Question
You can start with either implementing an insertion sort algorithm yourself (https://www.geeksforgeeks.org/insertion-sort/). Modify the program such that it now sorts strings (array of characters) instead
You can start with either implementing an insertion sort algorithm yourself (https://www.geeksforgeeks.org/insertion-sort/). Modify the program such that it now sorts strings (array of characters) instead of integers and uses dynamic memory allocation to allocate memory based on the number of elements in the array as well as the length of individual strings.
You can use scanf function to first read the number of strings that will be entered (say, N) and then allocate an array of size N that stores a pointer to a character. Then use a loop to read each string, determine the length of the string, and then allocate appropriate amount of memory for each string, store the reference of the memory allocated in the array of pointers created earlier, and then copy the string read into the allocated memory. Note that you have make an explicit copy of the string that you read into the memory allocated since the buffer used to read the string will be overwritten when the next string is read. Remember to free the memory allocated for each string and the array of pointers.
Use separate functions to read the strings, sort the strings, and display the sorted strings. Here is a sample function prototype:
/* function to read an array of strings */
void readarray(char *arr[], int N);
/* function to print an array of strings */
void printarray(char *arr[], int N);
/* function to sort the array of strings using insertion sort algorithm */
void insertionsort(char *arr[], int N);
Use appropriate string functions provided by the C library. A list of functions and details about each function can be found at: https://en.cppreference.com/w/c/string/byte Links to an external site.. You can use some sample input and output files for testing your code. Sample input and output files are provided here:
/input.txt
10
John
William
James
Charles
George
Frank
Joseph
Thomas
Henry
Robert
Step by Step Solution
There are 3 Steps involved in it
Step: 1
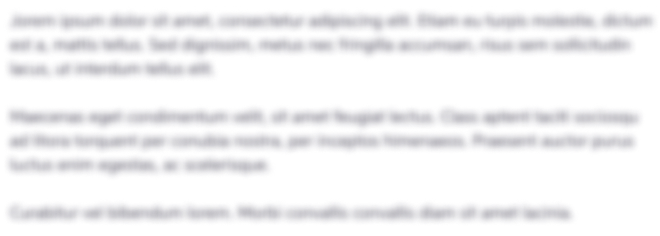
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started