Question
You will create a C++ program that can evaluate arithmetic operators with integer numbers having any number of digits. These numbers are an alternative to
You will create a C++ program that can evaluate arithmetic operators with integer numbers having any number of digits. These numbers are an alternative to xed size integers or oating point numbers that always have a maximum number of accurate digits (dependent on size of CPU register).
The input is a regular text le, where each line is terminated with an end-of-line character(s). Each line will containanarithmeticoperationbetweentwonumbers. Theprogramshoulddisplaytheinputexpressionand the results, separated with =.
The main program should be called innitearithmetic. infinitearithmetic "input=
The following is the pseudocode
// DoubleLinkedList.h struct Node { long long num; Node* prev; Node* next; };
class DoubleLinkedList { public: DoubleLinkedList(); // default construct ~DoubleLinkedList(); // deconstruct DoubleLinkedList(const std::string& num, int digitsPerNode); // user defined construct DoubleLinkedList(const DoubleLinkedList& list); // copy construct DoubleLinkedList& operator = (const DoubleLinkedList& list); // assignment consturct public: DoubleLinkedList operator + (const DoubleLinkedList& list) const; DoubleLinkedList operator * (const DoubleLinkedList& list) const; // optional DoubleLinkedList operator - (const DoubleLinkedList& list) const; // 10% extra DoubleLinkedList operator / (const DoubleLinkedList& list) const; // 20% extra DoubleLinkedList Sqrt(const DoubleLinkedList& list) const; public: const Node* GetHead() const; const Node* GetTail() const; void Append(Node* node); void Print() const; private: Node* head; Node* tail; int m_digitsPerNode; long long remainder; // for / operator float decimal; // for sqrt() 7 valid digits. }
// main.cpp #include "ArgumentManager.h" int main(int argc, char* argv[]) { if (argc < 2) { std::cerr("Usage: infinitearithmetic \"filename=xyz.txt;digitsPerNode=
Step by Step Solution
There are 3 Steps involved in it
Step: 1
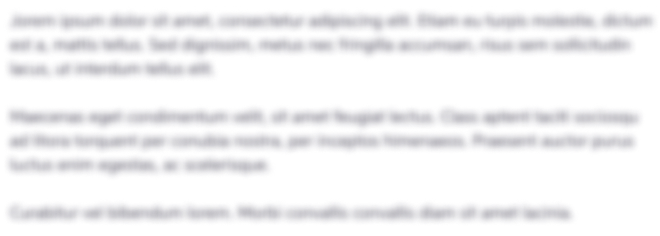
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started