Question
You have been asked to write a simulation program for an airport. The simulation will specify the number of runways and generate a file representing
You have been asked to write a simulation program for an airport. The simulation will specify the number of runways and generate a file representing scheduled flight arrivals and departures. A runway can only be assigned to one flight at a time. All arrivals and departures will reserve the runway for a specified amount of time respectively.
Since it is more expensive and dangerous to keep a plane waiting to land, arrivals have priority over departures. This means that a runway will be assigned to a plane ready to land before being assigned to any plane waiting to take off.
Airport Class:
package edu.metrostate.ics240.p4.sim;
import java.io.FileNotFoundException; import java.io.PrintWriter; import java.time.LocalTime; import java.util.Random;
import edu.metrostate.ics240.p4.sim.Event.EventType;
public abstract class Airport {
private static final double DEPARTURE_PROB = 0.20; private static final double ARRIVAL_PROB = 0.15; private static final int SIM_MINUTES = 60; private static final long SEED = 20170216001L; private static final Random RAND = new Random(SEED); protected static final String DELIM = "\\|";
/** * Generates a file of flight events based on default settings * @param fileName name of output file */ public static void genEventFile(String fileName) { genEventFile(fileName, ARRIVAL_PROB, DEPARTURE_PROB, SIM_MINUTES); }
/** * Generates a file of flight events based on provided parameters * @param fileName name of output file * @param arrivalProbability statistical probability of an arrival at a given time * @param departureProbability statistical probability of a departure at a given time * @param minutes number of minutes of simulator data to generate */ public static void genEventFile(String fileName, double arrivalProbability, double departureProbability, int minutes) { int flightId = 1; try (PrintWriter simFile = new PrintWriter(fileName);) { LocalTime time = LocalTime.parse("00:00");
for (int i = 0; i < minutes; i++) { if (RAND.nextDouble() <= arrivalProbability) { simFile.printf("%s|%s|%s ", time.plusMinutes(i), Event.EventType.ARRIVAL, String.format("A%03d", flightId++)); } if (RAND.nextDouble() <= departureProbability) { simFile.printf("%s|%s|%s ", time.plusMinutes(i), Event.EventType.DEPARTURE, String.format("D%03d", flightId++)); } } } catch (FileNotFoundException e) { e.printStackTrace(); } }
/** * Reads and processes the specified flight event file. * @param filename path to the flight event file. */ public abstract void processEventFile(String filename);
/** * Returns an array of all flights processed * * @return array of all flights processed */ public abstract Event[] getFlightsHandled();
}
Event Class:
import java.time.LocalTime;
public interface Event { public enum EventType { /** * Runway is available */ RUNWAY_AVAIL, /** * Runway is assigned to a flight */ RUNWAY_ASSIGN, /** * Arrival Flight */ ARRIVAL, /** * Departure Flight */ DEPARTURE, } /** * Returns the actual time of the arrival or departure. Note: the for RUNWAY_AVAL
event the * scheduled time is the actual time * @return the actualTime */ LocalTime getActualTime();
/** * Returns the event type for this flight event * @return the event type for this flight event */ EventType getEvent();
/** * Returns the identifier for this flight event * @return the ident the flight or runway identifier for this event */ String getIdent();
/** * Returns the schedule time for this event * @return the scheduledTime the scheduled time for this event */ LocalTime getScheduledTime();
}
Assignment:
Create a AirportSimulator class that extends the provided Airport abstract class
Feature | Signature | Requirement |
Constructor | AirportSimulator (int numRunways) | Precondition: numRunways >= 1; Postcondition: airport simulator with specified number of runways and default arrival and departure runway reserve times; |
| AirportSimulator(int numRunways, int arrivalReserveTime, int departureReserveTime) | Precondition: numRunways >= 1, arrivalReserveTime >= 1, departureReserveTime >= 1; Postcondition: airport simulator with specified number of runways and arrival and departure runway reserve times (in minutes); |
Methods | public void processEventFile(String filename) | Precondition: filename contains path to the events file; events file is pipe-delimited file containing the scheduled time, event type (ARRIVAL or DEPARTURE) and a Flight ID. |
| public Event[] getFlightsHandled(); | Returns an array of all flights processed |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
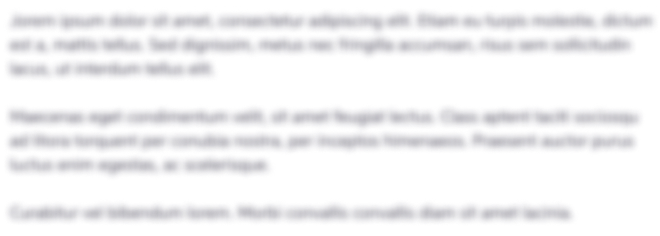
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started