Question
You are going on a long hike, and stop at a store at the trailhead to buy some supplies you will need. Within the function
You are going on a long hike, and stop at a store at the trailhead to buy some supplies you will need. Within the function Inventory() implement the shopping dialog. At the beginning, disp[lay the menu of available items. Report the price of various inventory items based on user's selection. Allow the user more than one selection, but only one of each. After each selection, display the price of the selection and the total cost so far. The user finishes by entering a Return (an empty line). After the user enters Return only, total the price of all selections and display the total cost. Use readKey() in the template code for all key input. All input is one character followed by a Return, and the character entered is the return value from readKey(). The Return character may be either a ' ' or a ' ', depending upon the computer. Most arrays needed are given. If you add variable declarations outside of Inventory(), use the keysword static . Use loops and array subscripting to implement the dialog. Attached is a template to help.
The items have the following prices: Rope: 10 gold Flashlight: 15 gold Climbing Equipment: 25 gold Clean Water: 1 gold A Machete: 20 gold Kayak: 200 gold Food Supplies: 5 gold
The user dialog should look like:
The following items are available: a) Rope b) Flashlight c) Climbing Equipment d) Clean Water e) A Machete f) A Kayak g) Food Supplies Enter a-g (Return) to see the price of the corresponding item (finished, press only Return) a Rope costs 10 silver. Would you like Rope (yn)? y Your total so far is 10 silver Enter a-g (Return) to see the price of the corresponding item (finished, press only Return) b Flashlight costs 15 silver. Would you like Flashlight (yn)? y Your total so far is 25 silver Enter a-g (Return) to see the price of the corresponding item (finished, press only Return) e A Machete costs 20 silver. Would you like A Machete (yn)? y Your total so far is 45 silver Enter a-g (Return) to see the price of the corresponding item (finished, press only Return) b You already have Flashlight Enter a-g (Return) to see the price of the corresponding item (finished, press only Return) f A Kayak costs 200 silver. Would you like A Kayak (yn)? y Your total so far is 245 silver Enter a-g (Return) to see the price of the corresponding item (finished, press only Return) Your final total is 245 silver
Use System.out.printf() and format specifications to make your output look like the above.
import java.io.IOException; public class Assign7 { static String[] items = new String[] { "Rope", "Flashlight", "Climbing Equipment", "Clean Water", "A Machete", "A Kayak", "Food Supplies" }; /* declare a static array to track selected, yes or no */ static int totalCost = 0; static int[] costs = new int[] { 10, 15, 25, 1, 20, 200, 5 }; static char[] choices = new char[] { 'a', 'b', 'c', 'd', 'e', 'f', 'g'}; static char readKey() { int buf, buf2; buf = 0; try { buf = System.in.read(); if (buf == ' ' || buf == ' ') return (char)buf; do { buf2 = System.in.read(); } while (buf2 != ' ' && buf2 != ' '); } catch (IOException iox) { iox.printStackTrace(); System.exit(1); } return (char)buf; } public static void Inventory() { /* assignment code goes here */ System.out.println("Assign7"); } public static void main(String[] args) { Inventory(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
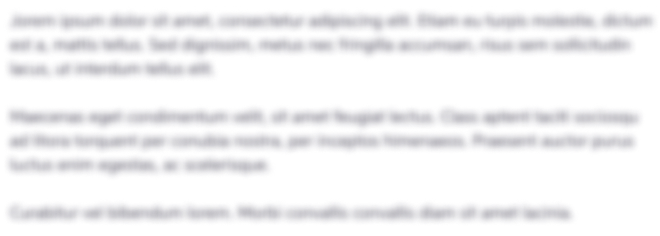
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started