Question
You will create a program that manages the inventory of a store using the TreeMap Data Structure. You will implement the Product class which will
You will create a program that manages the inventory of a store using the TreeMap Data Structure. You will implement the Product class which will hold the name, price, and plu of the Product as outlined below. You will also implement the Store class which will hold a TreeMap of Product objects along with their inventory count. The StoreClient class with a main method will be provided so you can test the classes you create. You will also be provided with a JUnit Test class: ProductTest.java to test your Product class individually. The details of what each method needs to accomplish are described under the Description heading in the table below for the Product and Store classes.
Part 1: Create the Product Class
First create the Product.java file. This class represents a Product in the Store. It has three instance fields: name, price, and plu (Price Look Up code). You will also implement the Comparable Interface so the Product can serve as a key in the TreeMap.
Product Class implements Comparable | Description |
private String name | Instance variable storing the name of the product Example: name could hold "Bananas" |
private double price | Instance variable storing the price for this particular product Example: price could hold 3.99 |
private int plu | Instance variable holding the plu (Price LookUp code) Example: plu could hold 9875 |
public Product(String name, double price, int plu) | Parameterized constructor that instantiates a Product with the given name, price, and plu. |
public double getPrice () | Accessor method that returns the price of this particular product in the store |
public void setPrice(double price) | Mutator method that will set the price for the product |
public String getName() | Accessor method that returns the name of this particular product in the store |
public int getPlu () | Accessor method that returns the plu of this particular product in the store |
public int compareTo(Product other) | The compareTo method will return an integer < 0 if the product's name comes before the other product's name "alphabetically", will return an integer > 0 if the product's name comes after the other product's name "alphabetically" and will return zero if both product names are equal. This method should be case-insensitive. |
public String toString() | Overrides the Objects toString() method to return the state of the product like "Product: Bananas Price: 3.99 PLU: 9875" |
Part 2: Store Class
Next, create the Store.java file. This class will provide the functionality outlined in the table below.
Store Class | Description |
private Map | Instance variable holding a map of all the products and their associated inventory count. Each Product object(the key) in the TreeMap will have an associated inventory count(Integer) as the value. |
public Store(String fileName) | Parameterized constructor which constructs the store's map instance variable. The constructor should use a try-catch statement and process the name, price, and plu information in the file. The file name is passed as a constructor parameter. Each product is on a separate line in the file. As you process the text file, you should identify each product's name, price, and plu. Once a product has been identified, the constructor should call the findProduct(name) method. The findProduct method will return null if the product name was not found in the inventory map. In this case, the constructor should create a new Product object and add it to the map with a count of 1. If the findProduct method returns a non-null Product object, the constructor should get the associated inventory count for this Product object and increment it. |
public Product findProduct(String name) | This method will check if the product is in the inventory map. It can be called from the constructor. If the product's name is in the inventory map, this method will return the Product object it found in the inventory map, otherwise it will return null. |
public String getMostFrequentProduct() | This method will look through the inventory map and return the name for the Product object that has the highest inventory count. |
public Map | Accessor method that returns the inventory map |
public String toString() | This method should return a string representation of the inventory map. It is fine to make use of the built-in toString() for the map. |
Program Input will consist of a text file named products.txt containing name, price, and plu information on separate lines as shown below. Note a space is used to separate each piece of information. You can assume no spaces will be in the individual product names. When you finish your program, your output should resemble what you see below when you run the main method in StoreClient.java.
Program Input: (products.txt)
Milk 5.99 3876 Bananas 3.99 9875 Cheerios 2.99 2431 Bread 4.99 4520 Bread 4.99 4520 Bananas 3.99 9875 Apples 5.99 7654 Bananas 3.99 9875
Program Output: {Product: Apples Price: 5.99 PLU: 7654=1, Product: Bananas Price: 3.99 PLU: 9875=3, Product: Bread Price: 4.99 PLU: 4520=2, Product: Cheerios Price: 2.99 PLU: 2431=1, Product: Milk Price: 5.99 PLU: 3876=1}
Product most frequently bought: Bananas
StoreClient.java
import java.util.*; /** * This class will test the Store class */ public class StoreClient { public static void main(String args[]) { // Store store = new Store("data/products.txt"); // System.out.println(store); System.out.println(); // System.out.println("Toy most frequently bought: " + store.getMostFrequentProduct()); System.out.println(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
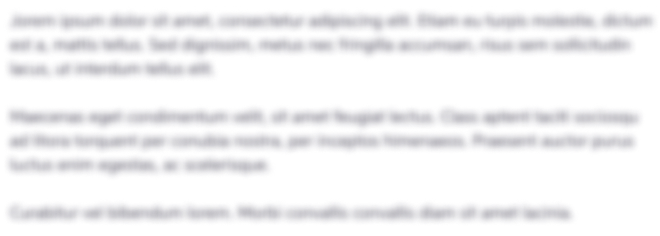
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started