Question
You are taking over a job to build software to keeps track of the standings for the National Hockey League (GAMES). Standings refers to how
You are taking over a job to build software to keeps track of the standings for the National Hockey League (GAMES). Standings refers to how many games hockey teams have won or lost (or in the case of the NHL where their are no ties) also lost in overtime.
The person who was previously tasked with this job has quit, and the hockey season is already underway and so there are already some files left behind by this person that contain the standings up until this current point.
In the NHL a hockey game involves 2 teams and for each team there 3 possible results:
- A team may win the game in regulation (REG), which contributes 2 points towards the winning team's point total for the season and 0 points towards the losing team's point total.
- A team may win the game in overtime (OT), which contributes 2 points towards the winning team's point total and 1 point towards the losing team's point total.
- A team may win the game in a shoot-out (SO), which contributes 2 points towards the winning team's point total and 1 point towards the losing team's point total.
As a point of terminology the NHL considers an OT loss to be any loss in either OT or SO.
As an example consider that the New Jersey Devils (an NHL team) in the 2013-2014 NHL season had 35 wins, 29 losses (in regulation) and 18 over-time losses (either in shoot-out or over-time). Their point-total for that season is shown below:
TEAM. Wins. Losses. OTLosses. Points
New Jersey DEVILS. 35. 29. 18. 88
Every month the NHL will send you the results of recent NHL games (see the provided .csv files, which simulate this) and so your task will be to interpret the results and store them into the same binary standings files that your predecessor created (as well as print them out to screen).
Unfortunately your predecessor (who had this job before you) left on unpleasant terms and took all of their source code with them, leaving you only the binary file that contains the wins, losses and over-time losses for each team (up to the end of October - i.e., they loaded the game-oct.csv file into their binary format before they quit).
You have to write a program that is capable of updating this binary file as new NHL games occur.
Binary File Format:
The binary file that contains the standings has the following format. For each of the X teams in the NHL they have an entry in the binary filestandings.binas follows:
Field. Size in Bytes. Description.
TeamID. 4. 4 byte integer representing the ID of the team for this record
WINS. 8. 8 byte long representing the the number of wins for this team
LOSSES 8. 8 byte long representing the number of losses for this team
OT-LOSSES. 8 8 byte long representing the number of over-time losses for this team
The teams appear in the binary file in the same order as they appear in the nhl-game-data/team_ids.csv file.
For example: the New Jersey DEVILS data is stored in the first record in the binary file that your predecessor has created.
CSV Files:
The TeamID can be reconciled from theteam_ids.csvCSV file containing the associations of int TeamID : String TeamName
shown below a sample fromteam_ids.csv(in thenhl-game-datadir):
id,team_name 1,New Jersey DEVILS 2,NY Islanders ISLANDERS 3,NY Rangers RANGERS 4,Philadelphia FLYERS ...
From the above we see that the id for NEW JERSEY Devils is 1.
The games are given in other csv files all starting with games-X.csv where X is a month prefix from the NHL season.
For example:game-oct.csvcontains all of the games played in October 2013 in the NHL.
(Those extreme NHL hockey fans among us will notice our database doesn't exactly align with the actual NHL schedule from 2013-2014 since some west coast timezone games that finished after midnight are listed on the next day - non-extreme NHL fans don't need to worry about this. Our assignment has all of the actual NHL games from 2013-2014 so the final results you obtain after loading all months should match:https://www.nhl.com/standings/2013/league)
The game csv files are all formatted as follows: date (not important),away team id,home team id, away team goals (not important), home team goals (not important), result
Where most of the data fields are self explanatory except the result which is formatted as follows:[home, away] [win, lose] [REG, SO, OT]
for example, a result equal to:home win REGimplies the home team should get 2 points and the away team gets 0 points added to their cumulative point total. A result ofaway win SOimplies the away team gets 2 points and the home team 1 points (for over-time loss), etc.
Driver Program
Driver Program Name
The Driver must be in a file called:HockeyDriver.java
As part of your assignment you should include a driver program. The driver program takes as input command line arguments of two possible types.
The first is of the form-t Xwhere X is a valid teamID, for example 1 which is the New Jersey DEVILS. On receiving command line arguments-t 1your program should print the current standings for team X (in this case New Jerset DEVILS).
Your standings output for each team should appear all on one line and contain the teams name, wins, losses, OT-Losses and points total. In the example below we also show a header line to aid readability. The unit-tests tests however require the second line to contain the team nameexactlyas shown in theteam_ids.csvand the integer values for wins, losses. ot losses and points (all on the same line).
Example:
TEAM WINS LOSSES OT LOSSES POINTS Winnipeg JETS 5 7 2 12
The second possible command line argument is-f filepath1 filepath2 ...
That is a-ffollowed by some number of ordered filepaths to nhl-game-data csv files.
Upon receiving a-fyour program should read the next argument which will be a game data file (e.g., nhl-game-data/games/nov.csv). Your program should read the game data and add into thestanding.binthe new data. Nowstandings.bincontains update to date game results. Your program should do this for each of the arguments that follow-f.
For example: upon argument input:-f nhl-game-data/nov.csv nhl-game-data/dec.csvYour binary standings.bin file should contain 31 records (one for each nhl team) with each containing the combined original data (from your predecessor the october csv data) plus the november and december data.
If all of the csv data files (except oct) are passed to your program (after the -f flag) then your program should contain the final NHL standings for 2013-2014 and match the following:https://www.nhl.com/standings/2013/league
After your program has combined all of the data files after the-fflag your program should print the standings to the screen. The standings should print in order of point totals (with teams with highest point totals at the top)
example:
TEAM WINS LOSSES OT LOSSES POINTS Boston BRUINS 54 19 9 117 Anaheim DUCKS 54 20 8 116 Colorado AVALANCHE 52 22 8 112 St Louis BLUES 52 23 7 111 San Jose SHARKS 51 22 9 111
IMPORTANT
When printing the standings your program must match the above formatting. One team per line, the team name must match exactly as it is found in the team_ids.csv file and it must contain on the same line as the team name the integer values for wins, losses, OT losses and points.
You may print the above header as well if you wantmarginallynicer output.
Notice in the tests directory some sample unit-tests to help you out.
Deliverables:
A well designed program that achieves the above description. Note that for this assignment we ask you to use Java Streams as part of your solution. It is worth 1/10 points and is up to you where you use them.
HockeyDriver class:
importjava.io.File;
/**
* Handle the assignment command line arguments
*/
publicclassHockeyDriver{
/**
* main program entry point
*@paramargs command line arguments (see output below)
*/
publicstaticvoidmain(String []args) {
//parse the args and take action
if(args.length<2) {
System.out.println("Please provide 2 program arguments");
System.out.println("Example: -t 11 (to display the current standings for team with ID = 11");
System.out.println("Example: -f nhl-game-data"+File.separator+"games-nov.csv (to load new game results and display resulting standings");
System.exit(0);
}
}
}
HockeyStandingsTests class:
importorg.junit.After;
importorg.junit.Before;
importorg.junit.Test;
importjava.io.ByteArrayOutputStream;
importjava.io.File;
importjava.io.IOException;
importjava.io.PrintStream;
importjava.nio.file.Files;
importjava.nio.file.Path;
importjava.nio.file.Paths;
importjava.nio.file.StandardCopyOption;
importjava.util.Scanner;
import staticorg.junit.Assert.assertEquals;
import staticorg.junit.Assert.assertTrue;
/**
* A couple of tests to ensure basic program functionality
*/
publicclassHockeyStandingsTests{
privatefinalByteArrayOutputStream outData=newByteArrayOutputStream();
privatefinalPrintStream origOutput=System.out;
privatefinalStringBASE_DIR="nhl-game-data";
privatefinalStringMINUS_T="-t";
privatefinalStringMINUS_F="-f";
privatefinalStringGAMES_OCT=BASE_DIR+File.separator+"games-oct.csv";
privatefinalStringGAMES_NOV=BASE_DIR+File.separator+"games-nov.csv";
privatefinalStringGAMES_DEC=BASE_DIR+File.separator+"games-dec.csv";
privatefinalStringGAMES_JAN=BASE_DIR+File.separator+"games-jan.csv";
privatefinalStringGAMES_FEB=BASE_DIR+File.separator+"games-feb.csv";
privatefinalStringGAMES_MAR=BASE_DIR+File.separator+"games-mar.csv";
privatefinalStringGAMES_APR=BASE_DIR+File.separator+"games-apr.csv";
privatefinalStringBACKUP=BASE_DIR+File.separator+"standings.backup";
privatefinalStringORIG=BASE_DIR+File.separator+"standings.bin";
@Before
publicvoidinit() {
//the backup should be the original state of the file
//load the backup before each test
//because just like we are working for the weekend
//we better start from the start
Path backup=Paths.get(BACKUP);
Path current=Paths.get(ORIG);
try{
Files.copy(backup, current, StandardCopyOption.REPLACE_EXISTING);
}catch(IOException e) {
e.printStackTrace();
}
System.setOut(newPrintStream(outData));
}
@After
publicvoidrestore() {
System.setOut(origOutput);
System.out.println(outData.toString());
}
@Test
publicvoidtestLoadNovThruApr() {
String[] args={MINUS_F,GAMES_NOV,GAMES_DEC,GAMES_JAN,GAMES_FEB,GAMES_MAR,GAMES_APR};
HockeyDriver.main(args);
String allOutput=outData.toString();
Scanner scan=newScanner(allOutput);
intcount=0;
while(scan.hasNextLine()){
String line=scan.nextLine();
//pick 3 random team totals for the whole season
//but never pick the Boston Bruins we don't need to revisit that
if(line.contains("New Jersey DEVILS")) {
assertTrue(line.contains("88"));
count++;
}
elseif(line.contains("Toronto MAPLE LEAFS")) {
assertTrue(line.contains("84"));
count++;
}
elseif(line.contains("Calgary FLAMES")) {
assertTrue(line.contains("77"));
count++;
}
}
assertEquals(3, count);
}
@Test
publicvoidprintWinnipegOctStandings() {
String[] args={MINUS_T,"11"};
HockeyDriver.main(args);
String allOutput=outData.toString();
Scanner scan=newScanner(allOutput);
intcount=0;
while(scan.hasNextLine()){
String line=scan.nextLine();
//Winnipeg accumulated 12 points in October 2013
if(line.contains("Winnipeg JETS")) {
count++;
assertTrue(line.contains("12"));
}
}
assertTrue(count>0);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
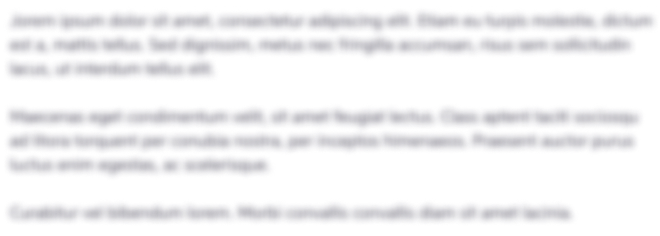
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started