Question
You have already written a Fraction class for a previous project. You will now be given a stripped down Fraction.java as a starter file. You
You have already written a Fraction class for a previous project. You will now be given a stripped down Fraction.java as a starter file. You will enhance it by implementing the Comparable interface. You must implement this interface using Generics. As such the signature of your Fraction class must now read as follows in the given Fraction.java
public class Fraction implements Comparable
If you look in the Java API (Google: Java 9 API Comparable) and scroll down you will eventually come to a section that tells you that by implementing Comparable you are required to write a method in your Fraction class that has this signature:
public int compareTo( Fraction other )
This method will return some int value less than 0 if this Fractions is less than the other Fraction. It should return 0 if the two Fractions are equal, and should return some int value greater than 0 if the other Fraction is greater than thisFraction. Most implementers just return -1, 0 or 1 so you can just do the same.
It is not sufficient to just write some code in the compareTo() method that creates a double value ratio of the numer/denom and then just directly compare it the other numer/denom in double form. Do you understand why this is vulnerable to rounding error? You must compare the numer and denom of the two fractions and determine which is bigger based on that. Do not covert to double or float anywhere in compareTo.
Notice you are given a new version of the toString method in your Fraction.java starter file not only echoes the numer and denom but also tacks on a double representation of the quotient. This double at the end of the print just makes it easier by eye to verify they values are sorted or not. Do not do the actual comparison via doubles.
Here is your FractionTester.java. and a stripped down to the bare bones Fraction.java file that will serve as a starting point.
You do not need to write any public methods that are not used by the main tester program. You may have to write private methods that are used internally to do the required job.
This tester generates several Fractions and inserts them into an ArrayList and then into a plain array. In order to pass an ArrayList into Collections.sort(), it must contains elements that are of a type that has a sorting relation defined on them. This is accomplished by implementing the Comparable interface in the Fraction class. The same is true for a plain array being passed in Arrays.sort(). Both of these sort methods require that the data type of the elements implement Comparable.
Here is the starter file:
public class Fraction implements Comparable { private int numer; private int denom; // ACCESSORS (SETTERS) public int getNumer() { return numer; } public int getDenom() { return denom; } // MUTATORS (GETTERS) public void setNumer( int n ) { numer = n; } public void setDenom( int d ) { if (d==0) { System.out.println("Can't have 0 in denom"); System.exit(0); } else denom=d; } // FULL CONSTRUCTOR - an arg for each class data member public Fraction( int n, int d ) { int gcd = gcd( n, d ); setNumer(n/gcd); setDenom(d/gcd); } private int gcd( int n, int d ) { int gcd = n 0 ) // NOT EFFICIENT AS EUCLID BUT SIMPLE if (n%gcd==0 && d%gcd==0) return gcd; else --gcd; return 1; // they were co-prime no GCD exceopt 1 :( } // COPY CONSTRUCTOR - takes ref to some already initialized Comparable object public Fraction( Fraction other ) { this( other.getNumer(), other.getDenom() ); // call my full C'Tor with other Fraction's data } // REQUIRED BY THE COMPARABLE INTERFACE // if this == other return 0; if this>other return 1; else return -1 public int compareTo( Fraction other ) { // you are only allowed to define one variable in this method // that variable should be a Fraction whose value is this - other // HINT: copy in your subtract() method from project 5 and RE-USE it not rewrite it // now you can just examin the numer and denom of your diff fraction // to determine that fraction is postive negative or 0 // return 0 -1 or 1 accordingly // NO OTHER VARIABLES Of ANY KIND // NO DOUBLES, NO CASTING return 0; // REPLACE WITH YOUR CODE } public String toString() { return getNumer() + "/" + getDenom() + "\t=" + + ((double)getNumer()/(double)getDenom()); } }// EOF
Here is what the output should look exactly like:
here is the sutract method from Project 5:
public Fraction subtract(Fraction f) { int a=this.numer; int b=this.denom; int c=f.numer; int d=f.denom; int num=(a*d - b*c); int den=(b*d); Fraction frac=new Fraction(num,den); frac.reduce(); return frac; }
Here is the tester file you need to use:
/* FractionTester.java A program that declares Fraction variables We will test your Fraction class on THIS tester. */ public class FractionTester { public static void main( String args[] ) { // use the word Fraction as if were a Java data type Fraction f1 = new Fraction( 44,14 ); // reduces to 22/7 System.out.println( "f1=" + f1 ); // should output 22/7
Fraction f2 = new Fraction( 21,14 ); // reduces to 3/2 System.out.println( "f2=" + f2 ); // should output 3/2
System.out.println( f1.add( f2 ) ); // should output 65/14 System.out.println( f1.subtract( f2 ) ); // should output 23/14 System.out.println( f1.multiply( f2 ) ); // should output 33/7 System.out.println( f1.divide( f2 ) ); // should output 44/21 System.out.println( f1.reciprocal() ); // should output 7/22
} // END main } // EOF
ArrayList OF FRACTIONS UNSORTED: 11/3 3.6666666666666665 95/17 5.588235294117647 93/94 0.9893617021276596 5/16 0.3125 2/3 0.6666666666666666 plainArr OF FRACTIONS UNSORTED: 11/3 3.6666666666666665 95/17 5.588235294117647 93/94 0.9893617021276596 5/16 0.3125 2/3 0.6666666666666666 ArrayList OF FRACTIONS SORTED: 5/16 0.3125 2/3 93/94 0.9893617021276596 11/3 3.6666666666666665 95/17 5.588235294117647 0.6666666666666666 plainArr OF FRACTIONS SORTED 5/16 0.3125 2/3 93/94 0.9893617021276596 11/3 3.6666666666666665 95/17 5.588235294117647 0.6666666666666666Step by Step Solution
There are 3 Steps involved in it
Step: 1
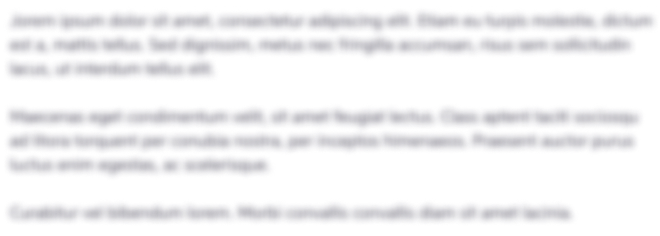
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started