last day of the trip; amount of round-trip airfare(if any); amount of car rental fees per day (if any); miles driven if a private vehicle was used per day(if any); parking fees per day (if any); Taxi/Uber fees per day (if any); conference or seminar registration fees; hotel expenses per night; and the amount of each meal eaten, per day.
Additional Information:
Total days on trip cannot be less than 1 or greater than 10.
Vehicle expense for personal vehicle use if 54.5 cents/mile.
Taxi/Uber fees: $20 max per day will be covered.
Parking fees: $6.00 max per day will be covered.
Hotel expenses: $110.00 max per night will be covered.
Meal expenses:
-On the first day of the trip
Breakfast is allowed if the departure time is before 7:00 am.
Lunch is allowed if the departure time is before 12:00 pm.
Dinner is allowed if the departure time is before 6:00 pm.
-On the last day of the trip:
Breakfast is allowed if the arrival time is after 7:00 am.
Lunch is allowed if the arrival time is before 1:00 pm.
Dinner is allowed if the arrival time is after 7:00 pm.
Your program should only ask for ALLOWABLE meals.
Max covered prices are (per day that the business person is on a trip and is allowed these meals )
-Breakfast : $12.00
-Lunch: $15.00
-Dinner: $19.00
Your program should calculate and display the total expenses for each category; the total amount of expenses incurred; the total amount covered by the company, the total amount the employee will not be reimbursed for (if any) for anything spent over the max covered amounts.
This is what Ive got so far. I need help with the logic and programming of this assignment please and thank you.
//Travel Expenses
#include
using namespace std;
struct Travel
{
int days;
int timeDepart;
int timeArrival;
float airfare;
float carRental;
float milesDriven;
float parkingFees;
float taxiFees;
float registrationFees;
float hotelExpenses;
float meals[10][3];
float totalSpent;
float totalAllowed;
string mealType[3]={"Breakfast", "Lunch", "Dinner"};
};
void getData(struct Travel &);
double getCalc(struct Travel &);
int main()
{
struct Travel employee;
getData(employee);
return 0;
}
void getData(struct Travel& employee)
{
cout<<"Enter the amount of days spent on the trip: ";
cin>>employee.days;
while(employee.days > 1 && employee.days > 10 )
{
cout<<"Enter a valid number of days(less than 10 days) spent on the trip: ";
cin>>employee.days;
}
cout<<"Enter the time of departure in Military Time (24-hour HHMM format): ";
cin>>employee.timeDepart;
while ( employee.timeDepart <0 || employee.timedepart> 2400)
{
cout << "Enter a number between 0000 and 2400: ";
cin >> employee.timeDepart;
}
cout<<"Enter the time of arrival in Military Time (24-hour HHMM format): ";
cin>>employee.timeArrival;
while ( employee.timeArrival <0 || employee.timearrival> 2400)
{
cout << "Enter a number between 0000 and 2400: ";
cin >> employee.timeArrival;
}
cout<<"Enter the amount of round-trip airfare: ";
cin>>employee.airfare;
while (employee.airfare < 0)
{
cout << "Enter an amount of round-trip airfare greater than 0: " ;
cin >> employee.airfare;
}
cout<<"Enter the amount spent on car rental: ";
cin>>employee.carRental;
while (employee.carRental < 0)
{
cout << "Enter an amount spent on car rental greater than 0: " ;
cin >> employee.carRental;
}
cout<<"Enter the miles driven if a private vehicle was used: ";
cin>>employee.milesDriven;
while (employee.milesDriven < 0)
{
cout << "Enter the number of miles driven greater than 0: " ;
cin >> employee.milesDriven;
}
cout<<"Enter the parking fees if any: ";
cin>>employee.parkingFees;
while (employee.parkingFees < 0)
{
cout << "Enter an amount of parking fees greater than 0 if any or 0 if none: " ;
cin >> employee.parkingFees;
}
cout<<"Enter the taxi fees: ";
cin>>employee.taxiFees;
while (employee.taxiFees < 0)
{
cout << "Enter an amount spent on taxis greater than 0 if any or 0 if none: " ;
cin >> employee.taxiFees;
}
cout<<"Enter the registration fees: ";
cin>>employee.registrationFees;
while (employee.registrationFees < 0)
{
cout << "Enter an amount spent on registration fees greater than 0 or 0 if none: " ;
cin >> employee.registrationFees;
}
cout<<"Enter the hotel expenses: ";
cin>>employee.hotelExpenses;
while (employee.hotelExpenses < 0)
{
cout << "Enter an amount spent on hotel expenses greater than 0 or 0 if none: " ;
cin >> employee.hotelExpenses;
}
//int numDays=employee.days;
for(int col=0; col
{
for(int row=0; row<3;++row)
{
cout<<"Enter cost for "<
cin>>employee.meals[col][row];
while (employee.meals[col][row] < 0)
{
cout << "Enter an amount spent on meals greater than 0 or 0 if none: " ;
cin >> employee.meals[col][row];
}
if(employee.days==1 && employee.timeDepart > 0 && employee.timeDepart <7000)
{
}
}
}
}
double getCalc(struct Travel& employee)
{
float milesCost;
float allowedTaxiFees, allowedParkingFees; allowedHotelExpenses;
milesCost=employee.milesDriven*.545;
for(int x=0;x
{
allowedTaxiFees+=
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
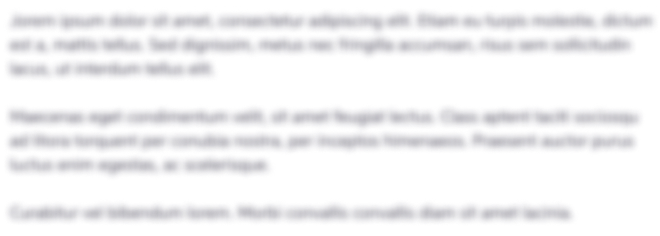
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started