Question
you will write and read your class SalaryEmployee to and from a file salary_employee.csv in format of comma separates values . You must use your
you will write and read your class SalaryEmployee to and from a file salary_employee.csv in format of comma separates values . You must use your own my_join and my_split functions.
Program menu as below:
(1) Add employee to employees
(2) Write employees to file
(3) Read employees from file
(4) Print employees
(0) Quit
With menu option 1 you will read employee names and their salary to objects.
With menu option 2 you will write all objects to csv-file.
With menu option 3 you will read objects from csv-file.
With menu option 4 you will print objects and their values in a format like: Id: 1 Name: Jane Doe Salary: 5555.
With menu option 0 you will quit the execution of program.
Your job is to code for menu options 2 and 3.
Write employees to file:
make sure that file salary_employee.csv is empty,
go thru salary employees, add its attributes to a list and use my_join to make csv-string from that list,
complete string with newline and write it to file,
when all employees are handled, remember to close the filehandle,
add the following code to the end: print(len(salary_employees) ," employee(s) added to salary_employee.csv").
Read employees from file:
open file salary_employee.csv for reading,
go thru the file line by line,
strip newline and use my_split to make attribute list from string,
make SalaryEmployee object and add it to salary_employees list,
close file when all lines are read,
add following code to the end: print(len(salary_employees) ," employee(s) read from salary_employee.csv").
**Expected output**
(1) Add employee to employees
(2) Write employees to file
(3) Read employees from file
(4) Print employees
(0) Quit
Please select one: 1
Please enter employee name (0 to quit):Jane Doe
Please enter salary:5555
Please enter employee name (0 to quit):John Johnson
Please enter salary:4567
Please enter employee name (0 to quit):Richard Roe
Please enter salary:3636
Please enter employee name (0 to quit):0
(1) Add employee to employees
(2) Write employees to file
(3) Read employees from file
(4) Print employees
(0) Quit
Please select one: 2
3 employee(s) added to salary_employee.csv
(1) Add employee to employees
(2) Write employees to file
(3) Read employees from file
(4) Print employees
(0) Quit
Please select one: 4
Id: 1 Name: Jane Doe Salary: 5555
Id: 2 Name: John Johnson Salary: 4567
Id: 3 Name: Richard Roe Salary: 3636
(1) Add employee to employees
(2) Write employees to file
(3) Read employees from file
(4) Print employees
(0) Quit
Please select one: 3
3 employee(s) read from salary_employee.csv
(1) Add employee to employees
(2) Write employees to file
(3) Read employees from file
(4) Print employees
(0) Quit
Please select one: 4
Id: 1 Name: Jane Doe Salary: 5555
Id: 2 Name: John Johnson Salary: 4567
Id: 3 Name: Richard Roe Salary: 3636
(1) Add employee to employees
(2) Write employees to file
(3) Read employees from file
(4) Print employees
(0) Quit
Please select one: 0
Service shutting down, thank you.
code:
def my_split(sentence, sep):
lst = []
tmp = ''
for c in sentence:
if c == sep:
lst.append(tmp)
tmp = ''
else:
tmp += c
if tmp:
lst.append(tmp)
return(lst)
def my_join(lst,sep):
mystr = ''
for elem in lst[0:-1]:
mystr += str(elem) + str(sep)
mystr += str(lst[-1])
return(mystr)
class Employee:
def __init__(self, id, name):
self.id = id
self.name = name
class SalaryEmployee(Employee):
def __init__(self, id, name, monthly_salary):
super().__init__(id, name)
self.monthly_salary = monthly_salary
def calculate_payroll(self):
return self.monthly_salary
while True:
print("(1) Add employee to employees(2) Write employees to file(3) Read employees from file(4) Print employees(0) Quit")
selection = int(input("Please select one: "))
if selection == 1:
salary_employees = []
name = " "
id = 1
while name != '0':
name = str(input("Please enter employee name (0 to quit):"))
if name != '0':
try:
salary = int(input("Please enter salary:"))
except:
salary = 0
salary_employees.append(SalaryEmployee(id,name,salary))
id += 1
### put your code here ###
elif selection == 4:
for employee in salary_employees:
print("Id:",employe.id, "Name:", employee.name, "Salary:", employee.monthly_salary)
elif selection == 0:
print("Service shutting down, thank you.")
break
else:
print("Incorrect selection.")
Step by Step Solution
3.53 Rating (156 Votes )
There are 3 Steps involved in it
Step: 1
To implement menu options 2 and 3 youll need to read and write SalaryEmployee objects to and from a CSV file You should also update the code structure ...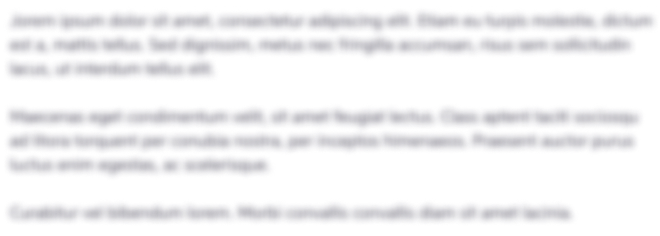
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started