Question
Writing traversal methods You will create two files in the csc403 package: one called A3SimplerBST.java and the other TestA3.java. Details on these programs appears below.
Writing traversal methods
You will create two files in the csc403 package: one called A3SimplerBST.java and the other TestA3.java. Details on these programs appears below.
Copy the file SimplerBST.java from the week 1 examples package and rename the file and class A3SimplerBST. Add two public methods:
- one named twoChildrenCount that takes no parameters and that, when called, traverses every node of the tree to count how many nodes have two children.
- one named sameValueCount that takes a paremeter of generic type V, and that, when called, traverses every node of the tree to count the number of nodes whose value (not its key) is equal to the value passed as a parameter. To check equality you must use the equals method and not the == operator.
As with the get, put, and delete methods, you will need each of the public methods described above to call a corresponding private recursive method.
Next, write a program called TestA3 that:
- Reads the words in the file data/tale.txt into an array using the StdIn.readAllStrings() method.
- Fills a A3SimplerBST symbol table object with counts of words in that array. It should also keep a count of the number of unique words by adding 1 to a counter whenever a word is first added to the symbol table.
- Prints the unique word count, which also is the number of nodes in the BST.
- Calls the twoChildrenCount method for the A3SimplerBST symbol table and prints the value returned.
- Calls the sameValueCount method for the A3SimplerBST symbol table to count and print how many words occur once and then again how many words occur 5 times.
Some difficulty in using node class in methods.
package week1examples;
/* * This is a simplified version of the BST class * from the algs32 package. * */ import stdlib.*; import algs13.Queue;
public class SimplerBST
private static class Node
public Node(K key, V val) { this.key = key; this.val = val; } } public SimplerBST() {}
/* ********************************************************************* * Search BST for given key, and return associated value if found, * return null if not found ***********************************************************************/ // does there exist a key-value pair with given key? public boolean contains(K key) { return get(key) != null; }
// return value associated with the given key, or null if no such key exists public V get(K key) { return get(root, key); } private V get(Node
/* ********************************************************************* * Insert key-value pair into BST * If key already exists, update with new value ***********************************************************************/ public void put(K key, V val) { if (val == null) { delete(key); return; } root = put(root, key, val); }
private Node
/* ********************************************************************* * Delete ***********************************************************************/
public void delete(K key) { root = delete(root, key); } private Node
public void printKeys() { printKeys(root); } private void printKeys(Node
private void inOrder(Node
/* *************************************************************************** * Visualization *****************************************************************************/
public void drawTree() { if (root != null) { StdDraw.setPenColor (StdDraw.BLACK); StdDraw.setCanvasSize(1200,700); drawTree(root, .5, 1, .25, 0); } } private void drawTree (Node
Step by Step Solution
There are 3 Steps involved in it
Step: 1
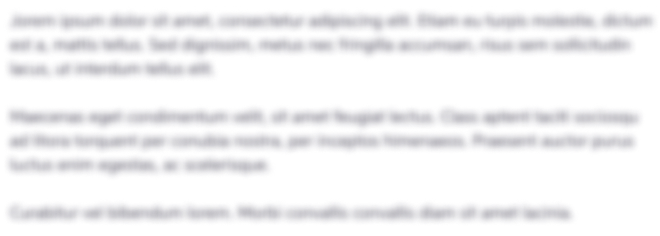
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started