Question
You are to create a class called Complex that supports complex numbers andassociated complex number functions. For more information on complex numbers andrelated functionality refer
You are to create a class called Complex that supports complex numbers andassociated complex number functions. For more information on complex numbers andrelated functionality refer to https://en.wikipedia.org/wiki/Complex_number.The Complex.h and Complex.cpp files are provided to you. You cannot change any functiondefintions. There are 15 test cases to pass and 5 additional for the extra credit.The results are captured in the Complex-results.txt file in the working directory.The four files provided will initially compile together.The complex number must be constructed using a linked list and the Node structurethat is defined in the Complex.h file. The "first" pointer points to the first node of thenumber (linked list). The Node structure has a next pointer that points tothe next node and is nullptr if the last node in the list. The Node structure has datafield and a type field to determine whether the node is the imaginary part or the real part.Each number must be constructed in an efficient way. That is, if the complex numberhas a 0 for the real or imaginary part, the node for that part must not exist. Therefore,these complex numbers have the following list/node structures:0 no nodes (first equal to nullptr).1. + 2i two nodes, one for the real and one for the imaginary part.2i one node, one for the imaginary part.2. one node, one for the real part.You can use any code from the homework #2 answers to help with your implementation.The functions to implement are as follows:
Complex() - default constructor. Creates a zero complex number
.2) Complex(ListElement r, ListElement i) - constructor, creates an r + i complex number.
3) ~Complex() - Destructor, deallocates memory.
4) Complex(const Complex & origList) - Copy constructor.
5) Complex & Complex::operator=(const Complex & rightHandSide) - assignment operator.
6) double getModulus() - returns the modulus of the complex number.
7) getComplex(ListElement rv[]) - The input is an array of length 2. The real part of thecomplex number is written to rv[0] and the imaginary part is written to rv[1].
8) getConjugate(ListElement rv[]) - the conjugate of the complex number is written to therv array. The real part of the complex number is written to rv[0] and the imaginary part iswritten to rv[1].
9) Complex operator+(const Complex & x, const Complex & y) - adds two complex numbers togetherand returns the result as a complex number.
10) Complex operator*(const Complex & x, const Complex & y) - multiplies two complex numbers togetherand returns the result as a complex number.Implementation Hint: Implement the Complex(ListElement r, ListElement i) constructor and then thegetComplex(ListElement rv[]) function first. This will allow you to pass the first test cases.Extra Creditdouble getPhase() - this returns the phase of the complex number in degrees. If x is the return valuethen x is defined in degrees as 180 <= x < -180
ALL FUNCTIONS NEED TO BE IMPLETEMENT HERE
COMPLEX.CPP
#include "Complex.h"
#include
#include
#include
void Complex::change() // used for testing, do not alter
{
if (first != nullptr)
{
first->data = first->data + 1;
}
}
Complex::Complex()
{
}
Complex::Complex(ListElement r, ListElement i) : first(nullptr)
{
}
Complex::~Complex()
{
}
//--- Definition of copy constructor
Complex::Complex(const Complex & origList)
{
}
//--- Definition of assignment operator
const Complex & Complex::operator=(const Complex & rightHandSide)
{
return *this;
}
double Complex::getModulus() const
{
return 0;
}
void Complex::getComplex(ListElement rv[]) const
{
}
void Complex::getConjugate(ListElement rv[]) const
{
}
Complex operator+(const Complex & x, const Complex & y)
{
Complex c;
return c;
}
Complex operator*(const Complex & x, const Complex & y)
{
Complex c;
return c;
}
double Complex::getPhase() const
{
double PI = 3.14159265358979323846;
return 0;
}
USE COMPLEX.H
#ifndef COMPLEX_H
#define COMPLEX_H
class Complex
{
public:
typedef double ListElement;
private:
enum NumberType { REAL = 1, IMAGINARY = 2 };
class Node
{
public:
ListElement data;
NumberType type;
Node * next;
};
Node *first; // pointer to first element in linked list
public:
FOR THE TEST CASE : USE COMPLEX.CPP
Page
of 6
ZOOM
#include
#include
#include
#include "MyFileIO.h"
#include "Complex.h"
void testCases();
int main()
{
testCases();
return 0;
}
void testCases()
{
std::string FILENAME = "Complex-results.txt";
std::string outString;
std::string PASSED = " Passed";
std::string FAILED = " Failed";
std::string TEST_CASE = "Test Case #";
int testCount = 1; // used to track test numbers
char ch[100000]; // array large enough for the file output
int char_ptr = 0; // file index pointer
double error = 0.00001;
Complex::ListElement rv1[2];
double mod;
Complex a;
Complex b(3, -4);
Complex c(0, 3);
Complex d(3, 0);
//Test Case #1 - test default constructor
a.getComplex(rv1);
if ((rv1[0] == 0) && (rv1[1] == 0))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #2 - test parameter constructor
b.getComplex(rv1);
if ((rv1[0] == 3) && (rv1[1] == -4))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #3 - test parameter constructor
c.getComplex(rv1);
if ((rv1[0] == 0) && (rv1[1] == 3))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #4 - test parameter constructor
d.getComplex(rv1);
if ((rv1[0] == 3) && (rv1[1] == 0))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #5 - test modulus
mod = a.getModulus();
if ((mod >= (0 - error)) && (mod <= (0 + error)))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #6 - test modulus
mod = b.getModulus();
if ((mod >= (5 - error)) && (mod <= (5 + error)))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #7 - copy constructor
Complex e(b);
e.getComplex(rv1);
if ((rv1[0] == 3) && (rv1[1] == -4))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #8 - copy constructor shallow copy
e.change();
b.getComplex(rv1);
if ((rv1[0] == 3) && (rv1[1] == -4))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #9 - assignment operator
Complex f;
f = b;
f.getComplex(rv1);
if ((rv1[0] == 3) && (rv1[1] == -4))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #10 - assignment operator shallow copy
f.change();
b.getComplex(rv1);
if ((rv1[0] == 3) && (rv1[1] == -4))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #11 - assignment operator self assignment
c = c;
c.getComplex(rv1);
if ((rv1[0] == 0) && (rv1[1] == 3))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #12 - addition operator
f = b + b;
f.getComplex(rv1);
if ((rv1[0] == 6) && (rv1[1] == -8))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #13 - multiply
Complex g;
g = b * b;
g.getComplex(rv1);
if ((rv1[0] == -7) && (rv1[1] == -24))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #14 - conjugate
b.getConjugate(rv1);
if ((rv1[0] == 3) && (rv1[1] == 4))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #15 - conjugate
d.getConjugate(rv1);
if ((rv1[0] == 3) && (rv1[1] == 0))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #16 - getPhase
Complex aa(-4, 0);
mod = aa.getPhase();
if ((mod >= (180 - error)) && (mod <= (180 + error)))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #17 - getPhase
Complex bb(-4, 4);
mod = bb.getPhase();
if ((mod >= (135 - error)) && (mod <= (135 + error)))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #18 - getPhase
Complex cc(4, 4);
mod = cc.getPhase();
if ((mod >= (45 - error)) && (mod <= (45 + error)))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #19 - getPhase
Complex dd(4, -4);
mod = dd.getPhase();
if ((mod >= (-45 - error)) && (mod <= (-45 + error)))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
//Test Case #20 - getPhase
Complex ee(-4, -4);
mod = ee.getPhase();
if ((mod >= (-135 - error)) && (mod <= (-135 + error)))
outString = TEST_CASE + valueOf(testCount) + PASSED +
getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED +
getCRLF();
std::cout << outString;
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
outString = getCRLF();
char_ptr = build_file_array(outString, char_ptr, ch);
outString = getCode(char_ptr, ch);
char_ptr = build_file_array(outString, char_ptr, ch);
outString = nowtoString();
char_ptr = build_file_array(outString, char_ptr, ch);
writefile(FILENAME, ch, char_ptr);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
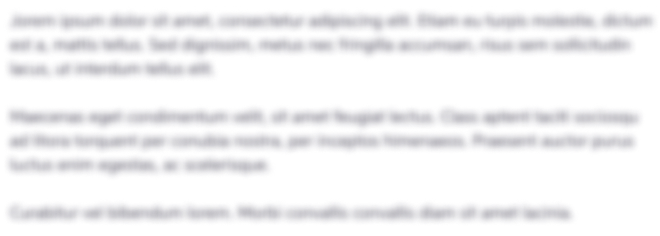
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started