Question
Write this code in Java: Use the two codes given below to create a new class called PhoneBookEntry. Use methods from these two classes to
Write this code in Java:
Use the two codes given below to create a new class called PhoneBookEntry. Use methods from these two classes to come up with the output for this new class. Classes should be in the same package but not the same object. In other words, these classes need to be imported to PhoneBookEntry class. This class runs by calling other two classes from the same package. The state for this class is:
An instance variable of type Name named name
An instance variable of type PhoneNumber named phoneNumber
and the behavior is:
a constructor that accepts a Name object and a PhoneNumber object and assigns them to the corresponding instance variables
A method, call that displays a message that the phone number is begin called (together with an indication of the call is toll-free).
toString, equals, and read methods that correspond to their counterparts in the Name class.
a main method with similar logic to before:
open the file phonebook.text
read in PhonebookeEnry objects
print out the entry
invoke the call method on them
if two adjacent names are the same, but not the numbers, print a message to that effect, but still print out the data for the second entry
if two adjacent entries are the same (name AND number), print out a duplicate error message (as in the previous classes)
keep track of the number of entries processed
The name of your class should be PhonebookEntry. Please remove the public attribute from your class definition (CodeLab restriction).
For example, if the file phonebook.text contains:
Edwards Steven (356)153-9460 Carter Joshua (290)261-7625 Carter Joshua (761)321-9457 Jackson Anthony (724)091-7819 Carter Barbara (759)301-3133 Evans Edward (072)023-0203 White Christopher (060)075-3782 White Christopher (060)075-3782 Taylor Michelle (369)348-9660 Baker Melissa (844)824-5853 Young Anthony (692)839-8466 Hall Michael (216)386-2922
the program should produce the following output:
phone book entry: Steven Edwards: (356)153-9460 Dialing Steven Edwards: (356)153-9460 phone book entry: Joshua Carter: (290)261-7625 Dialing Joshua Carter: (290)261-7625 Warning duplicate name encountered "Joshua Carter: (761)321-9457" discovered phone book entry: Joshua Carter: (761)321-9457 Dialing Joshua Carter: (761)321-9457 phone book entry: Anthony Jackson: (724)091-7819 Dialing Anthony Jackson: (724)091-7819 phone book entry: Barbara Carter: (759)301-3133 Dialing Barbara Carter: (759)301-3133 phone book entry: Edward Evans: (072)023-0203 Dialing Edward Evans: (072)023-0203 phone book entry: Christopher White: (060)075-3782 Dialing Christopher White: (060)075-3782 Duplicate phone book entry "Christopher White: (060)075-3782" discovered phone book entry: Michelle Taylor: (369)348-9660 Dialing Michelle Taylor: (369)348-9660 phone book entry: Melissa Baker: (844)824-5853 Dialing (toll-free) Melissa Baker: (844)824-5853 phone book entry: Anthony Young: (692)839-8466 Dialing Anthony Young: (692)839-8466 phone book entry: Michael Hall: (216)386-2922 Dialing Michael Hall: (216)386-2922 --- 12 phonebook entries processed.
NAME CLASS:
import java.io.*; import java.util.*;
class Name {
public Name(String last, String first) {
this.last = last;
this.first = first;
}
public Name(String first) {this("", first);}
public String getFormal() {return first + " " + last;}
public String getOfficial() {return last + ", " + first;}
public boolean equals(Name other) {return first.equals(other.first) && last .equals(other.last);}
public String toString() {return first + " " + last;}
public static Name read(Scanner scanner) {
if (!scanner.hasNext()) return null;
String last = scanner.next();
String first = scanner.next();
return new Name(last, first);
}
public String getInitials(){
/*getinitials method is created and it returns a string consisting of first and last name initials followed by "."*/
String intials = this.first.charAt(0)+"."+this.last.charAt(0)+".";
return intials;
}
private String first, last;
public static void main(String [] args) throws Exception {
Scanner scanner = new Scanner(new File("names.text"));
int count = 0;
Name name = read(scanner);
while(name != null) {
System.out.println("name: " + name.toString());
System.out.println("formal: " + name.getFormal());
System.out.println("official: " + name.getOfficial());
System.out.println("initials: "+name.getInitials()+" ");
count++;
Name nextname = read(scanner);//read the name from file into a new object named nextname.
if(nextname != null)//if it is not null, execution enters to check while.
while(name.equals(nextname) && nextname != null){
/* if name equals to nextname and nextname not equal to null the loop executes*/
System.out.println("Duplicate name \""+name.first+" "+name.last+"\" discovered");
name = nextname;//assigns nextname to name
nextname = read(scanner);//reads name from file into nextname.
count++;//count is incremented by 1.
}
name = nextname;//name is assigned with nextname
}
System.out.println("---");
System.out.println(count + " names processed.");
}
}
PhoneNumber
import java.io.File; import java.util.Scanner;
class PhoneNumber { private String number;
public PhoneNumber(String number) { this.number = number; }
public String getAreaCode() { return number.substring(1, 4); }
public String getExcahnge() { return number.substring(5, 8);
}
public String getLineNumber() { return number.substring(9, 13);
}
public boolean isTollFree() { if(number.charAt(1)=='8'){ return true; } return false; }
public String toString() { return this.number; }
public boolean equals(PhoneNumber other) { return number.equals(other.number); }
public static PhoneNumber read(Scanner sc) { if (!sc.hasNext()) return null; String number = sc.next(); return new PhoneNumber(number); }
public static void main(String[] args) throws Exception { Scanner sc = new Scanner(new File("numbers.text"));
int count = 0;
PhoneNumber number = read(sc); while (number != null) { PhoneNumber prev = number; System.out.println("phone number: " + number.toString()); System.out.println("area code: " + number.getAreaCode()); System.out.println("exchange: " + number.getExcahnge()); System.out.println("line number: " + number.getLineNumber()); System.out.println("is toll free: " + number.isTollFree());
System.out.println(); count++; PhoneNumber nextname = read(sc);//read the name from file into a new object named nextname.
if(nextname != null)//if it is not null, execution enters to check while.
while(number.equals(nextname) && nextname != null){
/* if name equals to nextname and nextname not equal to null the loop executes*/
System.out.println("Duplicate phone number \""+number+"\" discovered");
number = nextname;//assigns nextname to name
nextname = read(sc);//reads name from file into nextname.
count++;//count is incremented by 1.
}
number = nextname;//name is assigned with nextname
} System.out.println("---"); System.out.println(count + " phone numbers processed."); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
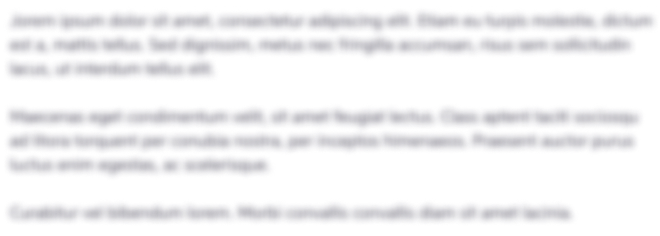
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started