Question
You will re-write your Lab 3 to get the input from a file rather than the user at the keyboard. NOTE : Make sure you
You will re-write your Lab 3 to get the input from a file rather than the user at the keyboard.
NOTE: Make sure you do only what you are asked, do not use concepts that have not been covered. Show me you can follow directions and that you can use the material covered in your book. Please email me with any questions. This lab will use decisions, repetition, functions, files, lists and exception handling.
Remember that program input and output is not the same thing as function input and output.Functioninputis what is passed in on the parameter list, NOT reading data from the keyboard.Function outputis anything returned to the calling function whether it is on the parameter list or in areturnstatement.
Your main will read information out of a file about your contacts and store the information into two lists. The file, contacts.txt, is with the assignment in Blackboard. This file contains records with 2 fields, name and birthdate, each field value is on a separate line. You do not know how many records are in the file so you will read util you get to the end of the file.
You will include exception handling to keep your program from crashing if the file does not exist. You will not be able to complete the program tasks if the file cannot be opened. Your program will just display a message and the program will end if the file cannot be opened.
You will use the concept of parallel lists: each list contains a different piece of information about the contact and the elements are related to each other by position. You will have one list for contact names and one list for birthdates. The name at index 0 in the names list goes with the birthdate at index 0 that is in the birthdates list as shown here:
Index
Names list
Birthdates list
0
Henry Bemis
8/4/2008
1
Penthor Mul
5/3/1928
After displaying the contact information you will display the average age of your contacts.
The names and corresponding birthdates will be stored at the same index but in a different list.
You will no longer need your "get_month" and "get_year" functions, so remove them from your program.
You will modify your "find_season" function so that it now takes as input ONE birthdate string that you read from the file and still return the season as a string. This function will have to isolate the month part of the birthdate so the season can be determined. (Hint: You can use the string split method from Chapter 8 in this lab.)
You will modify your value-returning function "is_leap_year" to take as input ONE birthdate string that you read from the file and will return a Boolean value - True = leap year; False = not a leap year. This function will have to isolate the year part of the birthdate so this decision can be made. (Hint: You can use the string split method from Chapter 8 in this lab.)
You will create a new function called get_age that will take as input the current date (which could be any date from the current date to a date in the future - no dates in the past) and ONE birthdate both as strings in the same format - m/d/yyyy and will return the current age of the contact with that birthdate. (Hint: You can use the string split method from Chapter 8 in this lab.)
Once you have read all records from the file and stored the names and birthdates into their respective lists, you will pass the lists to a function display_contacts that will display the following information in table format with column headings and columns lined up:
NameAgeSeasonLeap Year
Henry Bemis12SummerYes
Penthor Mul92SpringYes
etc....
The display_contacts function will ask the user for the current date in the format m/d/yyyy. The current date may be any date from the current date to any date in the future. It will call get_age(), find_season() and is_leap_year() for each contact one at a time and display the information in table form as shown above.
See the attached document for the correct output for this lab.
You willremovethe penny jar function from your program.
You will have the following functions in your program:
- main
- find_season - takes one birthdate and returns a string
- is_leap_year - takes one birthdate and returns whether it is a leap year or not
- get_age - takes the current date and one birthdate and returns the age of the contact with that birthdate
- display_contacts - takes as input the two lists you created from the entries in the file
You will have TWO lists of strings in your program:
- names
- birthdates
You will not have any other lists that contain info for every contact in the file.
The "split" method produces a list, but that will be the only other list you work with - just the names and birthdates lists.
NOTE- Violating any of the following will get your lab returned to you for correction before I will grade it:
- You will NEVER call main() more than once.
- You will NEVER use break, exit, quit or anything to leave a loop, function, or other construct prematurely.
- You will NEVER have a function call itself.
- You will NEVER use global variables.However, you may use global "constants" if used properly.
- You will haveonly onereturn statement in a function that returns a value.
attached file :-
James B W Bevis 10/12/1943 Henry Bemis 8/4/2008 Romney Wordsworth 8/29/2012 Osborne Cox 1/21/1989 Somerset Frisby 9/5/2010 Revis Jacara 2/16/1935 Bartlet Finchley 11/30/2001 Penthor Mul 5/3/1928 Walter Bedeker 4/27/1996 Clegg Forbes 3/18/2004 Jeremy Wickwire 12/9/1999 Luther Dongle 7/24/1978 Klim Dokachin 10/1/1975 Archibald Beechcroft 6/19/1991 Oliver Crangle 5/6/1954 Agnes Grep 11/4/2013 William Feathersmith 1/11/1967 Kalin Tros 12/28/1955 Clovis Bagwell 7/19/2003 Wallace V Whipple 3/8/1939 Jeff Myrtlebank 8/23/2001 Latham Bine 2/2/1949 Jim Pembry 4/1/1992
Function descriptions should look similar to this:
###############################################
# Function name: function name
# Input: Describe values passed on the parameter list
# Output: Describe the value(s) returned
# Purpose: This function does this...
That was my 3rd lab.
#get the month.
def get_month():
month = int(input('Enter your birth month (1 - 12): '))
if month >= 1 and month <= 12:
return month
# Get the year.
def get_year():
year = int(input('Enter your birth year: '))
if year >= 1 and year <= 2021:
return year
# is a lap year or not.
def is_leap_year(year):
if (year % 4) == 0:
if (year % 100) == 0:
if (year % 400) == 0:
return True
else:
return False
else:
return True
else:
return False
# find the season.
def find_season(month):
winter = [1, 2, 12]
spring = [3, 4, 5]
summer = [6, 7, 8]
fall = [9, 10, 11]
if month in winter:
return "winter"
if month in spring:
return "spring"
if month in summer:
return "summer"
if month in fall:
return "fall"
# Ask user the penny in jar.
def penny_jar(pennies):
dollars = pennies // 100
pennies %= 100
quarters = pennies // 25
pennies %= 25
dimes = pennies // 10
pennies %= 10
nickels = pennies // 5
pennies %= 5
if dollars > 1:
print(dollars, "dollars")
elif dollars == 1:
print("1 dollar")
if quarters > 1:
print(quarters, "quarters")
elif quarters == 1:
print("1 quarter")
if dimes > 1:
print(dimes, "dimes")
elif dimes == 1:
print("1 dime")
if nickels > 1:
print(nickels, "nickels")
elif nickels == 1:
print("1 nickel")
if pennies > 1:
print(pennies, "pennies")
elif pennies == 1:
print("1 penny")
# ask user name.
def main():
name = input('Enter your name: ')
month = get_month()
year = get_year()
if is_leap_year(year):
print("Hello, {name}! You were born in the {find_season(month)} and {year} was a leap year.")
else:
print("Hello, {name}! You were born in the {find_season(month)} and {year} was not a leap year.")
pennies = int(input("How many pennies do you have in your penny jar? "))
penny_jar(pennies)
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
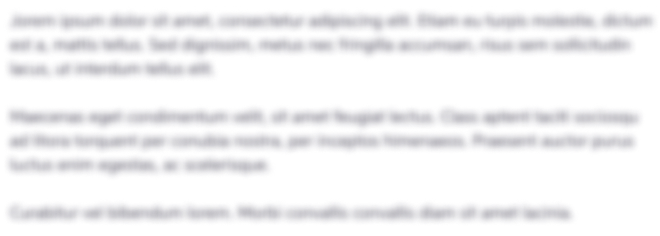
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started