Question
Write the pseudocode of the a function, deleteRecord, to delete a record in the linked list of records. Assume the following variables are already defined,
Write the pseudocode of the a function, deleteRecord, to delete a record in the linked list of records.
Assume the following variables are already defined, initialized and available for you to use:
start: The pointer to the first record of the list (or NULL)
uaccountno: The user's account number (integer)
Assume the following struct definition:
struct record { int accountno; char name[25]; char address[80]; struct record* next; };
Requirements:
Write the pseudocode for the function deleteRecord with the following features:
Must delete a record with the specified account number, AND return 0
Must NOT delete anything if there is not such a record, AND return -1
There must be no use of any C code (including curly braces) in the algorithm
You may ONLY use the allowed vocabulary from the list below with variations to accommodate different memory locations
A solution can be less than 30 lines. Your pseudocode CAN exceed 40 lines, but it would be a bad sign that it may contain some problems.
Vocabulary list:
replace the bold parts with your variables and expressions.
define variable_type called variable_name note: you can newly define ONLY these variable types:
an int
a pointer to record
example: define an int called i define a pointer to record called temp
release the space whose address is in variable_name note: variable_name must be a pointer to record.
example: release the space whose address is in temp
copy from variable_name1 to variable_name2
This copies the value in variable_name1 to variable_name2. If the variables are character arrays, you can assume you can directly copy the characters. You can also assign a result from an expression or a constant value. (be sure about the data type. NULL is for a pointer, not an integer!)
example: copy from temp1 to temp2 copy from string1 to string2 copy from i + 1 to i copy from 10 to i copy from NULL to temp
field_name in the record whose address is in variable_name By this expression, you can access a field inside the record. note1: field_name must be one of the fields in struct record. note2: variable_name must be a pointer to record, but must not be the next field in a record.
example: copy from name in the record whose address is in temp to val copy from val to name in the record whose address is in temp
but DON'T do this!: copy from val to name in the record whose address is in next in the record whose address is in temp
Comparison You can use the following comparisons:
variable_name1 is / is not equal to variable_name2
variable_name1 is less than variable_name2
variable_name1 is greater than variable_name2
while( Comparison ) note1: inside a while loop, put 4 white spaces for indents note2: you CANNOT use a break or continue statement.
example: while( accountno in the record whose address is in temp is equal to 0 ) ... while( accountno in the record whose address is in temp is not equal to 0 ) ...
if( Comparison ) note1: inside an if statement, put 4 white spaces for indents node2: you can use "else".
example: if( var is not equal to 0 ) ... else if( var2 is equal to 0 ) ... else ...
return variable_name This exits the function with the value given by variable_name note 1: variable_name must be an integer note 2: you must not use this statement more than once
Materials to submit
Your submission must include the following:
Pseudocode (.txt) for the addRecord function described above. Since lines of code could be very long, the file must be plain text. See the template below.
Traces that verify the algorithm for the following test cases:
Trying to delete a record in an empty list
Trying to delete a record to a list with three records (the last one is to be deleted)
Trying to delete a record to a list with three records (the list does not have the one specified to delete)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
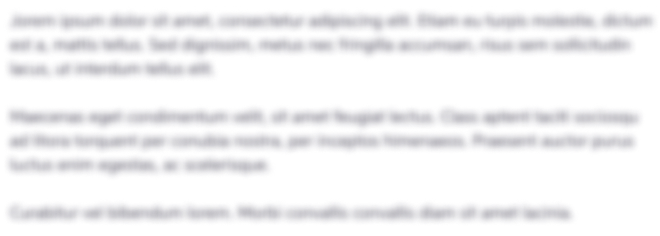
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started