Question
Write a static method named matrixAverages that accepts a two-dimensional array of integers as a parameter. The method averages each row and column of the
Write a static method named matrixAverages that accepts a two-dimensional array of integers as a parameter. The method averages each row and column of the matrix and prints out each of the averages.
A template .java file is provided (MatAvgTemplate.java) that will provide the class definition, method definition and several matrices that will be used for test cases.
The main() procedure should simply pass the matrix to matrixAverages which will do the computations AND print the results.
The prototype for matrixAverages is:
public static void matrixAverages(int[][] theMatrix);
Background
Recall that in row major order, the elements are arranged such that the right index advances the quickest. So the order of elements is for an array declared:
int[][] anArray = new int[2][3];
is
anArray[0][0], anArray[0][1], anArray[0][2]
anArray[1][0], anArray[1][1], anArray[1][2]
Averaging rows would involve adding the three elements in the top row and dividing by 3 followed by adding the elements in the 2nd row above and dividing by 3.
So
(anArray[0][0] + anArray[0][1] + anArray[0][2]) / (double) 3
and
(anArray[1][0] + anArray[1][1] + anArray[1][2]) / (double) 3
Averaging columns would involve adding pairs of elements that are above and below each other in the list above and dividing by 2.
So the averages would be:
(anArray[0][0] + anArray[1][0]) / (double) 2
(anArray[0][1] + anArray[1][1]) / (double) 2
(anArray[0][2] + anArray[1][2]) / (double) 2
The program must be generalized to handle any size 2 dimensional array.
Example Run
For the test array:
int[][] tmatrix1 = {{2, 10, 14, 200},
{8, 19, 30, 21},
{100, 1001, -4, 15},
{10, 20, -15, 25}};
The output should be:
Row 0 average is 56.5
Row 1 average is 19.5
Row 2 average is 278.0
Row 3 average is 10.0
Column 0 average is 30.0
Column 1 average is 262.5
Column 2 average is 6.25
Column 3 average is 65.25
public class MatAvgTemplate { public static void main(String[] args) { // These are matrices that provide 'test cases' for your code. int[][] tmatrix1 = {{2, 10, 14, 200}, {8, 19, 30, 21}, {100, 1001, -4, 15}, {10, 20, -15, 25}}; int[][] tmatrix2 = {{2, 10, 14, 200, -1, 7}, {8, 19, 30, 21, 10, -14}, {100, 0, 999, -4, 15, 16}, {10, 20, 2, -3, -15, 25}}; int[][] tmatrix3 = {{2, 10, 13}, {8, 19, 31}, {100, 998, 16}, {2, 171, 200}, {100, 899, 12}, {10, 20, 12}}; // Make calls to matrixAverages for each of the test // arrays provided above. } public static void matrixAverages(int[][] theMatrix) { // Compute and print the averages of each row of 'theMatrix'. // Compute and print the averages of each column in // 'theMatrix'.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
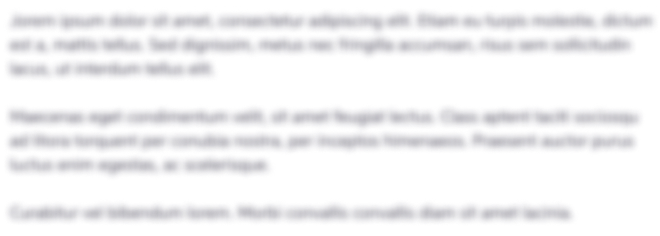
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started