Question
Write in Java Movie Theater Ticket App Write a program that allows a user to purchase movie tickets for a showing of one of three
Write in Java
Movie Theater Ticket App
- Write a program that allows a user to purchase movie tickets for a showing of one of three movies.
- Your program will work with two files - a Movie.java and Theater.java file - which will interact with each other.
- You should begin by creating three Movie objects which will store information about three movies of your choice
- Once the information has been stored, the program should welcome the user and ask the user to type in the name of one of the three movies
- Then, the program should allow the user to select seats based on a seating chart, stored as a 2D array.
- The user should be allowed to purchase as many tickets as desired (until the theater is sold out!)
- At the end of the program, display the total number of seats purchased by the user as well at the total cost.
- Below is the required starter code for this program:
Movies.java File:
- The Movies.java file contains 6 variables, storing information about the name, price, total number of tickets sold, and showtime of the movie, as well as a 2D array storing the seating chart for the movie.
- Note that all seats should be open at the start of the program (open seats represented as "X")
- Additionally, this class has 3 methods as described below
/** * @author * @author * Lab 6, Movie.java */ public class Movie { public String name; public double price; public String showTime; public final int SIZE = 9; public int ticketsSold; public String[][] seats = new String[SIZE][SIZE]; /** * Assigns the value of "X" to each * seat in the seats array */ public void initializeSeats() { } /** * Prints out information about the movie * in the format * * Ticket Price: $ * Show time: * Note that there are no <> around the output * The <> mean fill in here */ public void printMovieStats() { } /** * Prints out the seats array to the console * with row and column numbers * and with the placement of the SCREEN in * row 10 * Along with the message "Here are the * available seats for :" * Note that there are no <> around the output */ public void printSeatingChart() { } }
- Note: you are not allowed to implement any additional methods aside from those whose signatures are provided or to alter the method signatures in any way from what is provided, or you will not receive credit for this assignment.
Theater.java File:
- The Theater.java file contains a main method, and code to interact with the user and the Movie class.
- In main, you should create 3 Movie objects of your choice. Note that you may also update the Star Wars example code below to be a movie of your choice.
- Theater.java has an optional method described below. Note that you are not required to implement this method in your program.
- You may optionally add any additional methods to this class that you would find useful.
- When the program starts, the user should be presented with the three movie choices.
- The user should be allowed to type both capital and lower case letters for the movie name.
- The user can select one of the movies and purchase as many tickets as desired for that movie (see example output below).
- Initially, the theater should be empty (all seats marked with "X" to indicate they are open).
- As the user purchases tickets, the open seats should be replaced with a "T" (for taken).
- The program should do some error checking to prevent the user from purchasing a seat that is already taken (see example output below).
- At the end of the program, print the total number of tickets purchased and the total price (see sample output).
/** * @author * @author * Lab 6, Theater.java */ import java.util.Scanner; public class Theater { public static void main(String[] args) { int row, col; String choice; Scanner input = new Scanner(System.in); Movie starWars = new Movie(); starWars.name = "Star Wars: The Rise of Skywalker"; starWars.price = 10.50; starWars.ticketsSold = 0; starWars.showTime = "9:00pm"; starWars.initializeSeats();
//Add two more movie objects here - movies of your choice!
System.out.println("Welcome to the CineBucks Movie Theater!"); System.out.println(" Below are our current movies and showtimes: "); input.close(); } /** * This method is optional, but can be used * to avoid repetitive code for each movie * menu option * @param m the Movie object, for the movie * selected by the user * @param input the Scanner object */ public static void menuItem(Movie m, Scanner input) { } }
- When your program is working *identically* to the sample output shown below, including the demonstrated error checking, please submit both Movie.java and Theater.java to Canvas.
Sample Output:
Welcome to the CineBucks Movie Theater! Below are our current movies and showtimes: Star Wars: The Rise of Skywalker Ticket price: $10.50 Show time: 9:00pm Little Women Ticket price: $11.00 Show time: 6:50pm 1917 Ticket price: $14.95 Show time: 8:15pm Enter the name of the movie: little women Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X X X X X X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Enter the rowcol of the seat to purchase: 23 Your ticket is confirmed! Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X T X X X X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Another seat (y/n)?: y Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X T X X X X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Enter the rowcol of the seat to purchase: 23 Sorry! That seat is already taken! Please try again. Another seat (y/n)?: y Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X T X X X X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Enter the rowcol of the seat to purchase: 24 Your ticket is confirmed! Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X T T X X X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Another seat (y/n)?: y Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X T T X X X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Enter the rowcol of the seat to purchase: 24 Sorry! That seat is already taken! Please try again. Another seat (y/n)?: y Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X T T X X X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Enter the rowcol of the seat to purchase: 25 Your ticket is confirmed! Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X T T T X X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Another seat (y/n)?: y Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X T T T X X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Enter the rowcol of the seat to purchase: 26 Your ticket is confirmed! Here are the available seats for Little Women: 1 2 3 4 5 6 7 8 9 1 X X X X X X X X X 2 X X T T T T X X X 3 X X X X X X X X X 4 X X X X X X X X X 5 X X X X X X X X X 6 X X X X X X X X X 7 X X X X X X X X X 8 X X X X X X X X X 9 X X X X X X X X X *******SCREEN****** Another seat (y/n)?: n You ordered 4 tickets for Little Women at 6:50pm. Your total today is: $44.00. Thank you! Please come again.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
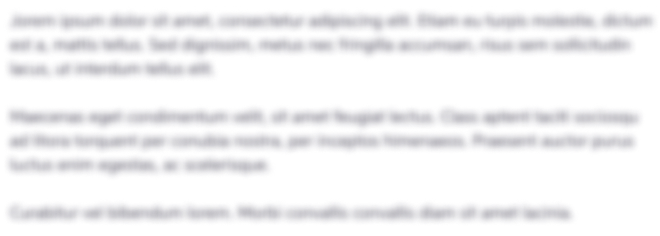
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started