Question
You will be working with three.java files in this lab. Pokemon.java - Class definition (completed) Player.java - Class definition PokemonField.java - Contains main() method Complete
You will be working with three.java files in this lab.
- Pokemon.java - Class definition (completed)
- Player.java - Class definition
- PokemonField.java - Contains main() method
-
Complete the Player class with the following specifications:
(1) add the following three private fields
-
String name
-
int level
-
ArrayList
pokemons (2) add a constructor, which takes one parameter to initialize the name field. The level is initialized as 0. The constructor also creates the ArrayList.
(3) add each of the following public member methods
- getName()
- getLevel()
- getPokemons()
- setLevel(int level)
- addPokemon(Pokemon p)
- removePokemon(Pokemon p)
- printStat()import java.util.Random;
-
import java.util.Scanner;
public class PokemonField { public static void main(String[] args) { Player player1 = new Player("dragonslayer"); Player player2 = new Player("voldemort"); Random random = new Random(); initialize(player1, player2); player1.printStat(); player2.printStat(); play(random, player1, player2); System.out.println("Game over"); player1.printStat(); player2.printStat(); } public static boolean fight(Random random, Pokemon p1, Pokemon p2){ double prob = (double)p1.getAttackPoints() / (p1.getAttackPoints() + p2.getAttackPoints()); while(p1.getHitPoints() > 0 && p2.getHitPoints() > 0) { if(random.nextDouble() < prob) { p2.setHitPoints(Math.max(p2.getHitPoints()- p1.getAttackPoints(), 0)); } else{ p1.setHitPoints(Math.max(p1.getHitPoints() - p2.getAttackPoints(), 0)); } } if(p1.getHitPoints() > 0){ System.out.println(p1.getName() + " won!"); return true; } else { System.out.println(p2.getName() + " won!"); return false; } } public static void initialize(Player player1, Player player2) { String[] pokemonNames1 = {"Butterfree", "Ivysaur", "Pikachu", "Squirtle"}; int[] pokemonMaxHps1 = {10, 15, 20, 30}; int[] pokemonAps1 = {1, 2, 2, 3}; String[] pokemonNames2= {"Jigglypuff","Oddish","Gloom","Psyduck","Machop"}; int[] pokemonMaxHps2 = {12, 18, 25, 27, 32}; int[] pokemonAps2 = {1, 1, 2, 2, 3}; for(int i = 0; i < pokemonNames1.length; i++) { /*FIX(1) create a Pokemon object with the following three arguments:pokemonNames1[i], pokemonMaxHps1[i], pokemonAps1[i] Add the Pokemon object to the Pokemon array list in player1 Increment player1's level by 1*/ } for(int i = 0; i < pokemonNames2.length; i++) { /*FIX(2) create a Pokemon object with the following three arguments:pokemonNames2[i], pokemonMaxHps2[i], pokemonAps2[i] Add the Pokemon object to the Pokemon array list in player2 Increment player2's level by 1*/ } } public static void play(Random random, Player player1, Player player2) { Pokemon p1, p2; int index1, index2; Scanner scnr = new Scanner(System.in); boolean result; System.out.println("Let's play!"); System.out.println(player1.getName() + " choose a pokemon to fight enter 1 to " + player1.getPokemons().size() + " or -1 to stop"); index1 = scnr.nextInt(); System.out.println(player2.getName() + " choose a pokemon to fight enter 1 to " + player2.getPokemons().size() + " or -1 to stop"); index2 = scnr.nextInt(); while(index1 != -1 && index2 != -1) { p1 = player1.getPokemons().get(index1-1); p2 = player2.getPokemons().get(index2-1); result = fight(random, p1, p2); if(result) { /*FIXME(3) p1 won, reset p2, remove p2 from player2, and add p2 to player 1, increment player1's level by 1*/ } else { /*FIXME(4) p2 won, reset p1, remove p1 from player1, and add p1 to player 2, increment player2's level by 1*/ } System.out.println(player1.getName() + " choose a pokemon to fight enter 1 to " + player1.getPokemons().size() + " or -1 to stop"); index1 = scnr.nextInt(); System.out.println(player2.getName() + " choose a pokemon to fight enter 1 to " + player2.getPokemons().size() + " or -1 to stop"); index2 = scnr.nextInt(); } } }
-
-
import java.util.ArrayList; public class Player { /*FIXME(1) Add three fields: name, level, pokemons*/ /*FIXME(2) Add a constructor, which takes one parameter: String n. Initialize name to n, level to 0, and create an ArrayList for pokemons*/ /*FIXME (3) Define the accessor for name*/ /*FIXME (4) Define the accessor for level*/ /*FIXME (5) Define the accessor for pokemons*/ /*FIXME (6) Define the mutator for level*/ /*FIXME(7) Complete this method by adding p into the Pokemon ArrayList*/ public void addPokemon(Pokemon p){ } /*FIXME(8) Complete this method by removing p from the Pokemon ArrayList*/ public void removePokemon(Pokemon p){ } public void printStat(){ System.out.println(" Player: " + name + " at level " + level + ", has " + pokemons.size() + " pokemons"); /*FIXME(9) Complete this method by printing the stat of each pokemon in the Pokemon ArrayList. Each pokemon's stat is printed in an individual line. Hint: You can call the printStat method in Pokemon class*/ } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
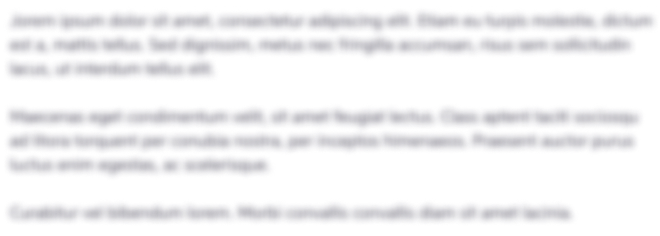
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started