Question
Write the binary representation of these signed two's complement binary numbers, but extended to 16 bits. 01001001 11111001 10000000 Compute the following bitwise operations. ~100111001
Write the binary representation of these signed two's complement binary numbers, but extended to 16 bits.
01001001
11111001
10000000
Compute the following bitwise operations.
~100111001
11100110 & 01110001
11100110 | 01110001
Part 2: Program and Data Representation
1. (Short answer) What is machine language, and how is it related to assembly language?
2. I have a register which contains the value 0xE315DEAD. I use sw to store it to memory. Write the sequence of bytes that would be placed in memory if our computer is using:
- Little-endian integers
- Big-endian integers
3. I have an array where each item is 16 bytes long. If I want to access the 7th item (that is, array[6]), how many bytes do I have to move forward from the beginning of the array?
4. Let's say t3 contains 44 and a1 contains 1054. For the instruction sb t3, (a1), explain what data is copied into what location.
5. In MIPS, when you load a byte from memory into a register:
- What happens to its value? (There are two options.)
- Why do we do this?
This class focuses mainly using MIPS
1. When we call functions, often those functions push registers on the stack and pop them off before they return. Why? (What bad things would happen if they didn't do that?)
2. For the following situations, write what kind of register you would use (a, v, t, s) and why.
x + 1 in the code check_block(x + 1, y).
i in the code for(i = 0; i < 10; i++) { ... }.
row * WIDTH in the code block_arr + row * WIDTH + col.
block_arr + row * WIDTH + col in the code return block_arr + row * WIDTH + col.
The address of my_var in the code my_var = their_var, where my_var is in memory!
Look at the following snippets of code. For each, write some C/Java-like pseudocode to show what the code does.
a.
la t0, ball_x lw t1, (t0) addi t1, t1, 1 sw t1, (t0)
b.
li s2, 0 top: move a0, s2 li v0, 1 # "print int" syscall syscall addi s2, s2, 1 blt s2, 10, top
c.
add a0, t0, t1 add a1, t2, t3 li a2, COLOR_WHITE jal Display_SetLED
d.
lw t0, ball_x ble t0, 2, flip_x blt t0, 61, update_x flip_x: lw t1, ball_vx neg t1, t1 sw t1, ball_vx update_x: lw t1, ball_vx add t0, t0, t1 sw t0, ball_x
Step by Step Solution
There are 3 Steps involved in it
Step: 1
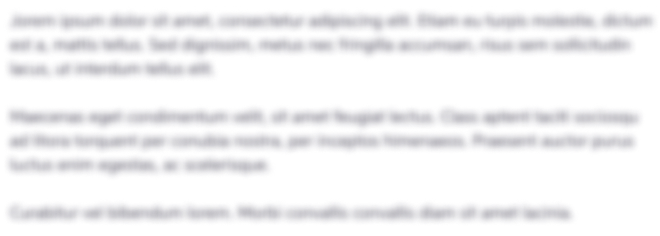
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started