Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write the java subclasses from the attachment instructions from abstarct class which is given below: Find the attachment for the problems and solve them in
Write the java subclasses from the attachment instructions from abstarct class which is given below: Find the attachment for the problems and solve them in Java programming language public abstract class Auction { //description of the item for sale private String itemDescription; //the high bid in the auction private double highBid; //the name of the high bidder private String highBidder; /** * Default constructor */ public Auction() { itemDescription = ""; highBid = 0; highBidder = ""; } /** * Constructor setting description of item for sale * @param description the item for sale */ public Auction(String description) { itemDescription = description; highBid = 0; highBidder = ""; } /** * Specify the description of the item for sale * @param itemForSale the item to be sold */ public void setItem(String itemForSale) { itemDescription = itemForSale; } /** * Get the item description of what is for sale * @return the item description */ public String getItemDescription() { return itemDescription; } /** * Make a bid on the item for sale * @param bid the amount the customer is bidding * @param bidderName the unique name of the bidder placing the bid */ public abstract void bid(double amount, String bidderName); /** * get the name of the current high bidder or empty string if * no public high bidder * @return the name of the current high bidder */ public String getHighBidder() { return highBidder; } /** * get the current high bid * @return the current high bid or zero if there is * no public high bid */ public double getHighBid() { return highBid; } /** * stop this auction i.e., * stop accepting bids on this item */ public abstract void endAuction(); /** * get purchase price * @return the final purchase price for the item or zero * if no bids were placed / the auction is still ongoing */ public abstract double getPurchasePrice(); }
Problem 1: Various Auctions There are many types of auctions, but the basic premise in an auction is that an auctioneer wants to sell an item and they want to ask people to put forth their bids of money. Eventually the auction ends and the highest bidder wins the prize. However there are a few different variants of auctions. The English Auction: In an English auction the auctioneer starts the bidding by asking for bids, and then announces the price to the bidders and asks if anyone is willing to bid higher. If someone wants to bid they yell out their price and show a card giving their bidder identification, if it is higher than the current highest, they become the high bidder. Once the auctioneer feels everyone is done bidding then she strikes the gavel ending the auction. The bidder having yelled out the highest bid is deemed to have bought the item at that price. Example auction: https://www.youtube.com/watch?v-N- no S15cE GOING.. GOING... The Blind Auction: In a blind auction bidders interested in purchasing an item submit one and only one bid without knowing how much everyone else is bidding. They seal their bid into an envelope and give it to the auctioneer. Once everyone who wants to bid has submitted their bids then the auctioneer opens the envelopes and awards the item to the highest bidder and they pay the amount of their bid. If anyone submits multiple bids, only their first bid is considered. If anyone asks about the current highest bid while the auction is not yet ended the answer is 0 and the highest bidder is (empty String) since all the bids are considered only once the auction has ended. The Vickrey Auction: The Vickrey Auctions works exactly the same way as the blind auction, with one exception. In the Vickrey Auction the person bidding the highest amount is deemed to be the winner, however they are only required to pay the amount of the 2nd highest bidder for the item. There are some theories that this encourages people to bid higher since they might win an item but then pay' less for it The Mystery Auction: The Mystery auction is a special variant of an english auction, except this time the bidders do not know what they are bidding on. Only after the auction ends and the winning bidder announced is the item revealed. If anyone asks what item is being sold, this type of auction should always answer "Mystery Item" unless the auction has ended, in that case the actual item name is Example Mystery auction: https://www.youtube.com/watch?v-gTf69_v6xc4&feature-youtu.be&t-104 For this problem you'll create a program that simulates each of the above auctions. You'll implement a Java class hierarchy to aid with code reuse. To get you started on this task the Auction abstract class is provided for you. Along with an example class hierarchy as shown (in photo) You should create a tester class with a method called: abstract Auction public static void testAuction(Auction a) which is capable of testing some basic functionality in any of your auction classes. The grader will further test your code with his/her own tester class. You should further add a public static void main(String I args) which calls your testAuction method for various Auctions. BlindAuction VickreyAuction Problem 1: Various Auctions There are many types of auctions, but the basic premise in an auction is that an auctioneer wants to sell an item and they want to ask people to put forth their bids of money. Eventually the auction ends and the highest bidder wins the prize. However there are a few different variants of auctions. The English Auction: In an English auction the auctioneer starts the bidding by asking for bids, and then announces the price to the bidders and asks if anyone is willing to bid higher. If someone wants to bid they yell out their price and show a card giving their bidder identification, if it is higher than the current highest, they become the high bidder. Once the auctioneer feels everyone is done bidding then she strikes the gavel ending the auction. The bidder having yelled out the highest bid is deemed to have bought the item at that price. Example auction: https://www.youtube.com/watch?v-N- no S15cE GOING.. GOING... The Blind Auction: In a blind auction bidders interested in purchasing an item submit one and only one bid without knowing how much everyone else is bidding. They seal their bid into an envelope and give it to the auctioneer. Once everyone who wants to bid has submitted their bids then the auctioneer opens the envelopes and awards the item to the highest bidder and they pay the amount of their bid. If anyone submits multiple bids, only their first bid is considered. If anyone asks about the current highest bid while the auction is not yet ended the answer is 0 and the highest bidder is (empty String) since all the bids are considered only once the auction has ended. The Vickrey Auction: The Vickrey Auctions works exactly the same way as the blind auction, with one exception. In the Vickrey Auction the person bidding the highest amount is deemed to be the winner, however they are only required to pay the amount of the 2nd highest bidder for the item. There are some theories that this encourages people to bid higher since they might win an item but then pay' less for it The Mystery Auction: The Mystery auction is a special variant of an english auction, except this time the bidders do not know what they are bidding on. Only after the auction ends and the winning bidder announced is the item revealed. If anyone asks what item is being sold, this type of auction should always answer "Mystery Item" unless the auction has ended, in that case the actual item name is Example Mystery auction: https://www.youtube.com/watch?v-gTf69_v6xc4&feature-youtu.be&t-104 For this problem you'll create a program that simulates each of the above auctions. You'll implement a Java class hierarchy to aid with code reuse. To get you started on this task the Auction abstract class is provided for you. Along with an example class hierarchy as shown (in photo) You should create a tester class with a method called: abstract Auction public static void testAuction(Auction a) which is capable of testing some basic functionality in any of your auction classes. The grader will further test your code with his/her own tester class. You should further add a public static void main(String I args) which calls your testAuction method for various Auctions. BlindAuction VickreyAuction
public abstract class Auction
{
//description of the item for sale
private String itemDescription;
//the high bid in the auction
private double highBid;
//the name of the high bidder
private String highBidder;
/**
* Default constructor
*/
public Auction()
{
itemDescription = "";
highBid = 0;
highBidder = "";
}
/**
* Constructor setting description of item for sale
* @param description the item for sale
*/
public Auction(String description)
{
itemDescription = description;
highBid = 0;
highBidder = "";
}
/**
* Specify the description of the item for sale
* @param itemForSale the item to be sold
*/
public void setItem(String itemForSale)
{
itemDescription = itemForSale;
}
/**
* Get the item description of what is for sale
* @return the item description
*/
public String getItemDescription()
{
return itemDescription;
}
/**
* Make a bid on the item for sale
* @param bid the amount the customer is bidding
* @param bidderName the unique name of the bidder placing the bid
*/
public abstract void bid(double amount, String bidderName);
/**
* get the name of the current high bidder or empty string if
* no public high bidder
* @return the name of the current high bidder
*/
public String getHighBidder()
{
return highBidder;
}
/**
* get the current high bid
* @return the current high bid or zero if there is
* no public high bid
*/
public double getHighBid()
{
return highBid;
}
/**
* stop this auction i.e.,
* stop accepting bids on this item
*/
public abstract void endAuction();
/**
* get purchase price
* @return the final purchase price for the item or zero
* if no bids were placed / the auction is still ongoing
*/
public abstract double getPurchasePrice();
}


Step by Step Solution
There are 3 Steps involved in it
Step: 1
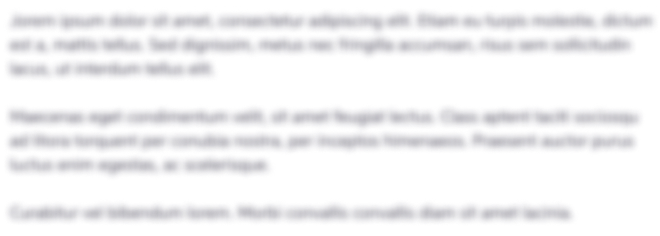
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started