Answered step by step
Verified Expert Solution
Question
1 Approved Answer
You are tasked with enhancing a river quiz game by adding several new features. The game should measure the time taken for the user to
You are tasked with enhancing a river quiz game by adding several new features. The game should measure the time taken for the user to answer each question using std::chrono, and mark answers as incorrect if they take more than seconds, displaying a time limit exceeded message as appropriate.
All random number generation should use std::random with a bit Mersenne Twister initialized once with std::randomdevice. Ensure that a river cannot be regenerated for six calls after it was last generated.
Implement a function getFastest in the Game class to return the players fastest correctly answered questions in nonincreasing order of speed or all if there are fewer than It should return a vector of strings with each string formatted as RiverRivertime with the time recorded in milliseconds.
Additionally, create three modes for random generation using std::discretedistribution:
Each continent is equally likely to be selected.
Each river is equally likely to be selected.
Consecutive calls to the getRandomRiver method should have a probability of producing rivers from the same continent.
Implement a method setGenerationModeint mode in the Game class to handle these customizations. The default mode should be either mode or
For performance, read the files in parallel in the Rivers constructor, protecting against data races where necessary. Also, modify the getContinent function to operate in Ok time, where k is the length of the river string in characters, without using thirdparty libraries.
The code should avoid global variables, excessively long functions, excessive nesting, and repetition. Ensure it is maintainable, readable, extendable, and simple, using templates, classes, and functions where appropriate.
Above is my question and below is my answer what should i change in the code to make it better.
#pragma once
#include "Rivers.h
#include
#include
#define ASSIGNMENTuncomment to switch to assignment
#define RUNPAUSETEST uncomment to run pause test for assignment only
class Game
Rivers r;
further variables...
public:
GameRivers& rivers : rrivers
int getScore return ;
int getTotal return ;
reset score and total to
void reset
this method should implement one round of the game only,
it should return true if the player wishes to continue s or d and false otherwise q
bool playRoundstd::ostream& out, std::istream& in;
std::vector getFastest return std::vector;
;
game.cpp
#include "Game.h
Be sure to refer to in and out NOT cin and cout in this code. Tests will fail otherwise
bool Game::playRoundstd::ostream& out, std::istream& in
return false;
Rivers.h
#pragma once
#include
#include
class Rivers
Insert data structures here...
public:
Time complexity:
Riversconst std::vector& filenames;
Time complexity:
std::string getRandomRiver;
Time complexity:
bool sameContinentstd::string river std::string river;
Time complexity:
std::string getContinentstd::string river;
No need to implement this until assignment
void setModeint mode
;
Rivers.cpp
#include "Rivers.h
Rivers::Riversconst std::vector& filenames
std::string Rivers::getRandomRiver
return ;
bool Rivers::sameContinentstd::string r std::string r
return false;
std::string Rivers::getContinentstd::string river
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
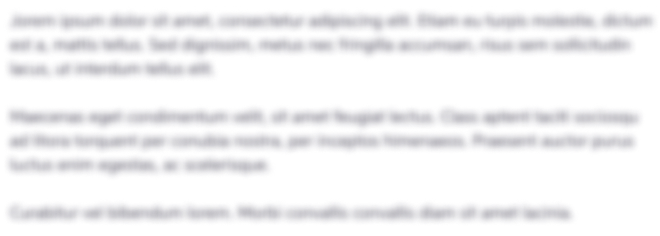
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started