Question
Write Java code, saved as a file called Item.java, which conforms to the behavior in the driver class below. Imagine you're writing a class to
Write Java code, saved as a file called Item.java, which conforms to the behavior in the driver class below.
Imagine you're writing a class to model various surveys on a website. Anyone with a valid login can take various surveys, but their answers will remain anonymous. The class you write should work with the file Driver.java.
public class Driver { public static void main(String[] args){ //setting the customers of the store String[] customers = {"jdoe1", "samsmith", "georgemason", "redcar7", "jane"}; Item.setCustomers(customers); //creating an item the store carries Item candy = new Item("Snickers candy bars", 13.45, 500); Item paperTowels = new Item("Bounty paper towels", 200); //any registered customer (from line 8) can purchase an item System.out.println(candy.purchase("samsmith") == true); System.out.println(paperTowels.purchase("redcar7") == true); System.out.println(paperTowels.purchase("jdoe1") == true); //an item cannot be purchased from an invalid customer System.out.println(paperTowels.purchase("BADUSER") == false); //items can be purchased as long as they are in stock Item flatscreen = new Item("Samsung flatscreen TV", 795, 5); System.out.println(flatscreen.purchase("samsmith") == true); System.out.println(flatscreen.purchase("samsmith") == true); System.out.println(flatscreen.purchase("samsmith") == true); System.out.println(flatscreen.purchase("samsmith") == true); System.out.println(flatscreen.purchase("samsmith") == true); System.out.println(flatscreen.purchase("samsmith") == false); //prints out the names of people who bought an item System.out.println(paperTowels.statistics()); //need getters for these attributes System.out.println(Item.getSoldItemsCount() == 8); } }
Then, use the UnitTests.java below and run them to see how many tests you passed.
import static org.junit.Assert.*; import java.io.BufferedReader; import java.io.FileReader; import java.io.InputStreamReader; import org.junit.Test; public class UnitTests { private static int passed = 0; @Test public void testStatic() { String[] clients = {"james", "kate"}; Item.setCustomers(clients); assertEquals("kate",Item.getCustomers()[1]); assertNotEquals(0,++passed); } @Test public void testCtor1() { Item catFood = new Item("Friskies", 33.23, 3); assertEquals("Friskies $33.23 : [null, null, null]",catFood.statistics()); Item dogFood = new Item("Purina", 43.53, 4); assertEquals("Purina $43.53 : [null, null, null, null]",dogFood.statistics()); assertNotEquals(0,++passed); } @Test public void testCtor2() { Item catFood = new Item("Friskies", 3); assertEquals("Friskies $0.0 : [null, null, null]",catFood.statistics()); Item dogFood = new Item("Purina", 4); assertEquals("Purina $0.0 : [null, null, null, null]",dogFood.statistics()); assertNotEquals(0,++passed); } @Test public void testCount() { Item.setSoldItemsCount(0); Item catFood = new Item("Friskies", 3); Item dogFood = new Item("Purina", 43.53, 4); assertEquals(0,Item.getSoldItemsCount()); assertNotEquals(0,++passed); } @Test public void testPurchase1() { Item.setSoldItemsCount(0); String[] clients = {"james", "kate", "sara"}; Item.setCustomers(clients); Item item = new Item("sugar", 500); for (int i = 0; i < 500; i++) assertEquals(true,item.purchase("james")); assertEquals(false,item.purchase("james")); assertEquals(500,Item.getSoldItemsCount()); assertNotEquals(0,++passed); } @Test public void testPurchase2() { Item.setSoldItemsCount(0); String[] clients = {"james", "kate", "sara"}; Item.setCustomers(clients); Item item = new Item("sugar", 500); assertEquals(false,item.purchase("BADUSER")); assertEquals(0,Item.getSoldItemsCount()); assertNotEquals(0,++passed); } @Test public void testPurchase3() { String[] clients = {"james", "kate", "sara", "john"}; Item.setCustomers(clients); Item item = new Item("sugar", 3); item.purchase("james"); item.purchase("sara"); item.purchase("kate"); assertEquals("sugar $0.0 : [james, sara, kate]",item.statistics()); item = new Item("sugar", 45, 3); item.purchase("james"); item.purchase("sara"); item.purchase("kate"); assertEquals("sugar $45.0 : [james, sara, kate]",item.statistics()); assertNotEquals(0,++passed); } @Test public void countPassed(){ System.out.println(systemCall("curl -k https://cs.gmu.edu/~kdobolyi/sparc/process.php?user=sparc_ZDX12QRX12345678-sample_ass2_1-PROGRESS")); assertEquals(7,passed); if (passed == 7) System.out.println(systemCall("curl -k https://cs.gmu.edu/~kdobolyi/sparc/process.php?user=sparc_ZDX12QRX12345678-sample_ass2_1-COMPLETED")); } public static StringBuffer systemCall(String cmd){ StringBuffer result = new StringBuffer(); try { System.err.println("doing "+ cmd); Runtime run = Runtime.getRuntime(); Process pr = run.exec(cmd); pr.waitFor(); BufferedReader buf = new BufferedReader(new InputStreamReader(pr.getErrorStream())); String line = null; while ((line=buf.readLine())!=null) result.append(line+" "); buf.close(); buf = new BufferedReader(new InputStreamReader(pr.getInputStream())); while ((line=buf.readLine())!=null) result.append(line+" "); buf.close(); pr.getOutputStream().close(); pr.destroy(); } catch (Exception e) { StackTraceElement[] elts = e.getStackTrace(); for(int i=0; i < elts.length; i++) result.append(elts[i].toString()+" "); } return result; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
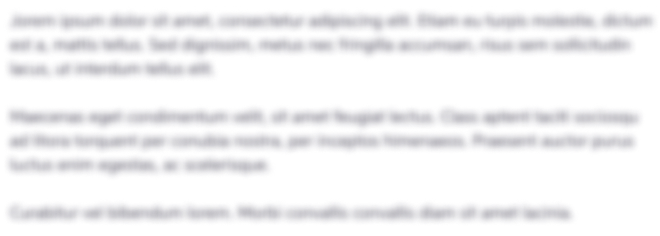
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started