Question
Your goal is to create, use, and test a Timer class. A Timer object should basically be equivalent to a stopwatch. Like a stopwatch, you
Your goal is to create, use, and test a Timer class. A Timer object should basically be equivalent to a stopwatch. Like a stopwatch, you must be able to start the Timer, stop the Timer, and clear the Timer. In addition, you must be able to getTime from the Timer. At a minimum, your class must contain the following member functions: void start(); // Starts the Timer void stop(); // Stops the Timer void clear(); // Clears time on the Timer float getTime(); // Returns elapsed time in seconds It will be up to you to implement the member functions identified within the Timer.h file. They must all be delcared as public member functions as they represent the standard methods in which users will interact with Timer objects. Therefore, those interactions would be labeled as public. The descriptions of the functions are as follows: 1. start() This method starts or resumes keeping track of the elapsed time on the Timer object. If the Timer is already running (via start()), then this method should have no impact. If the Timer is not currently running, then it will resume counting time from where it left off. 2. stop() This method will stop the Timer from adding to the elapsed time. If the Timer is already stopped, then this method should have no impact. 3. clear() Resets the internal counter representing elapsed time. This does not impact start() or stop(). If the Timer is already running (via start()) then the elapsed time will be cleared to zero and will then continue to count up. If the Timer is not running (having called stop()) then the elapsed time will be cleared to zero and the elapsed time will not increase until start() is called again. 4. float getTime() Returns the time that has elapsed in seconds. If the Timer is currently running then successive calls to getTime() should return larger values. If the Timer is not running, then successive calls to getTime() should return the same value
You are free to create additional member functions if you wish, however the ones listed above are the only member functions that should be public. Additional member functions would only be used to support the member functions above and therefore ought to be private as they would not be called externally by the user. Getting the time In order to provide the functionality of a timer, youll need to be aware of the current time. In the Timer.h file that I provide, you will find a private member function called now() that you can use to fetch the number of milliseconds that have passed since midnight on January 1, 1970. I wrote this code which uses the C++ chrono (time) library. With this now() method, you can get check the current time at two different points in time and subtract the older value (the start time) from the more recent value (the stop time) in order to find the difference between them (the elapsed time in microseconds). Please use this now() member function to get those snapshots of time to implement your Timer functionality. One note: be aware that the now() method returns a value relating to elapsed microseconds in 64-bit integer form (long long int). The getTime() method requires you to return elapsed seconds in float form. Therefore, be sure to convert from microseconds to seconds appropriately by dividing by your integer microsecond values by 1000000.0 (note the decimal point which allows the result also be a float). Use the support code and ideas I provide to write your own Timer class. Once completed, you should be able to create an instance of your class and invoke/use it in the following way inside of your ex 1.cpp file: #include
the Timer.h code
#pragma once
#include
class Timer {
private:
// TODO: Put any private member variables you want here
public:
Timer() {
// TODO: Initialize any member variables you'd like here
}
void start() {
// TODO: Implement me
}
void stop() {
// TODO: Implement me
}
void clear() {
// TODO: Implement me
}
float getTime() {
// TODO: Implement me
return -1; // TODO: Change me!
}
private:
// A private member function that you may find useful as you implement
// the functions listed above. It fetches the number of microseconds that
// have passed since 12:00 AM on Jan 1, 1970
// Don't worry too much about how it works right now; just trust me! :)
long long int now() {
using namespace std::chrono;
duration
return t.count();
}
// TODO: Put any additional private member functions you want here
};
the ex_1.cpp code
#include
#include
#include "Timer.h"
using namespace std;
int main() {
Timer t;
t.start(); // Start the clock
sleep(2); // Sleep for 2 seconds
cout << t.getTime() << endl; // Should print approximately 2.0
sleep(1); // Sleep for 1 second
t.stop(); // Stop the clock
cout << t.getTime() << endl; // Should print approximately 3.0
t.clear(); // Reset the stopped clock
cout << t.getTime() << endl; // Should print 0
return 0;
}
PLEASE USE C++ language
Step by Step Solution
There are 3 Steps involved in it
Step: 1
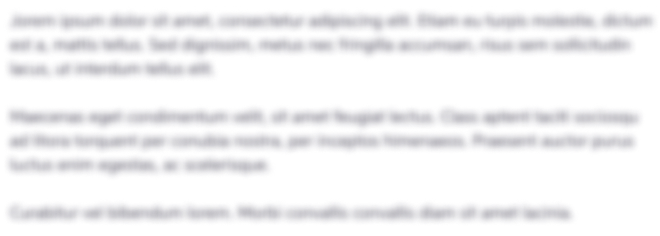
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started