Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write the following in C++ thank you very much!! Implement the struct named Vector in the namespace cs . The interface for Vector must be
Write the following in C++ thank you very much!!
Implement the struct named Vector in the namespace cs. The interface for Vector must be in a separate header file named csvector.h. Code the following functions for Vector starting from the following provided code.
namespace cs { /** * A cs::Vector represents a geometric (AKA Euclidean) vector, with double-precision IEEE 754 * floating-point scalar components (AKA the 'double' type in C++). */ struct Vector { /** The x component of this vector. */ double x = 0; /** The y component of this vector. */ double y = 0; /** The z component of this vector. */ double z = 0; /** * Normalizes this vector to length 1 (making it a unit vector). * * @return this vector by reference, for fluent interface */ Vector &Normalize(); /** Creates and returns a new vector that is this vector, normalized. */ Vector Normalized() const; /** * Calculates the magnitude (AKA length) of this vector. * * @return the magnitude of this vector */ double Magnitude() const; /** * Calculates the squared magnitude (AKA length) of this vector. This function is often used to * improve performance since, unlike magnitude(), it does not require a square-root operation. * * @return the squared magnitude of this vector */ double MagnitudeSquared() const; /** * Sets the components of this vector. * * @param x the new x component * @param y the new y component * @param z the new z component * @return this vector by reference, for fluent interface */ Vector &Set(const double x, const double y, const double z) { this->x = x; this->y = y; this->z = z; return *this; } /** * Limits the magnitude of this vector without changing its direction, if the given limit is * smaller than the current magnitude. This is accomplished by normalizing the vector and treating * the limit as a scalar multiplier. * * @param limit the new magnitude of the vector * @return this vector by reference, for fluent interface * @throws std::invalid_argument if the limit is negative */ Vector &Limit(const double limit); /** * Creates and returns a new vector that is this vector, with magnitude limited in the same * fashion as the Limit function. * * @return the new vector * @throws std::invalid_argument if the limit is negative */ Vector Limited(const double limit) const; /** * Sets the magnitude of this vector without changing its direction. This is accomplished by * normalizing the vector and treating the limit as a scalar multiplier. * * @param magnitude the new magnitude of the vector, if smaller than the current magnitude * @return this vector by reference, for fluent interface * @throws std::invalid_argument if the limit is negative */ Vector &SetMagnitude(const double magnitude); /** * Creates and returns a new vector that is this vector, with magnitude set in the same fashion as * the SetMagnitude function. * * @return the new vector * @throws std::invalid_argument if the limit is negative */ Vector ToMagnitude(const double magnitude) const; /** * Interpolates this vector linearly to another vector. * * @param that the other vector * @param amount the amount of interpolation; some value between 0.0 (current vector) and 1.0 * (other vector). 0.1 is very near the current vector; 0.5 is halfway in between. * @return this vector by reference, for fluent interface * @throws std::invalid_argument if the interpolation amount is outside [0, 1] */ Vector &InterpolateTo(const Vector &that, const double amount); /** * Creates and returns a new vector that is this vector, interpolated linearly to another vector. * * @param that the other vector * @param amount the amount of interpolation; some value between 0.0 (current vector) and 1.0 * (other vector). 0.1 is very near the current vector; 0.5 is halfway in between. * @return the new vector * @throws std::invalid_argument if the interpolation amount is outside [0, 1] */ Vector InterpolatedTo(const Vector &that, const double amount) const; /** Calculates and returns the the distance between this vector and another. */ double DistanceFrom(const Vector &that) const; /** Calculates and returns the angle (in radians) between this vector and another. */ double AngleBetween(const Vector &that); /** Calculates and returns the dot product of this vector and another */ double Dot(const Vector &that) const; /** Calculates and returns a new vector composed of the cross product between two vectors. */ Vector Cross(const Vector &that) const; /** Copies the components of another vector into this vector. */ void operator=(const Vector &that); /** Returns whether this vector's components equal those of another. */ bool operator==(const Vector &that) const; /** Returns whether this vector's components do not equal those of another. */ bool operator!=(const Vector &that) const; /** Returns whether this vector's magnitude is less than another's. */ bool operator<(const Vector &that); /** Returns whether this vector's magnitude is greater than another's. */ bool operator>(const Vector &that); /** Returns whether this vector's magnitude is less than (or equal to) another's. */ bool operator<=(const Vector &that); /** Returns whether this vector's magnitude is greater than (or equal to) another's. */ bool operator>=(const Vector &that); /** Increases this vector's magnitude by 1. */ Vector &operator++(); /** Increases this vector's magnitude by 1. */ Vector operator++(int); { Vector tmp(*this); this->operator++(); return tmp; } /** * Decreases this vector's magnitude by 1. * @throws std::invalid_argument if the resulting magnitude would be negative */ Vector &operator--(); /** * Decreases this vector's magnitude by 1. * @throws std::invalid_argument if the resulting magnitude would be negative */ Vector operator--(int) { Vector tmp(*this); this->operator--(); return tmp; } /** * Creates and returns a new vector in which each component is the sum of this vector's component * and the equivalent component in another vector. */ Vector operator+(const Vector &that); /** * Creates and returns a new vector in which each component is the subtraction of another vector's * component from this vector's equivalent component. */ Vector operator-(const Vector &that); /** * Creates and returns a new vector in which each component is the product of this vector's * component and the equivalent component in another vector. */ Vector operator*(const Vector &that); /** * Creates and returns a new vector in which each component is the sum of this vector's equivalent * component and the given scalar. */ Vector operator+(const double scalar); /** * Creates and returns a new vector in which each component is the subtraction of the given scalar * from this vector's equivalent component. */ Vector operator-(const double scalar); /** * Creates and returns a new vector in which each component is the product of this vector's * equivalent component and the given scalar. */ Vector operator*(const double scalar); /** * Creates and returns a new vector in which each component is the result of dividing this * vector's equivalent component by the given scalar. */ Vector operator/(const double scalar); /** Adds each component of another vector to this vector's equivalent component. */ Vector &operator+=(const Vector &that); /** Subtracts each component of another vector from this vector's equivalent component. */ Vector &operator-=(const Vector &that); /** Multiplies each component of another vector by this vector's equivalent component. */ Vector &operator*=(const Vector &that); /** Adds the given scalar to each of this vector's components. */ Vector &operator+=(const double scalar); /** Subtracts the given scalar from each of this vector's components. */ Vector &operator-=(const double scalar); /** Multiplies each of this vector's components by the given scalar. */ Vector &operator*=(const double scalar); /** Divides each of this vector's components by the given scalar. */ Vector &operator/=(const double scalar); /** * Read-only subscript (0 for x, 1 for y, 2 for z). * @throws std::invalid_argument if index is out of range */ const double operator[](std::size_t index) const; /** * Assignable subscript (0 for x, 1 for y, 2 for z). * @throws std::invalid_argument if index is out of range */ double &operator[](std::size_t index); /** Inserts this vector into an ostream, in the format "(x, y, z)" (without any rounding). */ friend std::ostream &operator<<(std::ostream &out, Vector obj); }; } // namespace cs
Step by Step Solution
There are 3 Steps involved in it
Step: 1
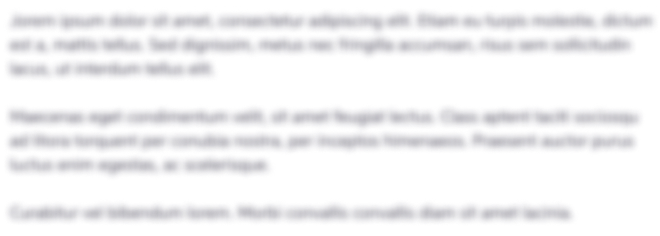
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started