Question
Write Java abstract class called 'Shape' that implements Comparable interface, Add an abstract method to 'Shape' called 'getArea' that returns a type Double. Compiling the
Write Java abstract class called 'Shape' that implements Comparable interface,
Add an abstract method to 'Shape' called 'getArea' that returns a type Double.
Compiling the class 'Shape' will generate compile errors, add additional code in your program to fix the errors
(hint: 'Shape' needs to implement compareTo using the method getArea()).
I.Write Java class called 'Circle' that inherits from the class 'Shape'.
II.Add class instance variable of type Double called radius to "Circle"
III.Add class instance variable of type Double called pi to "Circle", and initialise it to 3.14
IV.Add a constructor to 'Circle' that has a parameter of type Double and sets the value of the class instance variable 'radius' to the value of its parameter.
V.Add the main method to the class. (1 Mark)
VI.In the following code, convert the comments in line 1, 2 and 3 into java code and add all 6 lines to the main method: (3 Mark)
1 //create instance of the class called circle01 with the radius of 1.8
2 //create instance of the class called circle02 with the radius of 1.5
3 //compare the 2 circles based on the value of their area and store the results in a variable called 'comp',
(Hint: use the compareTo method that you implemented in question 2)
4 if(comp>0) System.out.printLn("circle01 is bigger");
5 else if(comp <0) System.out.printLn("circle02 is bigger");
6 else System.out.printLn("circle01 and circle02 are the same size")
VI. In class 'Circle' write method called 'sort' that gets an array of 'Circle' objects, sorts the array (you must use bubble sort algorithm) and returns the sorted array of 'Circle' objects, sorted by the value of their area.
VII. A.In class 'Shape' write method called 'searchShapeByArea' that has 2 Parameters (a ArrayList of type 'Shape' and an object of type 'Shape'). This method must search the contents of the first parameter (the ArrayList) to find the area of an object 'Shape' that matches the area of the second parameter object. The method must return boolean.
(you must use interface 'Iterator' in this method to search the ArrayList)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
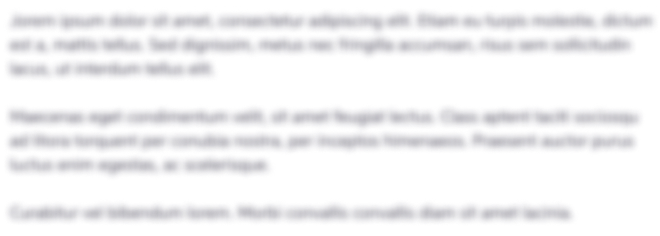
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started