Question
Your assignment is to implement a Binary Search Tree using the employee class you developed in Week 2. The employee class should contain the employee
Your assignment is to implement a Binary Search Tree using the employee class you developed in Week 2. The employee class should contain the employee information listed in the input section below. The program should contain all functions listed in the functionality section below.
Input Data about current employees should be on the file "Employee.txt". You will need to create your own employee file and submit it with the final project. Each employee should have the following attributes:
Employee_Number Integer
Employee_Last_Name String
Employee_First_Name String
Employee_Years_of_Service Integer
3 employees must be loaded from the Employee.txt file on program start up.
Functionality
Command | Processing |
ADD | Allows the user to Add an employee to the tree |
REMOVE | Allows the user to Remove an employee from the tree |
COUNT | Returns the number of Employees in the tree |
| Prints the employee information in the list to the console |
QUIT | Stops processing |
Output
All output should be on the console.
Data Structures
This program should utilize a binary search tree.
here is what I have to start....
main.cpp
#include
using namespace std;
#include "node.h" #include "employee_list.h"
int main() { //new list Employee list;
//append nodes to list string line; ifstream fin;
//reading the data from file Employee.txt fin.open("Employee.txt"); if (fin.is_open()) { while (getline(fin, line)) { stringstream ss(line); int emp_number, emp_year_of_service; string first_name, last_name; ss >> emp_number; ss >> last_name; ss >> first_name; ss >> emp_year_of_service;
//loading three records from the file employee.txt to the list list.Add(emp_number, last_name, first_name, emp_year_of_service); } }
//command utility string command; cout << "Enter desired command -> Add, Remove, Count, Print, Quit: "; cin >> command; while (command != "Quit") {
//print if (command == "Print")list.Print();
//add if (command == "Add") { int emp_number, emp_year_of_service; string first_name, last_name; cout << "Enter Employee Number: "; cin >> emp_number; cout << "Enter Last Name: "; cin >> last_name; cout << "Enter First Name: "; cin >> first_name; cout << "Enter Year of Service: "; cin >> emp_year_of_service; list.Add(emp_number, first_name, last_name, emp_year_of_service); }
//remove if (command == "Remove") { int emp_number, emp_year_of_service; string first_name, last_name; cout << "Enter Employee Number: "; cin >> emp_number; cout << "Enter Last Name: "; cin >> last_name; cout << "Enter First Name: "; cin >> first_name; cout << "Enter Year of Service: "; cin >> emp_year_of_service; list.Delete(emp_number, first_name, last_name, emp_year_of_service); }
//count if (command == "Count")cout << " The Number of employees in the list is: " << list.Count() << " ";
//enter command again cout << " Enter desired command -> Add, Remove, Count, Print, Quit: "; cin >> command; } }
employee_list.h
//employee class
class Employee { Node *head; public: Employee() { head = NULL; }; void Print(); int Count(); void Add(int emp_number, string last_name, string first_name, int emp_year_of_service); void Delete(int emp_number, string last_name, string first_name, int emp_year_of_service); };
//print command void Employee::Print() {
//temporary pointer Node *tmp = head;
//if empty if (tmp == NULL) { cout << "EMPTY" << endl; return; }
//one node in list if (tmp->Next() == NULL) { cout << "Employee #:" << tmp->return_emp_no() << ", Last Name: " << tmp->return_last_name() << ", First Name: " << tmp->return_first_name() << ", Years of Service: " << tmp->year_of_service() << " year(s)."; cout << endl; cout << ""; cout << "End of Line - Null" << endl; } else
{
//print the list do { cout << "Employee #:" << tmp->return_emp_no() << ", Last Name: " << tmp->return_last_name() << ", First Name: " << tmp->return_first_name() << ", Years of Service: " << tmp->year_of_service() << " year(s)."; cout << endl; cout << ""; tmp = tmp->Next(); } while (tmp != NULL); cout << " End of Line - Null" << endl; } }
//count command int Employee::Count() { //temporary pointer Node *tmp = head; int i = 0; //if empty << "years."; if (tmp == NULL) { cout << "EMPTY" << endl; return i; }
//one node in list if (tmp->Next() == NULL) { return 1; } else {
//print the list do { i++; tmp = tmp->Next(); } while (tmp != NULL); return i; } }
//add node to the linked list void Employee::Add(int emp_number, string last_name, string first_name, int year_of_service) {
//create new node Node* newNode = new Node(); newNode->SetData(emp_number, last_name, first_name, year_of_service); newNode->SetNext(NULL);
//create tempoary pointer Node *tmp = head; if (tmp != NULL) {
//if nodes already present move to end of list while (tmp->Next() != NULL) { tmp = tmp->Next(); }
//point last to new tmp->SetNext(newNode); } else {
//first node in list head = newNode; } }
//delete node from the list void Employee::Delete(int emp_number, string last_name, string first_name, int emp_year_of_service) { //create tempoary pointer Node *tmp = head;
//no nodes if (tmp == NULL) return;
//first node of the list if (tmp->return_emp_no() == emp_number && last_name.compare(tmp->return_first_name()) == 0 && first_name.compare(tmp->return_last_name()) == 0 && tmp->year_of_service() == emp_year_of_service) { head = tmp->Next(); delete tmp; } else {
//move thru the nodes Node *prev = NULL; do { if (tmp->return_emp_no() == emp_number && last_name.compare(tmp->return_first_name()) == 0 && first_name.compare(tmp->return_last_name()) == 0 && tmp->year_of_service() == emp_year_of_service) break; prev = tmp; tmp = tmp->Next(); } while (tmp != NULL);
//adjust pointers prev->SetNext(tmp->Next());
//delete current node delete tmp; } }
node.h
//node class
class Node { int emp_number; string emp_last_name; string emp_first_name; int emp_year_of_service; Node* next;
public: //constructor Node() {};
//assigning employees details void SetData(int e_no, string last_name, string first_name, int year_of_service) { emp_number = e_no; emp_last_name = last_name; emp_first_name = first_name; emp_year_of_service = year_of_service; };
//setting the next node void SetNext(Node* aNext) { next = aNext; };
//returning the data in the node int return_emp_no() { return emp_number; }; string return_last_name() { return emp_last_name; }; string return_first_name() { return emp_first_name; }; int year_of_service() { return emp_year_of_service; }; Node* Next() { return next; }; };
Step by Step Solution
There are 3 Steps involved in it
Step: 1
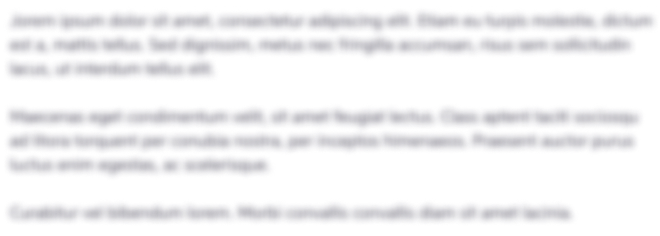
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started