Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write the code for a Python function called dotProduct that accepts a dictionary containing 'n' equal length lists such that each list is stored using
Write the code for a Python function called dotProduct that accepts a dictionary containing 'n' equal length lists such that each list is stored using a unique key. You may assume there are a minimum of 2 keys in the dictionary, but there may be 3, 4, 10, 1000, etc, or more keys. This function performs a dot product multiplication of all lists in the dictionary. In mathematics, the dot product or scalar product is an algebraic operation that takes two equal-length sequences of numbers (usually coordinate vectors), and returns a single number. An example of a dot product of 2 lists [1, 2, 3] and [6, 7, 8] would be: [1, 2, 3] [6, 7, 8] 1 * 6 + 2 * 7 + 3 * 8 = 44 The function prototype MUST be: def dotProduct(myDictParam) : An example of a dot product of 3 lists [-1, 6, 4, 22], [5, 2, 8, -1], and [7, 3, 1, 5], would be: [-1, 6, 4, 22] [ 5, 2, 8, -1] [ 7, 3, 1, 5] -1 * 5 * 7 + 6 * 2 * 3 + 4 * 8 * 1 + 22 * -1 * 5 = -77 NOTE: Your solution MUST use both the lamba and reduce functions.
Using the main below:
import math import random import string import collections import datetime import re import time import copy from functools import reduce # YOUR CODE BELOW... def loadDictionary(myDict, seq1, letter='X') : # your code here... # end def def dotProduct(myDictParam) : # your code here... # end def def integerToRoman(n) : # your code here... # end def def main( ) : letters = ['M', 'X', 'Y', 'Z'] myDict = { } seq1 = [31, 32, 33, 34, 35, 36, 37, 38, 39] seq2 = [5, -3, 8, 17, 1, 9, -4, -2, 7] seq3 = [6, -5, 12, -3, 25, 18, -7, 21, 88] seq4 = [8, 2, 29, 8, 10, 4, 91, 76, 15] newDict = loadDictionary(myDict, seq1, letters[0]) # key of 'M' newDict = loadDictionary(newDict, seq2) # key will use default value of 'X' result = dotProduct(newDict) print(newDict) print("dotProduct with seq1 and seq2: ", result) newDict = loadDictionary(newDict, seq3, letters[2]) # key of 'Y' result = dotProduct(newDict) print(newDict) print("dotProduct with seq1, seq2, and seq3: ", result) newDict = loadDictionary(newDict, seq4, letters[3]) # key of 'X' result = dotProduct(newDict) print(newDict) print("dotProduct with seq1, seq2, seq3, and seq4: ", result) print("keys in final dictionary: ", newDict.keys( )) for i in range(51) : print(integerToRoman(i)) romansList = [ 499, 500, 504, 508, 509, -6, 600, 777, 800, 850, 860, 870, 880, 890, 900, 1000, 1004, 1008, 1009, 1222, -1222, 1400, 1404, 1409, 1889, 1900, 1909, 1929, 1969, 5000, 3450, 3979, 3999, 4000, 4001, 88 ] for i in romansList : print(integerToRoman(i)) # end main( ) if __name__ == "__main__" : main( )
Step by Step Solution
There are 3 Steps involved in it
Step: 1
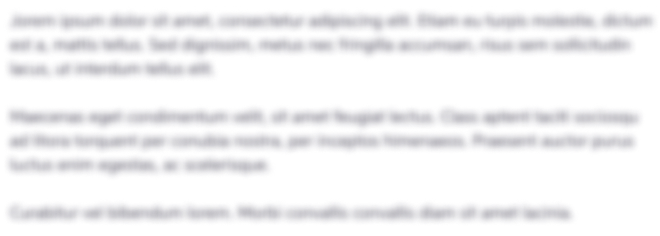
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started