Question
Write the implementation for the credit card class and enumeration that would satisfy the following sample UML diagram and client code and output. Some print
Write the implementation for the credit card class and enumeration that would satisfy the following sample UML diagram and client code and output. Some print statements occur inside the CreditCard class. An empty project has been provided for you with a Program.java class
In the unlikely event you are unfamiliar with a credit card or how it works, it is a financial instrument that let's you make purchases up to a certain limit. Each charge (e.g. you buy something) adds to your balance Each payment reduces your balance. A credit card typically has an interest rate with a compounding frequency (i.e. how often do we calculate interest owed) which can be annual, monthly, daily, or continuously (bad for debt, great for savings). An annual interest rate of 12% compounded monthly would mean that each time we calculated interest (once a month in this case) would use 1% for the interest calculation (12% per year / 12 months per year = 1%).
## Requirements
Components that you will be graded upon:
- Structure matches the UML diagram precisely (I will be unit testing this when I grade)
- Constructor correctly initializes instance variables from the constructor parameters and any other needed initialization
- get Methods for simple instance variables return the correct data (e.g. **getAccountNumber()**)
- The **charge** method which adds to the balance, but it should check and prevent charging any amount that would cause the balance to exceed the limit. Ensure that upon receiving the amount to charge the charge method calls your private round method and rounds the value to 2 places. The method returns true if successful or false if the new charge would cause the balance to be exceeded.
- The **makePayment** method reduces the balance by the amount of the payment.
- The **postInterest** method accepts an InterestCompoundingFrequency enumeration of (Daily,Monthly,Annually). It will use this to calculate the periodic interest rate where the periodic interest rate is the annual interest rate divided by the number of periods.
- For example, if monthly, then annual interest rate / 12 (i.e. months in a year). Daily use 365 periods. Annual is 1 period.
- Keep in mind that the annual interest rate is being passed in the form of 18.9% (for example) and not .189.
- After calculating the interest, but before adding it to balance or total interest accrued, the calculated interest must be rounded to 2 places using your private **round** method.
- The **raiseLimit** method allows for raising or lowering the limit by an incremental amount, not total amount. Example: if current limit is $150, raiseLimit(50) would raise it to $200.
- The **getMinimumPayment** method which calculates and returns the minimum payment at 2% of the current balance
- The **printStatement** prints the formatted output shown at the bottom of the output. Do this last as I may make this extra credit.
- The CreditCard class may not use the System.out directly but must use the PrintStream passed to the constructor.
- The **round** method is a method that rounds a number to a set number of decimal places using the half-up rounding method. How you implement this is up to you. It will impact your interest calculations
## UML Class Diagram
## Sample code in Program.main
```java
String accountNumber = "1234-5678-9012-3456";
String accountHolder = "Joe Gaucho";
double startBalance = 500;
double limit = 1000;
double annualInterestRate = 18.0;
CreditCard card = new CreditCard(
accountNumber,
accountHolder,
startBalance,
limit,
annualInterestRate,
System.out);
System.out.printf("Starting balance is: %f%n", card.getBalance());
boolean success = card.charge(300);
sucess = card.charge(500);
System.out.printf("Current balance is: %.2f%n", card.getBalance());
double minPayment = card.getMinimumPayment();
card.makePayment(minPayment);
card.postInterest(InterestCompoundingFrequency.Monthly);
System.out.printf("After payment and interest, balance is: %.2f. Current limit is: %.2f%n",
card.getBalance(),
card.getLimit());
card.raiseLimit(500);
System.out.printf("Current limit is: %.2f%n", card.getLimit());
card.printStatement();
```
## Output
```
//This is the result of the CreditCard.printStatement() method call
**********
* Account Number: 1234-5678-9012-3456
* Account Holder: Joe Gaucho
* Balance: 795.76
* Limit: 1500.00
**********
```
Credit Card enumeration> ----->Interest CompoundingFrequency accountNumber: String accountHolder: String balance: double limit: double annualInterestRate: double out: PrintStream totalInterestAccrued: double = 0 Daily Monthly Annually + CreditCard(accountNumber:String, accountHolder: String, balance: double, limit: double, annuallnterestRate:double, out: PrintStream) + getLimit(): double raiseLimit(increase: double): void charge amount: double): boolean + makePayment(amount: double): void + getMinimumPayment(): double + getBalance(): double getAccountHolder(): String + setAccountHolder(accountHolder: String): void + getAccountNumber(): String postinterest(frequency: InterestCompoundingFrequency): void getTotalInterestAccrued(): double getAnnualInterestRate(): double printStatement(): void round(value: double, places: int): doubleStep by Step Solution
There are 3 Steps involved in it
Step: 1
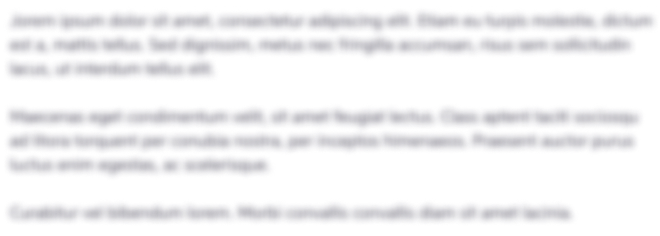
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started