Question
Write an algorithm of the C++ project below that describes both the conversion class and the driver program of the project below. By algorithm, this
Write an algorithm of the C++ project below that describes both the conversion class and the driver program of the project below. By algorithm, this should be a step-by-step set of instructions to solve a specific task. Each instruction will be written in English, and numbered in order. Each step should be detailed, concise, and unambiguous. The algorithm should be detailed enough to be translated to code, although it should not be actual code. You are describing steps to be carried out in any formal language, not just C++.
main driver file:
#include
#include
#include
#include "convert.h"
using namespace std;
int main()
{
string str;
cout <<
"Please enter a string representing a positive integer or decimal: ";
cin >> str;
convertToNumeric ctn(str);
long int integerValue = ctn.getIntValue();
double doubleValue = ctn.getDecValue();
double integral;
/* If the string represents an integer value, adding decimal portion to
* the decimal value.
*/
if (std::modf(doubleValue, &integral) == 0)
{
cout << "Integer Conversion: " << integerValue << endl;
cout << "Decimal Conversion: " << std::fixed << std::setprecision(1)
<< integral < } /* * Else, display the values normally. */ else { cout << "Integer Conversion: " << integerValue << endl; cout << "Decimal Conversion: " << doubleValue << endl; } /* * Demonstrating that the converted values are useable with * long int-specific and double-specific operations. */ cout << endl; cout << "Demonstration of long int: " << integerValue << " + 100 = " << (integerValue + 100) << endl; cout << "Demonstration of decimal: " << doubleValue << " + 100.12 = " << (doubleValue + 100.12) << endl; return 0; } convert.cpp file: #include #include "convert.h" using namespace std; convertToNumeric::convertToNumeric() { str = "123"; } /* * This accessor function is the overloaded constructor that takes a string * and calls the convert method to convert into respective integer and * decimal value. There is not return value. The input is s, which is the * string to be converted. */ convertToNumeric::convertToNumeric(string s) { str = s; if(convert(str)) { cout << "Conversion Successful!" << endl; } else { cout << "Conversion failed." < } } /* * This mutator function serves the purpose of converting a string into its * respective integer and decimal values. The input is s, which is the * string to be converted. The function returns a boolean value indicating * whether conversion is successful or not. */ bool convertToNumeric::convert(string s) { bool success = false; bool dotFound = false; /* * Here, we are looping through each character in the string and storing * the integer portion in cstk1 and the decimal portion in cstk2. * Ex: If s = 123.45, cstk1 = 321 and cstk2 = 54 * (since the stack follows LIFO) */ int i; for(i = 0; i < s.size(); i++) { if(s[i] == '.') dotFound = true; else if(dotFound) { cstk2.push(s[i]); } else { cstk1.push(s[i]); } } /* * Here, we are popping out each value from stack cstk1 and generating * the integer value. * Ex: s = 123.45 and cstk1 = 321, num = 0 * Iteration 1: num = 0 + (3 * 1) = 3 * Iteration 2: num = 3 + (2 * 10) = 23 * Iteration 3: num = 23 + (1 * 100) = 123 * Now intValue = 123 */ long int num = 0; int multiplyValue = 1; char val = '0'; while(cstk1.pop(val)) { int valInInt = val - '0'; num += (valInInt * multiplyValue); multiplyValue *= 10; } intValue = num; /* * Here, we are popping out each value from stack cstk2 and generating * the decimal value. * Ex: s = 123.45 and cstk2 = 54, num = 0, doubleValue = 123 * (equated to integer value) * Iteration 1: num = 0 + (5 * 1) = 5 * Iteration 2: num = 5 + (4 * 10) = 45 * Iteration 3: num = 45 / 100 = 0.45 * Now doubleValue = 123 + 0.45 = 123.45 */ doubleValue = double(num); num = 0; multiplyValue = 1; while(cstk2.pop(val)) { int valInInt = val - '0'; num += (valInInt * multiplyValue); multiplyValue *= 10; } doubleValue += (double(num) / multiplyValue); success = true; return success; } /* * The accessor function below is returning the integer value of the string * that has been converted. */ long int convertToNumeric::getIntValue() { return intValue; } /* * The accessor function below is returning the double value of the string * that has been converted. */ double convertToNumeric::getDecValue() { return doubleValue; } convert.h header file: #include #include "char_stack.h" #ifndef CONVERT_H #define CONVERT_H using namespace std; class convertToNumeric { public: convertToNumeric(); convertToNumeric(string str); bool convert(string str); long int getIntValue(); double getDecValue(); private: string str; long int intValue; double doubleValue; char_stack cstk1, cstk2; }; #endif char_stack.cpp file: #include "char_stack.h" //--------------------------------------------------------------------------- /* The stack is initially empty and the top variable is designed to reference * the topmost valid item on the stack. The stack is implemented as a fixed * size character array. When the stack is empty, top must be set to -1. */ char_stack::char_stack() { top = -1; } //--------------------------------------------------------------------------- /* In this mutator function, we can only pop an item off if the stack is * not empty. The input is item. The function returns a boolean indicating * success or faillure of popping. */ bool char_stack::pop(char& item) { bool success = false; if (!isEmpty()) { item = stk[top--]; success = true; } return success; } //--------------------------------------------------------------------------- /* In this mutator function, we can only push an item when the stack is not * full. The input is item. The function returns a boolean indicating * success or failure of pushing item on stack. */ bool char_stack::push(char item) { bool success = false; if (!isFull()) { stk[++top] = item; success = true; } return success; } //--------------------------------------------------------------------------- /* In this accessor function, the top variable represents the index of the * element that is currently holding the topmost item. The stack is * implemented as a fixed size array. Therefore, when the stack is empty, * top must be -1. The function returns a boolean indicating whether the * stack is empty. */ bool char_stack::isEmpty() const { return (top == -1); } //--------------------------------------------------------------------------- /* This is an accessor function. Since the stack is a fixed array of size * STACKSIZE, the topmost element is stored at index STACKSIZE minus 1. When * top equals STACKSIZE minus 1, the stack is full. The function returns * a boolean indicating whether the stack is full. */ bool char_stack::isFull() const { return (top == (STACKSIZE - 1)); } char_stack.h header file: #ifndef CHAR_STACK_H #define CHAR_STACK_H const char STACKSIZE = 100; class char_stack { public: char_stack(); bool pop(char& item); bool push(char item); private: bool isEmpty() const; bool isFull() const; int top; char stk[STACKSIZE]; }; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
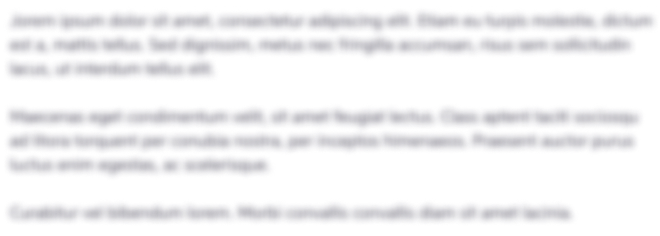
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started