Question
You are to build a depreciation calculator using C++ You are to build a depreciation calculator. Depreciation is an accounting concept and requires yearly cost
You are to build a depreciation calculator using C++
You are to build a depreciation calculator. Depreciation is an accounting concept and requires yearly cost allocations for purchases such as equipment and other material resources used in business. In other words, if a company purchases a piece of equipment (a car or truck would be one example) for $30,000 but expects to use that piece of equipment for several years, it cannot take the full expense of the item in the year it is purchased the expense must be portioned out over the life of the item.
Part A:
The simplest form of depreciation is called straight line. In this form the same amount of expense is recorded each year. Depreciation expenses are always based on the original purchase cost of an item less any salvage value that is expected at the end of the useful life of the item. In straight line depreciation, the difference between cost and salvage value is just divided by the number of years of service expected. In other words, if our $30,000 truck is expected to be worth $5000 after 5 years of useful life, then the depreciation per year is (30000 - 5000) / 5 = $5000 per year. A straight line depreciation schedule would be:
Year Start Value Depreciation End Value
1 30,000 5,000 25,000
2 25,000 5,000 20,000
3 20,000 5,000 15,000
4 15,000 5,000 10,000
5 10,000 5,000 5,000
Note that the following items are always needed: Cost, Salvage Value, and Life of Item
A sample run might be as follows:
Welcome to the depreciation calculator!
Please enter the Asset Cost (0 to quit): 30000
Please enter the Salvage Value: 5000
Please enter the Asset Life (in years): 5
The annual depreciation allowance on a $30,000.00 asset with a salvage value of $5,000.00 and a life of 5 years = $5,000.00 per year under straight-line.
Would you like to see a complete schedule (Y/N)? Y
Year Start Value Depreciation End Value
1 30,000.00 5,000.00 25,000.00
2 25,000.00 5,000.00 20,000.00
3 20,000.00 5,000.00 15,000.00
4 15,000.00 5,000.00 10,000.00
5 10,000.00 5,000.00 5,000.00
Please enter the Asset Cost (0 to quit): 0
Thanks for using the depreciation calculator!
In the above example, the creation of a full schedule is a user option. Also note that the program loops to calculate as many assets as needed. If a schedule is requested, be sure to format the numeric values in a neat columnar display (see recorded lecture for details).
Code the program using an Asset class. The class should be instantiated with the three input values and should have the following functions (methods):
getOrigCost which returns the asset cost sent at instantiation
getOrigSalvage which returns the salvage value
getOrigLife which returns the life of the asset (in years)
getAnnualDep which returns the annual depreciation amount
getBegBal(y) which returns the beginning balance of the asset in year y of its life
getEndBal(y) which returns the ending balance of the asset in year y
Follow the texts guideline for building a class header file and separate cpp file for actual class operations. As always, zip the entire MS C++ .NET project and submit it through the digital drop-box.
Part B:
Modify your Asset class so that it is capable of calculating Double Declining depreciation as well as straight line. This will require you to have an additional set of return methods: getDDDep(y), getDDBBal(y), and getDDEBal(y) for returning the double declining depreciation for any year y. The Annual Depreciation return function will now be overloaded as getAnnualDep(y) since the double-declining depreciation changes from year-to-year. The main control program will also, of course, need to ask the user which type of depreciation schedule is desired (Straight-line or Double
Declining).
I need help with Part B, I currently have the below:
Asset.h
#pragma once
class Asset
{
public:
Asset(double Cost, double Salvage, int life);
~Asset(void);
double getOrigCost(), getOrigSalvage(), getAnnualDep();
double getBegBal(int year), getEndBal(int year);
int getOrigLife();
private:
double ocost, osalv, anndep;
int olife;
double* bbal;
double* ebal;
};
Asset.cpp
#include "stdafx.h"
#include "Asset.h"
Asset::Asset(double c, double s, int l)
{
ocost = c;
osalv = s;
olife = l;
anndep = (c - s) / l;
//declare arrays for starting and ending values
bbal = new double[olife];
ebal = new double[olife];
//calculate straight line depreciation values
bbal[0] = ocost;
for (int i = 0; i < olife; i++)
{
if (i > 0)
{
bbal[i] = ebal[i - 1];
}
ebal[i] = bbal[i] - anndep;
}
}
double Asset::getAnnualDep()
{
return anndep;
}
double Asset::getOrigCost()
{
return ocost;
}
double Asset::getOrigSalvage()
{
return osalv;
}
int Asset::getOrigLife()
{
return olife;
}
double Asset::getBegBal(int y)
{
return bbal[y-1];
}
double Asset::getEndBal(int y)
{
return ebal[y-1];
}
Asset::~Asset(void)
{
}
Depreciation.cpp
// Depreciation.cpp : main project file.
#include "stdafx.h"
#include
#include "Asset.h"
using namespace std;
using namespace System;
int main()
{
//normal input tasks go here - with full data validation
Asset a = Asset(30000.00,2000.00,7);
cout << "The annual depreciation = " << a.getAnnualDep() << endl;
for (int i = 1; i <= a.getOrigLife(); i++)
{
cout << i << "\t" << a.getBegBal(i) << " " << a.getAnnualDep() << " " << a.getEndBal(i) << endl;
}
system("Pause");
return 0;
}
The above example under Part B would look like this:
Welcome to the depreciation calculator!
Please enter the Asset Cost (0 to quit): 30000
Please enter the Salvage Value: 5000
Please enter the Asset Life (in years): 5
The annual depreciation allowance on a $30,000.00 asset with a salvage value of $5,000.00 and a life of 5 years = $5,000.00 per year under straight line or $12,000.00 first year depreciation under double-declining.
Would you like to see a complete schedule for Straight-Line, DDL, or Neither? (S/D/N)? D
Year Start Value Depreciation End Value
1 30,000.00 12,000.00 18,000.00
2 18,000.00 7,200.00 10,800.00
3 10,800.00 5,000.00 5,800.00
4 6,480.00 800.00 5,000.00
5 0.00 0.00 5,000.00
Please enter the Asset Cost (0 to quit): 0
Thanks for using the depreciation calculator!
Notes on Double Declining depreciation:
In this alternate method, you still use purchase price and salvage value, but the depreciation calculated each year is at twice the rate of straight line (but not exactly twice the amount): the catch here is that the calculation takes the straight-line rate doubles it, and then applies that rate to the previous-years ending balance. Typically, at some point the double-declining method will produce yearly depreciation amounts below straight line but instead of continuing with the declining method, at that point the straight line amount is used instead. Using our example, we first see that the straight line method = 20% per year (100% divided by 5 years); the double-declining method would thus be 40% per year but based on the changing ending asset values in each year. Thus, a pure double-declining schedule would be:
Year Start Value Depreciation End Value
1 30,000 12,000 (=30,000 * .4) 18,000
2 18,000 7,200 (=18,000 * .4) 10,800
3 10,800 4,320 (=10,800 * .4) 6,480
4 6,480 1,480 ** 5,000
5 --no depreciation allowed--
Note that in year 4, the calculated depreciation would have been 2592 (6,480 * .4), but only 1480 was allowed because that brought the asset value down to its salvage value level. Even though the asset is used in year 5, there is no taxable depreciation expense allowed. Note again, however, that in year 3 the calculated depreciation is actually less than the straight-line amount would be for that year (4,320 vs. 5,000); at that point, depreciation is usually switched to straight-line, so the actual schedule calculated should have been:
Year Start Value Depreciation End Value
1 30,000 12,000 (=30,000 * .4) 18,000
2 18,000 7,200 (=18,000 * .4) 10,800
3 10,800 5,000 5,800 (5000 > [10,800 * .4])
4 6,480 800 5,000
5 --no depreciation allowed-
Again, the salvage value is as low as the ending balance is permitted to go.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
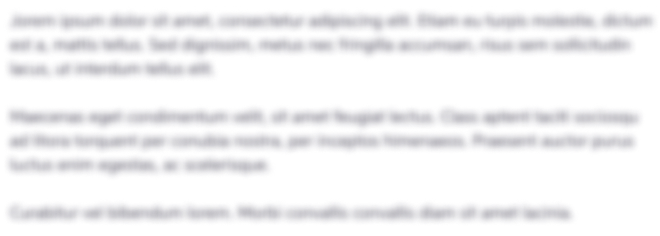
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started