Question
Write a python program that will read polling data taken from Real Clear Politics to see how GOP candidates were doing from January through mid-March
Write a python program that will read polling data taken from Real Clear Politics to see how GOP candidates were doing from January through mid-March in Florida, Ohio, and North Carolina.
You will use a subset of data that we provide for this assignment. The links you need are (open these as txt file in python to make it easiest to read/work with):
http://tinyurl.com/florida-gop1
http://tinyurl.com/ohio-gop2
http://tinyurl.com/nc-gopp
The fields in each file are, in order:
polling company,date range,how many polled,margin of error,cruz,kasich,rubio,trump
You must write the functions below, and only the functions below, to complete this.
Functions
1. read_data_file(filename): Given a string representing a filename of the file that you want to open, open the file and read the data into a list of lists, in which each poll is a list consisting of the data items mentioned above. You will need to cast the numeric values to int before returning. Return this lists of lists.
For example, running read_data_file('ohio-gop.csv') should return:
[['Quinnipiac', '3/8 - 3/13', 721, 4, 16, 38, 3, 38], ['Monmouth', '3/11 - 3/13', 503, 4, 15, 40, 5, 35], ['CBS News/YouGov', '3/9 - 3/11', 828, 4, 27, 33, 5, 33], ['NBC/WSJ/Marist', '3/4 - 3/10', 564, 4, 19, 39, 6, 33], ['FOX News', '3/5 - 3/8', 806, 4, 19, 34, 7, 29], ['PPP (D)', '3/4 - 3/6', 638, 4, 15, 35, 5, 38], ['Quinnipiac', '3/2 - 3/7', 685, 4, 16, 32, 9, 38], ['CNN/ORC', '3/2 - 3/6', 359, 5, 15, 35, 7, 41], ['Quinnipiac', '2/16 - 2/20', 759, 4, 21, 26, 13, 31]]
2. poll_winner(poll_to_examine): Given a list in the order shown in the example, return two values - the last name of who is winning, along with the margin of victory (i.e. how much did first place beat second place). For example, it may be Trump and +14 for one poll or Rubio and +3 for another poll. When printed, it will appear as (Trump, +14), but the parentheses and other punctuation are just done by the print. Your return statement should have two values in it, such as return name, value.
For example, running poll_winner(['Company X', 'Some Dates', 100,5,5,50,25,20]) should return:
('Kasich', '+25') - this is two values, not to be confused with a tuple or set
If there is a tie, return just the word TIE with no value.
3. latest_poll_winner_by_state(state): Given a state, return the same information as poll_winner for the latest poll for that given state. Note that this is always the first poll in the file. Also, note that you can use poll_winner inside this function, which makes this function ostensibly very short.
For example, running latest_poll_winner_by_state('florida-gop.csv') should return:
('Trump', '+24') - this is two values, not to be confused with a tuple or set
If there is a tie, return just the word TIE with no value.
4. average_of_polls(state, number_of_polls=5): Given a state and optionally a number of polls, calculate the average of all relevant values and return a new list representing the average poll.
For example, running average_of_polls('florida-gop.csv') should return:
['Average Poll', 5, 651, 4, 19, 9, 22, 44] - the 5 in the date field represents the number of polls used in the average
Step by Step Solution
There are 3 Steps involved in it
Step: 1
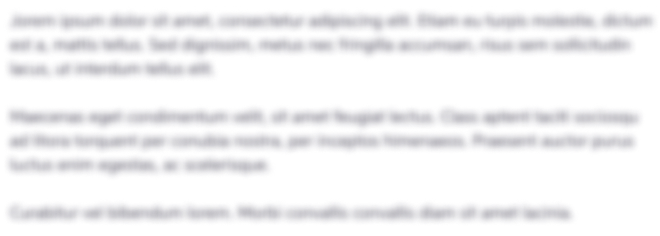
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started