Question
YOU MUST USE THE SKELETON CODE IN ANSWER. IT IS PROVIDED BELOW. This is the first assignment in a two-part series. The overall goal is
YOU MUST USE THE SKELETON CODE IN ANSWER. IT IS PROVIDED BELOW.
This is the first assignment in a two-part series. The overall goal is to create a set of classes that are capable of managing and displaying dates by the Gregorian and Julian calendars. The purpose of these two assignments is to have you learn about creating classes, encapsulation, abstraction, and polymorphism. The first in the series takes a naive view of creating classes, resulting in a program that has a lot of duplicated code. Then, in the second part, you'll clean up all of the duplication through the use of the Java programming language features, making a big improvement of the first assignment in the series.
Many calendars have been in use throughout human history, depending upon the culture and time. Two that have most impacted western civilization are the Julian and Gregorian calendars. The Julian calendar was in use for many centuries, but has a problem in that it "gains" about three days every four centuries. The Gregorian calendar, more or less, solves this problem by changing how it computes leap years to better account for the time it takes the Earth to complete a full orbit.
Both calendars have the same months and number of days in each month, including 29 days in February on leap years. As noted above, the Gregorian calendar has a different rule for computing leap years. Additionally, the first valid date for the Gregorian calendar is October 15, 1582.
For the purposes of this assignment, we are mostly interested in the difference in how leap years are determined, and using that, want to represent dates using both calendars. For example, today (when I'm writing this) on the Gregorian calendar is October 4th, 2018, but on the Julian calendar is September 21st, 2018.
Details
The following details the requirements for the assignment:
- Create two classes, GregorianDate and JulianDate.
- The two date classes must expose only the following public instance methods and constructors, and provides an implementation that is appropriate for the calendar:
- Default constructor that sets the date to the current date. See notes below about dates to help you figure out how to do this appropriately for each calendar.
- Overloaded constructor that takes year, month, and date values and sets the date to that date: GregorianDate(int year, int month, int day) and JulianDate(int year, int month, int day)
- A method that adds a specified number of days to the date: void addDays(int days)
- Remember to track when the month and years change.
- A method that subtracts a specified number of days to the date: void subtractDays(int days)
- Remember to track when the month and years change.
- A method that returns true/false if the date is part of a leap year: boolean isLeapYear()
- A method that prints the date (without a carriage return) in mm/dd/yyyy format: void printShortDate()
- A method that prints the date (without a carriage return) in Monthname dd, yyyy format: void printLongDate()
- The following 'get' accessor methods. These aren't used by the sample driver code, but would normally be part of a class like this. These accessor methods are used by the unit tests. Note the word "current" doesn't mean "today", it means whatever the value is represented by the date, it is the "current value" of the date.
- String getCurrentMonthName()
- int getCurrentMonth()
- int getCurrentYear()
- int getCurrentDayOfMonth()
- Store the year, month, and day values as private instance (non-static) data members of the class.
- The two date classes may provide only the following private methods, which you'll use in support of the other public instance methods:
- A method that returns true/false if a a specified year is a leap year: boolean isLeapYear(int year)
- A method that returns the number of days in a month (for a year): int getNumberOfDaysInMonth(int year, int month)
- A method that returns the number of days in a year: int getNumberOfDaysInYear(int year)
- A method that returns the name of a month; first letter capitalized, remainder lowercase: String getMonthName(int month)
The provided skeleton project contains code in the Assign5.java file that exercises all of the features of both classes. You'll want to first comment this code and slowly uncomment as you build up your classes.
In thinking about how to write the code for this assignment, follow the concepts you learned from Stepwise Refinement and bottom up implementation. The Stepwise Refinement has been done for you, that is the various methods you are required to write are detailed above. Your responsibility is the bottom up implementation. Stub in the required methods, returning dummy values until you are able to write the correct implementation. Start with the base methods, such as the boolean isLeapYear(int year) method and build up from there. Write small tests in the main method to test each method as you go. Don't move on to another method until you have the one you are currently working on correctly implemented. In other words, build up your code slowly, one method at a time and validating as you go, rather than trying to write all of the methods and then debugging afterwards.
Helpful Information About Dates
- Leap years (https://en.wikipedia.org/wiki/Leap_year (Links to an external site.)Links to an external site.):
- Julian calendar: If the remainder of the year when divided by 4 is 0
- Gregorian calendar (from wiki link above): "Every year that is exactly divisible by four is a leap year, except for years that are exactly divisible by 100, but these centurial years are leap years if they are exactly divisible by 400".
- Use System.currentTimeMillis() to obtain the number of milliseconds that have occurred since January 1, 1970.
- The number returned from this method does not account for the time zone of the computer it is running on, you need to account for that. To get the time zone offset to correct for local time you can call java.util.TimeZone.getDefault().getRawOffset() This gives the number of milliseconds to "add" to the current time. I say "add" because it is a negative number, it is the offset you need.
- For a Gregorian date, you can use this knowledge to start by initializing a date to 1/1/1970, then adding the number of days the number of milliseconds since then have taken place; remember to account for the time zone as described above.
- For a Julian date, you have to set the starting date to January 1, 1 (1/1/1), then add in the number of days until (Gregorian) January 1, 1970, then, update the date with the number of milliseconds since 1/1/1970; of course, remember to account for the time zone as described above. The number of Julian days from 1/1/1 to 1/1/1970 is 719164. Here is a handy reference to compute the number of days between two dates: http://www.csgnetwork.com/juliancountdaysfromtocalc.html (Links to an external site.)Links to an external site.
The following are some links that provide additional information about the two calendars. You don't need any of these, just providing them for information purposes, if you are interested in learning more about the calendars.
- Julian calendar wiki: link (Links to an external site.)Links to an external site.
- Gregorian calendar wiki: link (Links to an external site.)Links to an external site.
- Converting between Julian and Gregorian calendar dates: link (Links to an external site.)Links to an external site.
- Online Julian date converter: link (Links to an external site.)Links to an external site.
- Leap year wiki: link (Links to an external site.)Links to an external site.
The provided driver code will produce output like the following, depending, of course, on which day you run your code:
--- Demonstrating Julian Dates --- Today's date is : February 7, 2019 Tomorrow will be : February 8, 2019 Yesterday was : February 6, 2019 This year is not a leap year. Demonstrating: 2/27/2100 Adding 1 day to the date : 2/28/2100 Adding another day : 2/29/2100 Just one more day : 3/1/2100 Going backwards by 2 days : 2/28/2100 Demonstrating: 2/27/2000 Adding 1 day to the date : 2/28/2000 Adding another day : 2/29/2000 Just one more day : 3/1/2000 Going backwards by 2 days : 2/28/2000 Demonstrating: 2/27/2001 Adding 1 day to the date : 2/28/2001 Adding another day : 3/1/2001 Just one more day : 3/2/2001 Going backwards by 2 days : 2/28/2001 Demonstrating: 12/30/1999 Adding 1 day to the date : 12/31/1999 Adding another day : 1/1/2000 Just one more day : 1/2/2000 Going backwards by 2 days : 12/31/1999 --- Demonstrating Gregorian Dates --- Today's date is : February 20, 2019 Tomorrow will be : February 21, 2019 Yesterday was : February 19, 2019 This year is not a leap year. Demonstrating: 2/27/2100 Adding 1 day to the date : 2/28/2100 Adding another day : 3/1/2100 Just one more day : 3/2/2100 Going backwards by 2 days : 2/28/2100 Demonstrating: 2/27/2000 Adding 1 day to the date : 2/28/2000 Adding another day : 2/29/2000 Just one more day : 3/1/2000 Going backwards by 2 days : 2/28/2000 Demonstrating: 2/27/2001 Adding 1 day to the date : 2/28/2001 Adding another day : 3/1/2001 Just one more day : 3/2/2001 Going backwards by 2 days : 2/28/2001 Demonstrating: 12/30/1999 Adding 1 day to the date : 12/31/1999 Adding another day : 1/1/2000 Just one more day : 1/2/2000 Going backwards by 2 days : 12/31/1999
Unit Tests
You are provided unit tests that help you know if your code is correct. These tests cover key elements of your code, but aren't exhaustive. However, if you pass all of them, your code is probably correct.
// Provided skeleton below// CLASS ASSIGN5
public class Assign5 { public static void main(String[] args) { System.out.printf("--- Demonstrating Julian Dates --- "); demoJulianFromToday(); JulianDate futureYear = new JulianDate(2100, 2, 27); demoDateJulian2(futureYear); JulianDate leapYear = new JulianDate(2000, 2, 27); demoDateJulian2(leapYear); JulianDate notLeapYear = new JulianDate(2001, 2, 27); demoDateJulian2(notLeapYear); JulianDate endOfYear = new JulianDate(1999, 12, 30); demoDateJulian2(endOfYear); System.out.printf(" --- Demonstrating Gregorian Dates --- "); demoGregorianFromToday(); GregorianDate futureYearG = new GregorianDate(2100, 2, 27); demoDateGregorian2(futureYearG); GregorianDate leapYearG = new GregorianDate(2000, 2, 27); demoDateGregorian2(leapYearG); GregorianDate notLeapYearG = new GregorianDate(2001, 2, 27); demoDateGregorian2(notLeapYearG); GregorianDate endOfYearG = new GregorianDate(1999, 12, 30); demoDateGregorian2(endOfYearG); }
public static void demoJulianFromToday() { JulianDate date = new JulianDate(); System.out.print("Today's date is : "); date.printLongDate(); System.out.println(); date.addDays(1); System.out.print("Tomorrow will be : "); date.printLongDate(); System.out.println(); date.subtractDays(2); System.out.print("Yesterday was : "); date.printLongDate(); System.out.println(); if (date.isLeapYear()) { System.out.println("This year is a leap year!"); } else { System.out.println("This year is not a leap year."); } }
public static void demoGregorianFromToday() { GregorianDate date = new GregorianDate(); System.out.print("Today's date is : "); date.printLongDate(); System.out.println(); date.addDays(1); System.out.print("Tomorrow will be : "); date.printLongDate(); System.out.println(); date.subtractDays(2); System.out.print("Yesterday was : "); date.printLongDate(); System.out.println(); if (date.isLeapYear()) { System.out.println("This year is a leap year!"); } else { System.out.println("This year is not a leap year."); } }
public static void demoDateJulian2(JulianDate date) { System.out.println(); System.out.print("Demonstrating: "); date.printShortDate(); System.out.println(); System.out.print("Adding 1 day to the date : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Adding another day : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Just one more day : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Going backwards by 2 days : "); date.subtractDays(2); date.printShortDate(); System.out.println();
public static void demoDateGregorian2(GregorianDate date) { System.out.println(); System.out.print("Demonstrating: "); date.printShortDate(); System.out.println(); System.out.print("Adding 1 day to the date : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Adding another day : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Just one more day : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Going backwards by 2 days : "); date.subtractDays(2); date.printShortDate(); System.out.println(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
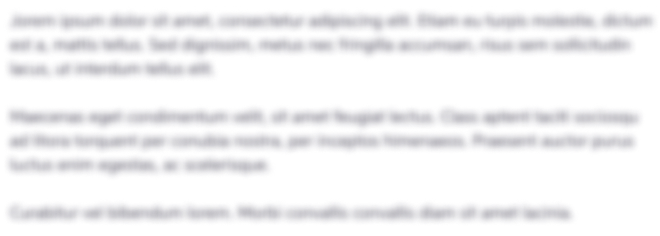
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started