Question
Write a Python function, compound_properties(), that is passed a file name of a CSV file (assume the file is in the same folder as your
Write a Python function, compound_properties(), that is passed a file name of a CSV file (assume the file is in the same folder as your Python file) and a compounds formula unit structure**. The file will contain metal elements and their properties. In the function, you are tasked to read the compound formulation (by the molform() function described below), find out properties of those constituent in the compound in the CSV file, and return a tuple of:
-
The element in the compound with the lowest boiling point (full name, like Hydrogen for H)
-
The average boiling point of all elements, round to the nearest integer
-
The molecular mass of that compound (the atomic mass of each element, multiplied by the number of atoms in the molecule), round to the nearest integer:
For example,
>>>compound_properties(input.csv, Fe1S1O4) #FeSO4 (Oxygen, 1314, 152)
Write a second function, molform(), that will be called by the first function, to take the compounds formula and return it as a dictionary with the elements as the keys and the number of atoms as the values. Note that, you can assume element names wont exceed two letters in length, but the number of atoms might be huge.
You may assume the compound structure will specify the number of atoms, even if the IUPAC formula would omit the subscript 1. For instance, ethanol, C2H6O, would be passed to the program as the string C2H6O1.
Your function must read files with the format of atoms.csv. Each new column of data is separated by a comma (CSV). You can test your function using a subset of the file atoms.csv (create the subset by removing many lines from it).
Do not change the file name or function names. Do not use input() or print(). Your file should not contain any additional lines of code outside the function definitions. Some test cases are included in the docstrings.
**Note: If you do not know how to read chemical compounds, watch this short video: https://www.youtube.com/watch?v=mvGOOyqwdhc
Help with files/CSVs
Reading files
Reading files is made very easy in Python. There are only 3 steps to it: open file, read it and close the file. You can use the following code to read a file.
f = open(path_to_file, mode='r') lines = f.readlines() f.close()
Now, lines is a list of all the lines in the file you just read, with each element corresponding to one line in the file.
Reading CSV files
CSV files are just regular text files. Basically, every line in the CSV is a row in the table. Columns of the table are separated by comma, thus the name Comma Separated Values, aka, CSV.
You can read the csv as a normal file and split by comma to get the values on your own, or you can use pythons csv module to save some work, although it wont make much difference regarding the CSV you are reading in this lab.
For the usage of pythons csv module, check here: https://docs.python.org/3.7/library/csv.html
Sample Input and Output:
>>>isinstance(molform("C2H6O1"), dict)
True >>>isinstance(molform("C2H6"), dict)
True
>>>isinstance(molform("C2"), dict) True >>>molform("C2H6O1") {'C': 2, 'H': 6, 'O': 1} >>>molform("C1H4")
{'C': 1, 'H': 4} >>>isinstance(compound_properties("atoms.csv", "K1Fe4"), tuple)
True >>>isinstance(compound_properties("atoms.csv", "K1Fe4")[0], str)
True >>>isinstance(compound_properties("atoms.csv", "K1Fe4")[1], int)
True >>>compound_properties("atoms.csv", "K1Cl1O3") ('Oxygen', 454, 123) >>>compound_properties ("atoms.csv", "C6H12O6")
('Hydrogen', 1470, 180)
The atoms.csv Document:
The initial code given (lab6.py document):
cudef compound_properties(csv_name, compound_formula):
"""(str, str) -> tuple This function takes two parameters: the csv filename and the formula of the compound.
It should call the molform() function to get the composition of the molecule and get the required property from the csv file. The csv file will have all the properties required.
This function is required to return a tuple of three properties: 1. The NAME of the atom with the lowest boiling point. For example, if it's oxygen, return 'Oxygen', not 'O' 2. The average (mean) of the atoms' boiling point in the molecule, round to the nearest integer For example, for FeSO4, it's the average boiling point of Fe, S and O, do not multiply the boiling point by the number of the atoms 3. The molecular mass of the compound, round to the nearest integer For example, C1O2 (carbon dioxide) has weight of 12*1+16*2 = 44 """
def molform(compound_formula): """(str) -> dictionary When passed a string of the compound formula, returns a dictionary with the elements as keys and the number of atoms of that element as values. When the string is empty, return an empty dictionary
>>> molform("C2H6O1") {'C': 2, 'H': 6, 'O': 1} >>> molform("Cr1H4") {'Cr': 1, 'H': 4} >>> molform("C132983H211861N36149O40883S693") {'C': 132983, 'H': 211861, 'N': 36149, 'O': 40883, 'S': 693} """
atomicNumber, symbol, name, atomicMass, boilingPoint 1, H , Hydrogen,1.00794,20 2, He , Helium,4.002602,4 3, Li, Lithium,6.941,1615 4, Be, Beryllium,9.012182,2743 5, B , Boron,10.811,4273 6, C , Carbon, 12.0107,4300 7, N , Nitrogen, 14.0067,77 8, O, Oxygen, 15.9994,90 9, F, Fluorine, 18.9984032,85 10, Ne , Neon,20.1797,27 11, Na, Sodium,22.98976928,1156 12, Mg , Magnesium,24.305,1363 13, Al, Aluminum,26.9815386,2792 14, Si , Silicon,28.0855,3173 15, P , Phosphorus,30.973762,554 16, S, Sulfur,32.065,718 17, CI, Chlorine,35.453,239 18, Ar , Argon,39.948,87 19, K, Potassium,39.0983,1032 20, Ca, Calcium,40.078,1757 21, Sc, Scandium,44.955912,3103 22, Ti , Titanium,47.867,3560 23, V , Vanadium,50.9415,3680 24, Cr, Chromium,51.9961,2944 25, Mn , Manganese,54.938045,2334 26, Fe , Iron,55.845,3134 27, Co, Cobalt,58.933195,3200 28, Ni , Nickel,58.6934,3186 29, Cu , Co 30, Zn , Zinc,65.38,1180 31, Ga, Gallium,69.723,2477 32, Ge, Germanium,72.64,3093 33, As , Arsenic,74.9216,887 34, Se , Selenium,78.96,958 35, Br, Bromine,79.904,332 36, Kr , Krypton,83.798,120 37, Rb , Rubidium,85.4678,961 38, Sr, Strontium,87.62,1655 39, Y , Yttrium,88.90585,3618 40, Zr, Zirconium,91.224,4682 41, Nb , Niobium,92.90638,5017 42, Mo , Molybdenum,95.96,4912 43, Tc , Technetium,98,4538 44, Ru, Ruthenium,101.07,4423 pper,63.546,3200 45, Rh, Rhodium,102.9055,3968 46, Pd , Palladium,106.42,3236 47, Ag, Silver,107.8682,2435 48, Cd, Cadmium,112.411,1040 49, In , Indium,114.818,2345 50, Sn , Tin,118.71,2875 51, Sb , Antimony, 121.76,1860 52, Te , Tellurium,127.6,1261 53, 1, lodine, 126.90447,457 54, Xe , Xenon,131.293,165 55, Cs , Cesium,132.9054519,944 56, Ba, Barium, 137.327,2143 57, La , Lanthanum,138.90547,3737 58, Ce , Cerium,140.116,3633 59, Pr , Praseodymium,140.90765,3563 60, Nd, Neodymium, 144.242,3373 61, Pm , Promethium,145,3273 62, Sm, Samarium,150.36,2076 63, Eu , Europium,151.964,1800 64, Gd, Gadolinium,157.25,3523 65, Tb , Terbum, 158.92535,3503 66, Dy , Dysprosium,162.5,2840 67, Ho , Holmium,164.93032,2973 68, Er, Erbium,167.259,3141 69, Tm, Thulium,168.93421,2223 70, Yb, Ytterbium,173.054,1469 71, Lu, Lutetium,174.9668,3675 72, Hf, Hafnium,178.49,4876 73, Ta , Tantalum ,180.94788,5731 74, W , Tungsten, 183.84,5828 75, Re , Rhenium,186.207,5869 76, Os, Osmium,190.23,5285 77, Ir, Iridium, 192.217,4701 78, Pt, Platinum,195.084,4098 79, Au, Gold,196.966569,3129 80, Hg, Mercury,200.59,630 81, TI, Thallium,204.3833,1746 82, Pb , Lead,207.2,2022 83, Bi, Bismuth,208.9804,1837 84, Po , Polonium,209,1235 85, At, Astatine,210,610 86, Rn, Radon,222,211 87, Fr, Francium,223,950 88, Ra, Radium,226,2010 89, Ac, Actinium, 227,3473 90, Th , Thorium,232.03806,5093 91, Pa , Protactinium,231.03588,4273 92, U, Uranium,238.02891,4200 93, Np, Neptunium,237,4273 94, Pu, Plutonium,244,3503 95, Am, Americium,243,2284 96, Cm, Curium,247,3383 atomicNumber, symbol, name, atomicMass, boilingPoint 1, H , Hydrogen,1.00794,20 2, He , Helium,4.002602,4 3, Li, Lithium,6.941,1615 4, Be, Beryllium,9.012182,2743 5, B , Boron,10.811,4273 6, C , Carbon, 12.0107,4300 7, N , Nitrogen, 14.0067,77 8, O, Oxygen, 15.9994,90 9, F, Fluorine, 18.9984032,85 10, Ne , Neon,20.1797,27 11, Na, Sodium,22.98976928,1156 12, Mg , Magnesium,24.305,1363 13, Al, Aluminum,26.9815386,2792 14, Si , Silicon,28.0855,3173 15, P , Phosphorus,30.973762,554 16, S, Sulfur,32.065,718 17, CI, Chlorine,35.453,239 18, Ar , Argon,39.948,87 19, K, Potassium,39.0983,1032 20, Ca, Calcium,40.078,1757 21, Sc, Scandium,44.955912,3103 22, Ti , Titanium,47.867,3560 23, V , Vanadium,50.9415,3680 24, Cr, Chromium,51.9961,2944 25, Mn , Manganese,54.938045,2334 26, Fe , Iron,55.845,3134 27, Co, Cobalt,58.933195,3200 28, Ni , Nickel,58.6934,3186 29, Cu , Co 30, Zn , Zinc,65.38,1180 31, Ga, Gallium,69.723,2477 32, Ge, Germanium,72.64,3093 33, As , Arsenic,74.9216,887 34, Se , Selenium,78.96,958 35, Br, Bromine,79.904,332 36, Kr , Krypton,83.798,120 37, Rb , Rubidium,85.4678,961 38, Sr, Strontium,87.62,1655 39, Y , Yttrium,88.90585,3618 40, Zr, Zirconium,91.224,4682 41, Nb , Niobium,92.90638,5017 42, Mo , Molybdenum,95.96,4912 43, Tc , Technetium,98,4538 44, Ru, Ruthenium,101.07,4423 pper,63.546,3200 45, Rh, Rhodium,102.9055,3968 46, Pd , Palladium,106.42,3236 47, Ag, Silver,107.8682,2435 48, Cd, Cadmium,112.411,1040 49, In , Indium,114.818,2345 50, Sn , Tin,118.71,2875 51, Sb , Antimony, 121.76,1860 52, Te , Tellurium,127.6,1261 53, 1, lodine, 126.90447,457 54, Xe , Xenon,131.293,165 55, Cs , Cesium,132.9054519,944 56, Ba, Barium, 137.327,2143 57, La , Lanthanum,138.90547,3737 58, Ce , Cerium,140.116,3633 59, Pr , Praseodymium,140.90765,3563 60, Nd, Neodymium, 144.242,3373 61, Pm , Promethium,145,3273 62, Sm, Samarium,150.36,2076 63, Eu , Europium,151.964,1800 64, Gd, Gadolinium,157.25,3523 65, Tb , Terbum, 158.92535,3503 66, Dy , Dysprosium,162.5,2840 67, Ho , Holmium,164.93032,2973 68, Er, Erbium,167.259,3141 69, Tm, Thulium,168.93421,2223 70, Yb, Ytterbium,173.054,1469 71, Lu, Lutetium,174.9668,3675 72, Hf, Hafnium,178.49,4876 73, Ta , Tantalum ,180.94788,5731 74, W , Tungsten, 183.84,5828 75, Re , Rhenium,186.207,5869 76, Os, Osmium,190.23,5285 77, Ir, Iridium, 192.217,4701 78, Pt, Platinum,195.084,4098 79, Au, Gold,196.966569,3129 80, Hg, Mercury,200.59,630 81, TI, Thallium,204.3833,1746 82, Pb , Lead,207.2,2022 83, Bi, Bismuth,208.9804,1837 84, Po , Polonium,209,1235 85, At, Astatine,210,610 86, Rn, Radon,222,211 87, Fr, Francium,223,950 88, Ra, Radium,226,2010 89, Ac, Actinium, 227,3473 90, Th , Thorium,232.03806,5093 91, Pa , Protactinium,231.03588,4273 92, U, Uranium,238.02891,4200 93, Np, Neptunium,237,4273 94, Pu, Plutonium,244,3503 95, Am, Americium,243,2284 96, Cm, Curium,247,3383Step by Step Solution
There are 3 Steps involved in it
Step: 1
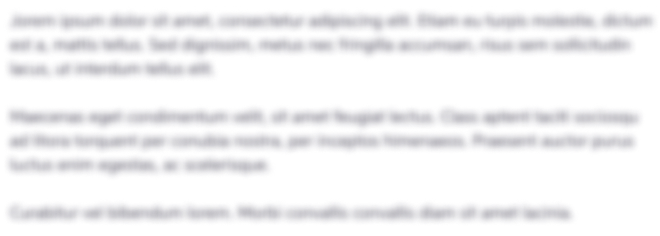
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started