Question
You might recognize the code on the next page as being the wds.c program from the text and spell checker fame. Modify this program to
You might recognize the code on the next page as being the wds.c program from the text and spell checker fame. Modify this program to print each word on a separate line with the phrase has double letters after any word that has the same letter twice adjacent to each other in the word.
For example, the input:
would output:
Ever really looked at the moon, Lloyd?
Ever
really has double letters
looked has double letters
at
the
moon has double letters
Lloyd has double letters
/* File: tfdef.h */ #define TRUE 1 #define FALSE 0
/* File: chrutil.c */ /* This file contains various utility functions for processing characters */ #include#include "tfdef.h" #include "chrutil.h" /* Function converts ch to an integer if it is a digit. Otherwise, it prints an error message. */ int dig_to_int(char ch) { if (IS_DIGIT(ch)) return ch - '0'; printf("ERROR:dig_to_int: %c is not a digit ", ch); return ERROR; } /* Function converts a positive integer less than 10 to a corresponding digit character. */ char int_to_dig(int n) { if (n >= 0 && n < 10) return n + '0'; printf("ERROR:int_to_dig: %d is not in the range 0 to 9 ", n); return NULL; } /* Function reads the next integer from the input */ int getint() { int n = 0; int got_dig = FALSE; signed char ch; ch = getchar(); /* read next char */ while (IS_WHITE_SPACE(ch)) /* skip white space */ ch = getchar(); while (IS_DIGIT(ch)) { /* repeat as long as ch is a digit */ n = n * 10 + dig_to_int(ch); /* accumulate value in n */ got_dig = TRUE; #ifdef DEBUG printf("debug:getint: ch = %c ", ch); /* debug statement */ printf("debug:getint: n = %d ", n); /* debug statement */ #endif ch = getchar(); /* read next char */ } if(ch == EOF) return EOF; /* test for end of file */ if(!got_dig) return ERROR; /* test for no digits read */ return n; /* otherwise return the result */ } /* Function tests if c is an alphabetic letter. */ int letterp(char c) { if (IS_LOWER(c) || IS_UPPER(c)) return TRUE; return FALSE; } /* Function returns TRUE if c is a delimiter, i.e., it is a white space or a punctuation. Otherwise, it returns FALSE. */ int delimitp(char c) { if (whitep(c) || punctp(c)) return TRUE; return FALSE; } /* Function returns TRUE if c is white space; returns FALSE otherwise. */ int whitep(char c) { if (c == ' ' || c == '\t' || c == ' ') return TRUE; return FALSE; } /* Function returns TRUE if c is a punctuation; returns FALSE otherwise. */ int punctp(char c) { if (c == '.' || c == ',' || c == ';' || c == ':' || c == '?' || c == '!') return TRUE; return FALSE; } /* Function checks if c is a vowel. */ int vowelp(char c) { switch(c) { case 'a': case 'A': case 'e': case 'E': case 'i': case 'I': case 'o': case 'O': case 'u': case 'U': return TRUE; default: return FALSE; } } /* Function tests if c is printable. */ int illegal(char c) { if (IS_PRINT(c) || IS_WHITE_SPACE(c)) return FALSE; return TRUE; }
#include
#include "tfdef.h"
#include "chrutil.h"
main()
{ signed char ch;
int inword,
lns, wds, chrs;
/* flag indicating when in a word */
/* Counters for lines, words, chars. */
} }
lns = wds = chrs = 0; /* initialize counters to 0 */
inword = FALSE; /* before beginning we are not in a word */
while ((ch = getchar()) != EOF) /* while there are more chars */
{ chrs = chrs + 1;
if (ch == )
/* count characters */
/* if newline char */
/* count lines */
lns = lns + 1;
/* if not in a word and not a delimiter */
/* then this must be the beginning of a word */
if (!inword && !delimitp(ch))/* if not in word and not delim*/
{ inword = TRUE; /* remember we are in a word */
wds = wds + 1; /* count words */
}
/* otherwise if in a word, but found a delimiter */
/* then we just went beyond the end of the word */
else if (inword && delimitp(ch))/* if in word and a delim*/
{ inword = FALSE;
putchar( );
}
if (inword)
putchar(ch);
/* no longer in word */
/* end word with newline */
/* if in a word */
/* print the character */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
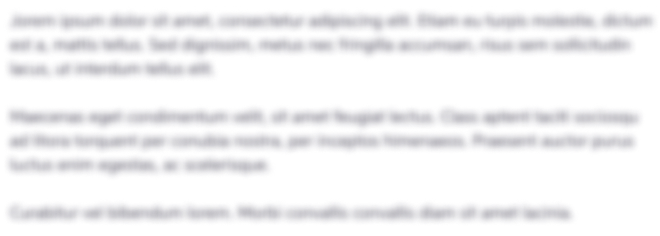
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started