Answered step by step
Verified Expert Solution
Question
1 Approved Answer
You will be writing a Classroom Organizer to help with seating students. In this assignment, you'll be using your knowledge of iteration and arrays
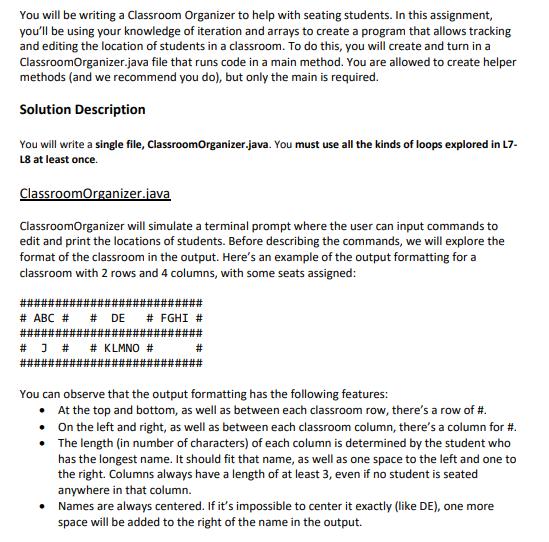
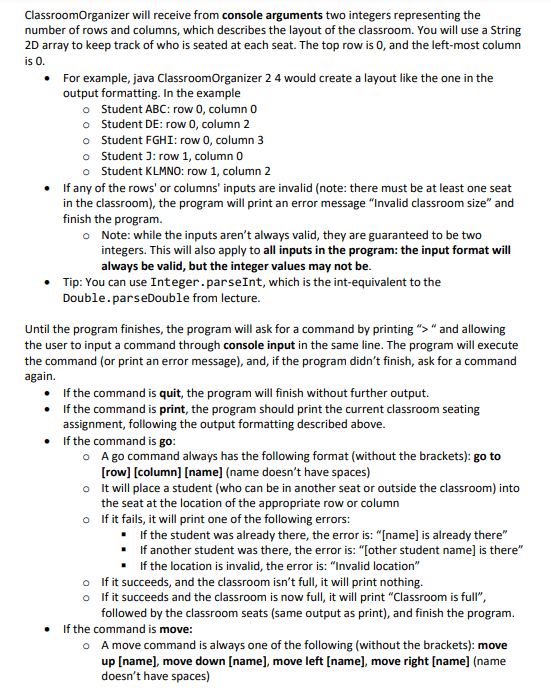
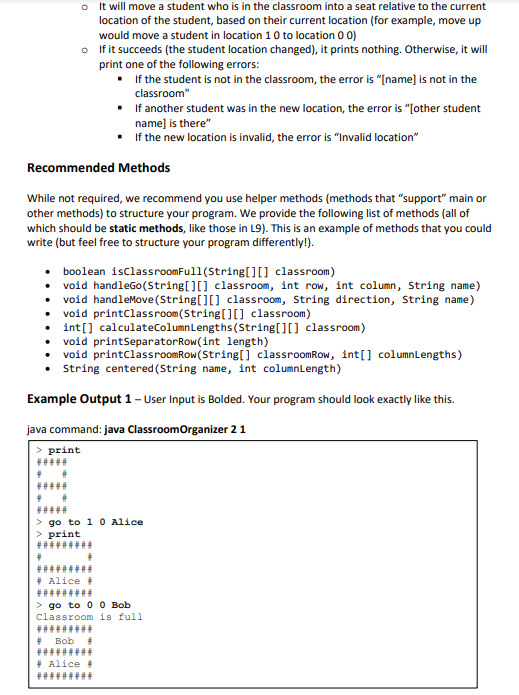
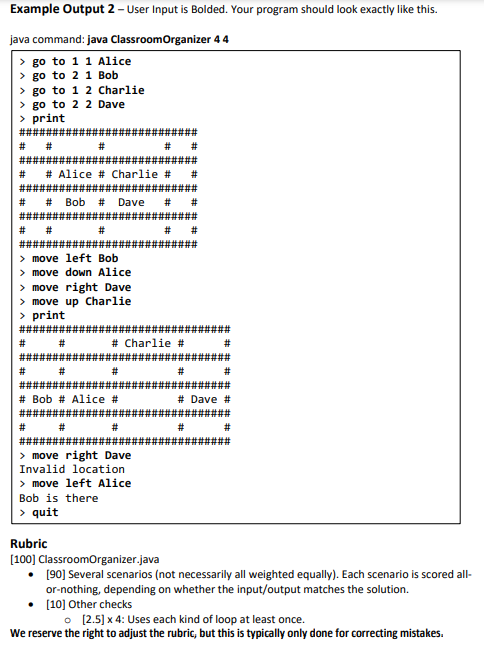
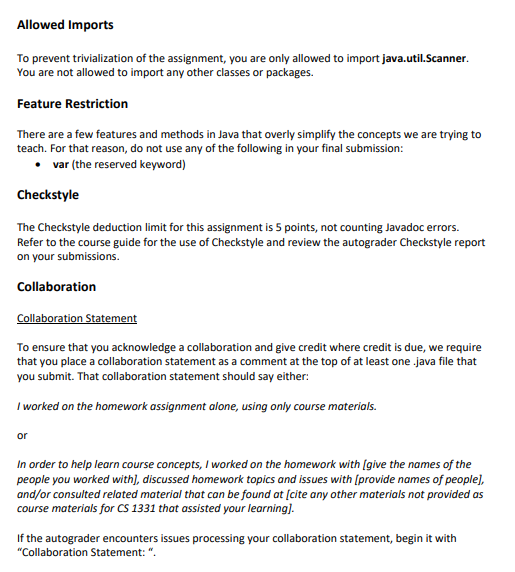
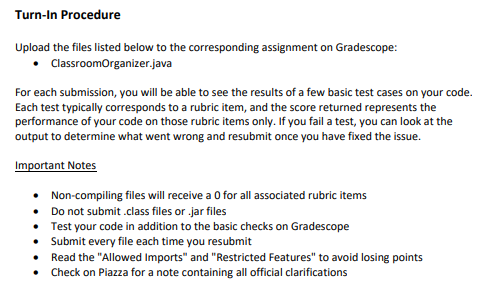
You will be writing a Classroom Organizer to help with seating students. In this assignment, you'll be using your knowledge of iteration and arrays to create a program that allows tracking and editing the location of students in a classroom. To do this, you will create and turn in a ClassroomOrganizer.java file that runs code in a main method. You are allowed to create helper methods (and we recommend you do), but only the main is required. Solution Description You will write a single file, ClassroomOrganizer.java. You must use all the kinds of loops explored in L7- L8 at least once. ClassroomOrganizer.java ClassroomOrganizer will simulate a terminal prompt where the user can input commands to edit and print the locations of students. Before describing the commands, we will explore the format of the classroom in the output. Here's an example of the output formatting for a classroom with 2 rows and 4 columns, with some seats assigned: ### # ABC # ##### # DE # FGHI # # J # # KLMNO # # You can observe that the output formatting has the following features: At the top and bottom, as well as between each classroom row, there's a row of #. On the left and right, as well as between each classroom column, there's a column for #. The length (in number of characters) of each column is determined by the student who has the longest name. It should fit that name, as well as one space to the left and one to the right. Columns always have a length of at least 3, even if no student is seated anywhere in that column. Names are always centered. If it's impossible to center it exactly (like DE), one more space will be added to the right of the name in the output. ClassroomOrganizer will receive from console arguments two integers representing the number of rows and columns, which describes the layout of the classroom. You will use a String 2D array to keep track of who is seated at each seat. The top row is 0, and the left-most column is 0. For example, java ClassroomOrganizer 2 4 would create a layout like the one in the output formatting. In the example o Student ABC: row 0, column 0 Student DE: row 0, column 2 o o Student FGHI: row 0, column 3 o Student J: row 1, column 0 o Student KLMNO: row 1, column 2 If any of the rows' or columns' inputs are invalid (note: there must be at least one seat in the classroom), the program will print an error message "Invalid classroom size" and finish the program. o Note: while the inputs aren't always valid, they are guaranteed to be two integers. This will also apply to all inputs in the program: the input format will always be valid, but the integer values may not be. Tip: You can use Integer.parseInt, which is the int-equivalent to the Double.parseDouble from lecture. Until the program finishes, the program will ask for a command by printing "> " and allowing the user to input a command through console input in the same line. The program will execute the command (or print an error message), and, if the program didn't finish, ask for a command again. If the command is quit, the program will finish without further output. If the command is print, the program should print the current classroom seating assignment, following the output formatting described above. If the command is go: o A go command always has the following format (without the brackets): go to [row] [column] [name] (name doesn't have spaces) o It will place a student (who can be in another seat or outside the classroom) into the seat at the location of the appropriate row or column o If it fails, it will print one of the following errors: If the student was already there, the error is: "[name] is already there" If another student was there, the error is: "[other student name] is there" If the location is invalid, the error is: "Invalid location" o If it succeeds, and the classroom isn't full, it will print nothing. o If it succeeds and the classroom is now full, it will print "Classroom is full", followed by the classroom seats (same output as print), and finish the program. If the command is move: o A move command is always one of the following (without the brackets): move up [name], move down [name], move left [name], move right [name] (name doesn't have spaces) Recommended Methods While not required, we recommend you use helper methods (methods that "support" main or other methods) to structure your program. We provide the following list of methods (all of which should be static methods, like those in L9). This is an example of methods that you could write (but feel free to structure your program differently!). o It will move a student who is in the classroom into a seat relative to the current location of the student, based on their current location (for example, move up would move a student in location 10 to location 0 0) o If it succeeds (the student location changed), it prints nothing. Otherwise, it will print one of the following errors: If the student is not in the classroom, the error is "[name] is not in the classroom" If another student was in the new location, the error is "[other student name] is there" If the new location is invalid, the error is "Invalid location" boolean isClassroom Full (String[][] classroom) void handleGo (String[][] classroom, int row, int column, String name) void handleMove (String[][] classroom, String direction, String name) void printClassroom (String[] [] classroom) int[] calculateColumn Lengths (String[][] classroom) void printSeparator Row(int length) void printClassroom Row (String[] classroomRow, int[] columnLengths) String centered (String name, int columnLength) Example Output 1 - User Input is Bolded. Your program should look exactly like this. java command: java ClassroomOrganizer 21 > print ***** # > go to 1 0 Alice > print ********* # # Alice # **** > go to 0 0 Bob classroom is full ********* # Bob # # Alice # *** Example Output 2 - User Input is Bolded. Your program should look exactly like this. java command: java ClassroomOrganizer 44 > go to 1 1 Alice > go to 2 1 Bob > go to 1 2 Charlie > go to 2 2 Dave > print ###* # # ########## # ###* # #### # # # Alice # Charlie # # # # Bob # Dave # # > move left Bob > move down Alice # > move right Dave > move up Charlie > print ##: # # # # # ## # Bob # Alice # #### # # # Charlie # # # > move right Dave Invalid location > move left Alice Bob is there > quit # # # # # # # # Dave # ### # Rubric [100] ClassroomOrganizer.java [90] Several scenarios (not necessarily all weighted equally). Each scenario is scored all- or-nothing, depending on whether the input/output matches the solution. . [10] Other checks o [2.5] x 4: Uses each kind of loop at least once. We reserve the right to adjust the rubric, but this is typically only done for correcting mistakes. Allowed Imports To prevent trivialization of the assignment, you are only allowed to import java.util.Scanner. You are not allowed to import any other classes or packages. Feature Restriction There are a few features and methods in Java that overly simplify the concepts we are trying to teach. For that reason, do not use any of the following in your final submission: var (the reserved keyword) Checkstyle The Checkstyle deduction limit for this assignment is 5 points, not counting Javadoc errors. Refer to the course guide for the use of Checkstyle and review the autograder Checkstyle report on your submissions. Collaboration Collaboration Statement To ensure that you acknowledge a collaboration and give credit where credit is due, we require that you place a collaboration statement as a comment at the top of at least one .java file that you submit. That collaboration statement should say either: I worked on the homework assignment alone, using only course materials. or In order to help learn course concepts, I worked on the homework with [give the names of the people you worked with], discussed homework topics and issues with [provide names of people], and/or consulted related material that can be found at [cite any other materials not provided as course materials for CS 1331 that assisted your learning]. If the autograder encounters issues processing your collaboration statement, begin it with "Collaboration Statement: ". Turn-In Procedure Upload the files listed below to the corresponding assignment on Gradescope: ClassroomOrganizer.java For each submission, you will be able to see the results of a few basic test cases on your code. Each test typically corresponds to a rubric item, and the score returned represents the performance of your code on those rubric items only. If you fail a test, you can look at the output to determine what went wrong and resubmit once you have fixed the issue. Important Notes Non-compiling files will receive a 0 for all associated rubric items Do not submit .class files or .jar files Test your code in addition to the basic checks on Gradescope Submit every file each time you resubmit Read the "Allowed Imports" and "Restricted Features" to avoid losing points Check on Piazza for a note containing all official clarifications
Step by Step Solution
There are 3 Steps involved in it
Step: 1
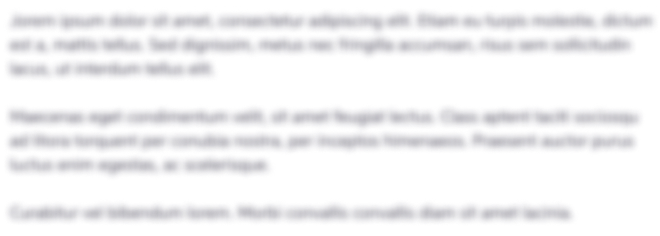
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started