Question
You must select, copy and paste the output created by your program You must upload your source code file to the Assignment submission online Make
You must select, copy and paste the output created by your program
You must upload your source code file to the Assignment submission online
Make sure your program has comments
You must follow the coding conventions and indent your code before submitting otherwise you automatically lose 2 points.
Do not use Arrays
Objectives:
1. Write a subroutine that takes a parameter
2. Write a subroutine that returns a value
3. Modify your solution to previous assignment
Problem
Modify your spellNumber() subroutine from Assignment 6 to make it return a String containing the number spelled out. Your new version of spellNumber() will do NO WRITING TO THE CONSOLE. This subroutine will still have a parameter that tells it what number to spell out. Here is your test program:
class TestSpellNumber {
public static void main(String args []) {
for(int i=-9;i<=9;i++){
System.out.println(UsefulRoutines7.spellDigit(i));
}
System.out.println("------------------");
for(int i=-99;i<=99;i++){
System.out.println(UsefulRoutines7.spellNumber(i));
}
}
}
First get the first three lines on the main() above to work. That entails modifying the method spellDigit() to make it return the String instead of printing it. Then modify the subroutine spellNumber() to make it return the String instead of printing it.
Notes:
This program will have no user input.
The subroutines spellDigit() and spellNumber() will contain no calls to System.out.println()
The subroutine spellNumber() will work for any integer that is between -99 and 99.
You must submit both source code files (TestSpellNumber.java and UsefulRoutines.java)
______________________________________________________________________________________________________________________________________________
Here is my program from my assignment 6 :
=========================================================================================================================
public class UsefulRoutines6 {
/** * This method spells out a single-digit number, given in the parameter. It * doesn't return anything or get any user input, but there is output. */
public static void spellDigit(int number) { if (number < -9 || number > 9) { System.out.println("Error"); } else { if (number == 0) { System.out.println("Zero"); } else { String output = ""; if (number < 0) { output = "negative "; number = number * -1;// convert to positive so we have fewer cases } switch (number) { case 1: System.out.println(output + "one"); break; case 2: System.out.println(output + "two"); break;
// ...list the rest } }
} }
/** * This method spells out a two-digit number, given in the parameter. It * doesn't return anything or get any user input, but there is output. This * method calls spellDigit for numbers smaller than 10 and for the ones * place of numbers larger than 20, unless that digit is a 0. */ public static void spellNumber(int number) { if (number < -99 || number > 99) { System.out.println("Error"); } else { String output = ""; if (number < 0) { output = "negative "; number = number * -1; } System.out.print(output); if(number<10){ spellDigit(number); } else if(number < 20){ switch (number){ case 10: System.out.println("ten"); break; case 11: System.out.println("eleven"); break; // list the rest thru 19 } } else{ //numbers between 20 and 99 int tens = number/10; int unit = number%10; //System.out.println("tens="+tens+" units="+unit); switch(tens){ case 2: System.out.print("twenty "); break; case 3: System.out.print("thirty "); break; //list rest thru 9 = ninety } if(unit!=0){ //we do not want to print zero spellDigit(unit); } else{ //goto next line System.out.println(); } } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
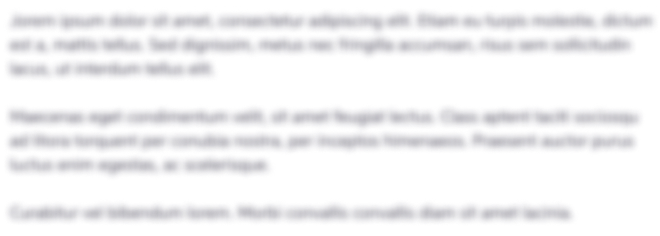
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started