Question
You may have noticed that the Smart Home application we built in the last assignment was a little clumsy to implement. If we keep adding
You may have noticed that the Smart Home application we built in the last assignment was a little
clumsy to implement. If we keep adding new devices, variables will be harder to track, and settings will
be harder to manage. You may also notice that certain parts of the Smart Home could be grouped into
different categories (for example, both the Music and Television devices shared audio settings). We
could make our program more efficient and capable of growth by using inheritance. Object-oriented
design to the rescue!
Here are your program requirements for your revised Smart Home:
• Utilize a parent class called "Device." This should have methods and variables to access and
change the power status and the room they are located in
• Create Child Classes called "Entertainment," "Appliance," "Security," and "Light." These should
all inherit from "Device"
• Create Child Classes that inherit from "Entertainment" that include "Music" and "Television"
• Create Child Classes that inherit from "Appliance" that include "HVAC," "Oven," and
"Refrigerator"
• Create Child Classes that inherit from "Security" that include "MotionSensor" and "Camera"
• Each class should have constructors and member variables. Keep variables private; only allow
methods to access them
• Populate each class with relevant functionality (you can use Assignment 1 for some insight). Be
sure to locate methods in the appropriate hierarchy. If functionality is common across all
"Entertainment" devices (such as "changeVolume()"), then put that in the "Entertainment"
class. If something is unique to "Television," then put it in that class
• Instantiate at least one instance of the following in your main program: Light, Music, Television,
HVAC, Oven, Refrigerator, MotionSensor, and Camera. This should be in a new file called
"SmartHome2.java"
• As with the previous assignment, allow users the ability to input specific commands. You should
have at least 12 distinct commands of your choosing; however, one of them should be "Help."
When the user types "Help," have the system print out all commands for reference. Each
command (except for "Help") should control one or more Smart Home devices.
Include appropriate comments throughout your code. Remember to use standard Java naming
conventions. Put your name and information about the program at the beginning of your program.
Submit your SmartHome2.java, all other Class and Java files in your project, and a screenshot of it in
operation on your computer. Package all of these in a zip file and name the submission in the format:
NAME_COURSE_ASSIGNMENT_DATE.zip
MY CURRENT CODE:
import java.util.*;
import java.io.*;
public class SubmittedSmartHome
{
public static void main(String args[]) throws IOException
{
double Ftemperature=70; // Declares FTemperature double variable and set's it to 70 degrees fahrenheit
String str; // Declares String named str
FileReader filer; // Declares a file Reader named filer
BufferedReader bufferr; // Declares a buffer reader named bufferr
boolean matchfound; // declares a boolean named matchfound for Channel and Song choices
int ch; //Declares a integer variable named ch
Scanner sc=new Scanner(System.in);
do
{
System.out.println ("Welcome Home, which of the following commands would you like to execute?"); // Welcomes home the user and asks which command they would like to execute
System.out.println("1:Change Temperature\n2:Play Music\n3:Stop Music\n4:Turn on Television\n5:Turn off Television\n6:Turn on Light\n7:Turn off Light\n8:Make a call\n9:Answer Doorbell\n10:Close System\nEnter your choice:"); // Lists all commands available each on it's own line
ch=sc.nextInt(); // Allows for the user to input which command they would like to execute
sc.nextLine();
switch(ch)
{
case 1: // Sets up case 1 which is changing the temperature
System.out.println("Current Room Temperature in fahrenheit="+Ftemperature); // States the current temperature that the house is set at
System.out.println("What Temperature in fahrenheit you would like?"); // Asks what temperature the user would like to set the house to
double temp=sc.nextDouble(); // Allows the user to input the temperature they would like to set the house to on a new line.
if(temp>Ftemperature) // Opens if/else for setting the AC to heat or cool
{
System.out.println("Air Conditioning is now set to heat"); // If the A/C is set to a higher temperature, out puts a message stating that the heat is on
}
else
{
System.out.println("Air Conditioning is now set to cool"); // If the A/C is set to a lower temperature, outputs a message stating that the cooling is now on.
}
Ftemperature=temp;
System.out.println("The temperature is set to" +Ftemperature +" in fahrenheit"); // outputs the temperature that the user input as the currently set temperature
break;
case 2:
System.out.println("What song would you like to listen to?"); // Asks the user for which song they would like to listen to
String song=sc.nextLine(); // Allows the user to input which song they would like to listen to on a new line
filer=new FileReader("songs.txt"); // Reads the file "songs.txt" for the list of songs that can be played
bufferr=new BufferedReader(filer); //
matchfound=false;
while(( str=bufferr.readLine())!=null)
{
if(str.equalsIgnoreCase(song))
{
matchfound=true;
break;
}
}
bufferr.close(); // closes the buffer reader
filer.close(); // closes the file reader
if(matchfound)// if a match is found on the song.txt file, it will output the following message on the screen.
{
System.out.println("Now playing the requested song"); // Will output this message on the screen if a match for the song is found.
}
else // opens else
{
System.out.println("The song you requested is unavailable, please use the list below for songs available."); // If a song is not on songs.txt it will output this message onto the screen
filer=new FileReader("songs.txt"); // lists the songs listed in songs.txt when user inputs a song not listed
bufferr=new BufferedReader(filer);
while(( str=bufferr.readLine())!=null)
{
System.out.println(str);
}
bufferr.close(); // closes buffer reader
filer.close(); // closes file reader
}
break;
case 3:
System.out.println("Stopping music currently playing"); // Prints out message that the music currently playing is being stopped
break;
case 4:
System.out.println("Which Channel you would like?"); // Asks the user which channel they would like on the TV when it turns on
String channel=sc.nextLine(); // Allows for the user to input a channel number
filer=new FileReader("channels.txt"); // Reads the file "channel.txt" for the list of channels that are available
bufferr=new BufferedReader(filer);
matchfound=false;
while((str=bufferr.readLine())!=null)
{
if(str.equalsIgnoreCase(channel))
{
matchfound=true;
break;
}
}
bufferr.close();
filer.close();
if(matchfound) // if a match is found on the channnel.txt file, it will output the following message on the screen.
{
System.out.println("Turning on TV to requested channel"); // Will output this message on the screen if a match for the channel is found on the list.
}
else
{
System.out.println("The channel you requested is unavailable. Please see below list for available channels.");
filer=new FileReader("channels.txt"); // lists the channels listed in channels.txt when user inputs a channel number that is not listed
bufferr=new BufferedReader(filer);
while(( str=bufferr.readLine())!=null)
{
System.out.println(str);
}
bufferr.close();
filer.close();
}
break;
case 5:
System.out.println("Turning off the TV"); // Prints a message on the screen confirming that the TV has been turned off in
break;
case 6:
System.out.println("Which room would you like to turn on the light for?"); // Asks the user which room they would like to turn the lights on in.
System.out.println("Bedroom, Kitchen, Living room, Bathroom, or Garage"); // Lists the options for rooms where the lights can be turned on in.
String roomnamed=sc.nextLine(); // Allows the user to input which room they would like the lights to be turned on in
System.out.println("The lights are now on in the "+roomnamed); // Confirms that the lights have been turned on in the room that the user has input
break;
case 7:
System.out.println("The lights have been turned off"); // Prints out a line stating that the lights have been turned off
break;
case 8:
System.out.println("Stopping all currently playing audio"); // Prints out message that all currently playing audio is being stopped
System.out.println("What phone number would you like to call?"); // Asks what phone number the user would like to call
String no=sc.nextLine(); // allows for user to input the 10 digit phone number
if(no.length()!=10) // Sets the length required for phone numbers to be 10 digits long.
{
System.out.println("Please try again, the phone number you entered wasn't formatted correctly");// Prints an error message if the phone number entered is not 10 digits
String no1=sc.nextLine(); // allows for user to input the 10 digit phone number
}
break;
case 9:
System.out.println("Stopping all currently playing audio"); // Prints out message that all currently playing audio is being stopped
System.out.println("Please enter the message you would like to send to the person at the door:"); // Asks what message the user would like to send to whomever is at the door
String message=sc.nextLine(); // Allows for the user to input the message they would like to send
System.out.println("The message: ' " + message +" ' has been sent"); // Prints out a confirmation of the message and that it has been sent.
break;
case 10:
System.out.println("Shutting down all systems"); // Prints out that the system is shutting down
sc.close();
break;
default:
System.out.println("Invalid Command"); // Prints out "Invlid command" if the command entered is not one of the options
}
}while(ch!=10);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
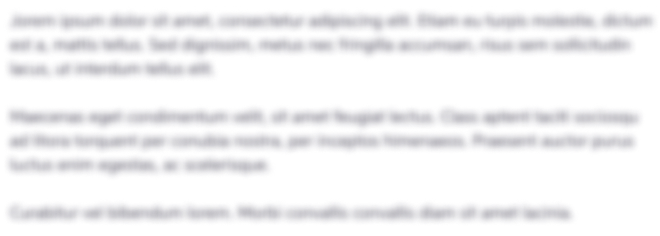
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started