Question
You are asked to write an app to maintain a list of activities that the user likes to participate in. The app should load all
You are asked to write an app to maintain a list of activities that the user likes to participate in. The app should load all activity information from a data file (activities.txt) once the app is started. It allows the user to view, add, remove, and search for activities. The app should save the data back to the same data file when the program exits. Please see Sample Run under Criteria for Success.
What Your Program Should Do
Write an interactive text based menu interface (using a loop) that will allow the user to
Enter information for a new activity.
Display information for all the activities in the database sorted by activity name with index number for each activity.
Display information for all the activities in the database with the same Type.
Remove an activity by index.
Noted:
Use a struct named Activity to model each activity. For the string attributes, such as activity name, activity location, and activity level, you are required to use C-string, a character array terminated by \0. You should use the
For the Type attribute, you can use enum or named integer constants.
For the Rating attribute, you can use integer.
You may not use any while(true) loops or any break statements inside of while loops.
No global variables.
You must do data validation for any required responses and numbers - for example if you have a set of choices for the Type, then you must validate that the user enters a Type within the choice list. You must validate that the rating is between 1 and 10.
Must follow the C++ Style guidelines just like your other assignments.
Use an array of structs to model the collection of activities. You can have a maximum of 30 activities in your list.
Use strstr to do partial comparison to list activities by a location.
See the Inventory example in zyBooks. 15: CS161B: Section 15.2 (strstr and strcmp examples), the video store example in 15: CS161B: Section 15.6 (enums and multiple files and looping menu), and 15:CS161B: Section 15.7, Example to insert sorted into an array. You can find links to these under Criteria for Success.
For submission, your data file should contain at least 10 lines of test data. It should have test cases for different activity names, activity locations and the same location with different activity names.
You must have multiple files for this assignment. activity.h for the struct and the function prototypes, activity.cpp for the function implementations, and main.h and main.cpp for other functions and implementations, and the main() function.
Search for activities by a certain activity name.
Quit
For each activity, you need to keep track of:
Activity name (e.g., Skiing)
Activity location (e.g., Mt Hood Meadows)
Activity Level (e.g., Easy, Difficult, Not for the faint of heart etc.)
Rating (e.g., 1-10 about how fun the experience is)
Type: athletic, food, arts, games, or others - have about 5 different Types of activities for the user to choose from.
Allow the program to keep looping until the user wants to quit. When the program starts, it should load the tasks from an external file (activities.txt) into memory.
When it loads the data into the array, it should insert them sorted alphabetically by activity name. Do not use a sorting algorithm - as you insert the activity into the array, make sure they are inserted in the right position.
When a user enters information about the new activity, the program needs to read them in, and insert them in the correct position.
When the user quits the program, save them in memory and eventually write them to the external data file (activities.txt). The file format could look like: (you can use enum or named constants for Type)
Skiing;Mt Hood Meadows;Difficult;6;0
Wine Making;Umpqua Valley;Complicated;9;1
Catan;Epic Gaming;Easy;4;3
Oil Painting;Fun Studios;Tricky;6;2
Pottery;Fun Studios;Easy;7;2
Snowboarding;Mt Hood Meadows;Not for Faint of heart;8;0
The ';' is used as a delimiter or field separator. Each record ends with a new line character.
The numbers 0, 1, 2, 3, and 4 identify the Type that the activity belongs to. For example, 0 could be Athletics, 1 could be Food, 2 could be Arts, 3 could be Games, and 4 could be Others.
Sample Run
The below sample run is an example of how the output should look. The user responses are bolded
Welcome! This program will help you manage your activities. Pick an option from below: (a)Add a new activity (b)List activities by name (c)List activities by location (d)List activities by Type (e)Remove an activity (f)Search by activity name (q)Quit b 1. Catan;Epic Gaming;Easy;4;Games 2. Oil Painting;Fun Studios;Tricky;6;Arts 3. Pottery;Fun Studios;Easy;7;Arts 4. Skiing;Mt Hood Meadows;Difficult;6;Athletics 5. Snowboarding;Mt Hood Meadows;Not for Faint of heart;8;Athletics 6. Wine Making;Umpqua Valley;Complicated;9;Food Pick an option from below: (a)Add a new activity (b)List activities by name (c)List activities by location (d)List activities by Type (e)Remove an activity (f)Search by activity name (q)Quit p Invalid option!! Please try again! Pick an option from below: (a)Add a new activity (b)List activities by name (c)List activities by location (d)List activities by Type (e)Remove an activity (f)Search by activity name (q)Quit d Enter Type number(0-Athletics, 1-Food, 2-Arts, 3-Games, and 4-Others): 0 Skiing;Mt Hood Meadows;Difficult;6;Athletics Snowboarding;Mt Hood Meadows;Not for Faint of heart;8;Athletics Pick an option from below: (a)Add a new activity (b)List activities by name (c)List activities by location (d)List activities by Type (e)Remove an activity (f)Search by activity name (q)Quit C Enter location name: Hood Meadows 1. Skiing;Mt Hood Meadows;Difficult;6;Athletics 2. Snowboarding;Mt Hood Meadows;Not for Faint of heart;8;Athletics
Pick an option from below: (a)Add a new activity (b)List activities by name (c)List activities by location (d)List activities by Type (e)Remove an activity (f)Search by activity name (q)Quit e 1. Catan;Epic Gaming;Easy;4;Games 2. Oil Painting;Fun Studios;Tricky;6;Arts 3. Pottery;Fun Studios;Easy;7;Arts 4. Skiing;Mt Hood Meadows;Difficult;6;Athletics 5. Snowboarding;Mt Hood Meadows;Not for Faint of heart;8;Athletics 6. Wine Making;Umpqua Valley;Complicated;9;Food
Pick the index to remove: 4 Activity removed! 1. Catan;Epic Gaming;Easy;4;Games 2. Oil Painting;Fun Studios;Tricky;6;Arts 3. Pottery;Fun Studios;Easy;7;Arts 4. Snowboarding;Mt Hood Meadows;Not for Faint of heart;8;Athletics 5. Wine Making;Umpqua Valley;Complicated;9;Food Pick an option from below: (a)Add a new activity (b)List activities by name (c)List activities by location (d)List activities by Type (e)Remove an activity (f)Search by activity name (q)Quit a Enter the activity name (50 characters or less): Rowing Enter the activity location (50 characters or less): Oaks Amusement Park Enter the activity level : Tricky Enter the activity rating : aaa Invalid rating! Please enter a valid rating! Enter the activity rating : 8 Enter Type number(0-Athletics, 1-Food, 2-Arts, 3-Games, and 4-Others): 0 Activity added! 1. Catan;Epic Gaming;Easy;4;Games 2. Oil Painting;Fun Studios;Tricky;6;Arts 3. Pottery;Fun Studios;Easy;7;Arts 4. Rowing;Oaks Amusement Park;Tricky;8;Athletics 5. Snowboarding;Mt Hood Meadows;Not for Faint of heart;8;Athletics 6. Wine Making;Umpqua Valley;Complicated;9;Food Pick an option from below: (a)Add a new activity (b)List activities by name (c)List activities by location (d)List activities by Type (e)Remove an activity (f)Search by activity name (q)Quit f Enter the activity name (50 characters or less): Snowboarding Activity found! 5. Snowboarding;Mt Hood Meadows;Not for Faint of heart;8;Athletics Pick an option from below: (a)Add a new activity (b)List activities by name (c)List activities by location (d)List activities by Type (e)Remove an activity (f)Search by activity name (q)Quit f Enter the activity name (50 characters or less): Skiing Activity not found!! Pick an option from below: (a)Add a new activity (b)List activities by name (c)List activities by location (d)List activities by Type (e)Remove an activity (f)Search by activity name (q)Quit q Activities written to file! Thank you for using my program!! |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
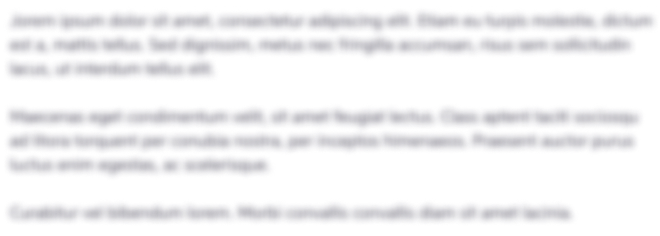
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started