Question
Write the definition of the function moveNthFront that takes as a parameter a positive integer, n. The function moves the nth element of the queue
Write the definition of the function moveNthFront that takes as a parameter a positive integer, n. The function moves the nth element of the queue to the front. The order of the remaining elements remains unchanged. For example, suppose: queue = {5, 11, 34, 67, 43, 55} and n = 3. After a call to the function moveNthFront: queue = {34, 5, 11, 67, 43, 55}. Add this function to the class queueType. Also, write a program to test your method.
I am currentlt displaying---------->
Other queu is not empty
Front element of other queue : 5
queue: 5 9 16 4 2
testing move nth front on queue 2
queue: 4 5 9 16 2
I want to display------------------------->
Other queu is not empty
Front element of other queue : 5
Enter set of numbers to queue //(user types 5 9 16 4 2)
queue1: 5 9 16 4 2
Enter number to be moved //(user types16)
n:16
testing move nth front on queue2
queue2: 16 5 9 4 2
UPDATED PROGRAM:
the user inputs the values for queue1: and for n:
#include
#include
using namespace std;
template
class queueADT
{
public:
virtual bool isEmptyQueue() const = 0;
virtual bool isFullQueue() const = 0;
virtual void initializeQueue() = 0;
virtual Type front() const = 0; //Function to return the first element of the queue.
virtual Type back() const = 0; //Function to return the last element of the queue.
virtual void addQueue(const Type& queueElement) = 0; //Function to add queueElement to the queue.
virtual void deleteQueue() = 0; //Function to remove the first element of the queue.
virtual void moveNthFront(int n) = 0;
};
template
class queueType : public queueADT
{
private:
int maxQueueSize; //variable to store the maximum queue size
int count; //variable to store the number of elements in the queue
int queueFront; //variable to point to the first element of the queue
int queueRear; //variable to point to the last element of the queue
Type *list; //pointer to the array that holds the queue elements
public:
queueType(int queueSize = 100) //Constructor
{
if (queueSize <= 0)
{
cerr << "Size of the array to hold the queue must "
<< "be positive." << endl;
cerr << "Creating an array of size 100." << endl;
maxQueueSize = 100;
}
else
maxQueueSize = queueSize; //set maxQueueSize to queueSize
queueFront = 0; //initialize queueFront
queueRear = maxQueueSize - 1; //initialize queueRear
count = 0;
list = new Type[maxQueueSize]; //create the array to hold the queue elements
}
queueType(const queueType
{
maxQueueSize = otherQueue.maxQueueSize;
queueFront = otherQueue.queueFront;
queueRear = otherQueue.queueRear;
count = otherQueue.count;
list = new Type[maxQueueSize];
//copy other queue in this queue
for (int j = queueFront; j <= queueRear; j = (j + 1) % maxQueueSize)
list[j] = otherQueue.list[j];
} //end copy constructor
~queueType() //Destructor
{
delete[] list;
}
const queueType
{
int j;
if (this != &otherQueue) //avoid self-copy
{
maxQueueSize = otherQueue.maxQueueSize;
queueFront = otherQueue.queueFront;
queueRear = otherQueue.queueRear;
count = otherQueue.count;
delete[] list;
list = new Type[maxQueueSize];
//copy other queue in this queue
if (count != 0)
for (j = queueFront; j <= queueRear; j = (j + 1) % maxQueueSize)
list[j] = otherQueue.list[j];
} //end if
return *this;
}
bool isEmptyQueue() const //Function to determine whether the queue is empty.
{
return (count == 0);
} //end isEmptyQueue
bool isFullQueue() const //Function to determine whether the queue is full.
{
return (count == maxQueueSize);
} //end isFullQueue
void initializeQueue() //Function to initialize the queue to an empty state.
{
queueFront = 0;
queueRear = maxQueueSize - 1;
count = 0;
}
Type front() const //Function to return the first element of the queue.
{
assert(!isEmptyQueue());
return list[queueFront];
}
Type back() const //Function to return the last element of the queue.
{
assert(!isEmptyQueue());
return list[queueRear];
} //end back
void addQueue(const Type& newElement) //Function to add queueElement to the queue.
{
if (!isFullQueue())
{
queueRear = (queueRear + 1) % maxQueueSize; //use mod
//operator to advance queueRear
//because the array is circular
count++;
list[queueRear] = newElement;
}
else
cerr << "Cannot add to a full queue." << endl;
}
void deleteQueue() //Function to remove the first element of the queue.
{
if (!isEmptyQueue())
{
count--;
queueFront = (queueFront + 1) % maxQueueSize; //use the
//mod operator to advance queueFront
//because the array is circular
}
else
cerr << "Cannot remove from an empty queue." << endl;
}
//below is the required function
void moveNthFront(int n) {
Type nth = list[n];
for (int i = n; i>queueFront; --i) {
list[i] = list[i - 1];
}
list[queueFront] = nth;
}
};
void testCopyConstructor(queueType
int main()
{
queueType
int x, y;
x = 4;
y = 5;
queue1.addQueue(x);
queue1.addQueue(y);
x = queue1.front();
queue1.deleteQueue();
queue1.addQueue(x + 5);
queue1.addQueue(16);
queue1.addQueue(x);
queue1.addQueue(y - 3);
testCopyConstructor(queue1);
queue2 = queue1;
cout << "queue1: ";
while (!queue1.isEmptyQueue())
{
cout << queue1.front() << " ";
queue1.deleteQueue();
}
cout << endl;
cout << "Testing move nth front on queue 2 ";
cout << "queue2: ";
queue2.moveNthFront(4);
while (!queue2.isEmptyQueue())
{
cout << queue2.front() << " ";
queue2.deleteQueue();
}
cout << endl;
getchar();
return 0;
}
void testCopyConstructor(queueType
{
if (!otherQueue.isEmptyQueue())
{
cout << "Other Queue is not empty" << endl;
cout << "Front element of Other Queue : "
<< otherQueue.front() << endl;
otherQueue.deleteQueue();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
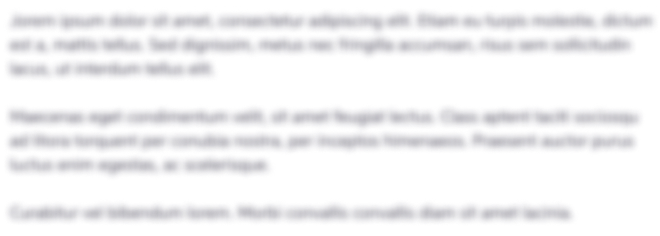
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started