Question
You can create instances of types that are defined as an interface . True False 2. You are writing a JUnit class to test the
You can create instances of types that are defined as an interface.
| True |
| False |
2. You are writing a JUnit class to test the constructor of a Rectangle class. The Rectangle class constructor that you are testing looks like this:
public Rectangle(int length, int width){ if (length < 0 || width < 0) { throw new IllegalArgumentException();} this.length = length; this.width = width; }
You write the following test to determine if the class throws an IllegalArgumentException correctly, but you are not sure where you should write a fail command.
@Test public void test02_constructor_exception() { try { Rectangle j = new Rectangle(-1, 3); // line 5 } catch (IllegalArgumentException e) { // line 7 } catch (Exception e) { // line 9 } // line 11 } Choose all of the following places you should add a fail("..."); command to your test.
| line 7 |
| line 9 |
| line 5 |
| line 11 |
Question 34 pts
In this program below, which lines would lead to compiler errors? Try determine your answers without using Eclipse...then, check your answers in Eclipse.
public class MyOuterClass { private int outer; private class MyInnerClass { private int inner; private MyInnerClass() { inner = 2; } // constructor private void setInner(int s) { inner = s;} }// MyInnerClass public MyOuterClass() { } // constructor public void method1() { MyInnerClass innerObject = new MyInnerClass(); // line 11 innerObject.inner = 12; // line 12 innerObject.outer = 13; // line 13 innerObject.setInner(14); // line 14 inner = 15; // line 15 outer = 16; //line 16 } public static void main(String [] args) { MyInnerClass innerObject = new MyOuterClass(); // line 19 MyOuterClass outerObject = new MyOuterClass(); // line 20 outerObject.method1(); // line 21 } }
Choose all of the following lines that would give a compiler error:
| line 20 |
| line 15 |
| line 12 |
| line 19 |
| line 21 |
| line 13 |
| line 14 |
| line 11 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
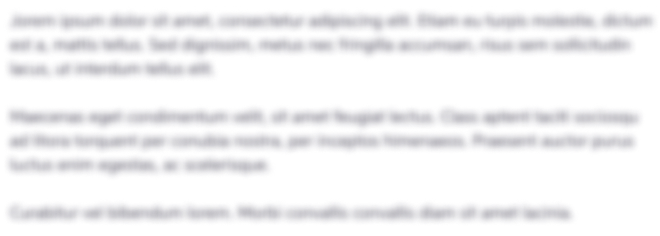
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started