Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
Your job is to design and create a program to calculate the letter grade based on a numerical score. You will first read the numerical
Your job is to design and create a program to calculate the letter grade based on a numerical score. You will first read the numerical scores from the user as input into a double array, and then calculate the letter grade and fill another parallel array and print the two arrays as output. The numerical score must be between and inclusive. The user should not be allowed to enter a score less than or greater than You will also sort the arrays based on the scores, and calculate the median of the scores and output the sorted arrays and the median in main Write the following functions and call them in the same order as instructed in the main function to accomplish this Task. void welcome This function prints a welcome message to the user. void readScoresdouble scores int &count Reads a list of scores from the user. A indicates the end of the input and is not stored in the array. Use an appropriate loop!! Check out this example code a for loop is count controlled, and a while loop is condition based. Call the readDouble function to do this. You must catch all invalid data such as characters, negative numbers other than etc. The variable count keeps track of the number of scores entered. You must also do validation to make sure that the number is between and inclusive and nothing other than that. Use a while loop to do this. void readDoublestring prompt, double &num This function should be used any time you read any floats or doubles from the user. Use this function to read the numerical scores from the user. It takes a string prompt, outputs it reads a number from the user, validates and returns the num by reference. Write it exactly like the readInt from assignment but declare a double or float instead of an int. See Sampleacpp for the readInt function. You must catch all invalid data such as characters, negative numbers etc. void calcGradedouble scores char grade int count Use a loop to process each array element and calculate the letter grade for each score. Use the table below to assign grades A to F to the corresponding numerical score. Letter Grade Score Level A Exceeds B Meets C Approaching D Not Yet F No Evidence void printListdouble scores char grades int count Go through a for loop and print the scores and the corresponding grades for each item. void sortdouble scores char grade int count Sort the arrays using the given sorting algorithm. This is called Selection Sort. Use only this algorithm to sort your list. To see how the Selection Card Sort Algorithm works, watch this video from Virginia Tech. Be careful and make sure you sort based on the scores array and swap the corresponding element in the grade array to maintain the correspondence between the two arrays. Meaning if you swap the scores in index and you must also swap the corresponding grades in index and Watch this Python Video to help you with the sorting algorithm. Try this Selection Sort Animation by Y Daniel Liang. procedure selection sort list : array of items count : size of list for i to count set current element as minimum min i go through the list and find the smallest element for j i to count if listj listmin then min j; end if end for swap the minimum element with the current element If they are not the same element if min i then swap listmin and listi end if end for end procedure double mediandouble scores int countnew to this level After sorting, write this function to identify the median score. The median is located in the middle of the array if the arrays size is odd. Otherwise, the median is the average of the middle two values. Return the median to main and output in main with two decimal places. int main Declare all variables needed. The arrays double scores and char grades must be declared in main Call readScores and send scores and count to it This will fill the scores array from the user. You should call the readDouble function to read and validate the scores before adding them to the scores array. count will have the number of values read. Call calcGradefunction that takes the scores array and an empty grades array and fills the grades array with letter grades. Call the printList function to print the lists. Call the sort function to sort the list based on scores. Call the printList function to print the lists. Call the median function to find the median and print it in main Assume the arrays will always contain fewer than values. You must not let the user enter more than values. Open the Algorithmic Design Document, make a copy, and follow the steps to create your algorithm. You must express your algorithm as pseudocode. Check ou
Your job is to design and create a program to calculate the letter grade based on a numerical score. You will first read the numerical scores from the user as input into a double array, and then calculate the letter grade and fill another parallel array and print the two arrays as output.
The numerical score must be between and inclusive. The user should not be allowed to enter a score less than or greater than
You will also sort the arrays based on the scores, and calculate the median of the scores and output the sorted arrays and the median in main
Write the following functions and call them in the same order as instructed in the main function to accomplish this Task.
void welcome This function prints a welcome message to the user.
void readScoresdouble scores int &count
Reads a list of scores from the user. A indicates the end of the input and is not stored in the array. Use an appropriate loop!! Check out this example code a for loop is count controlled, and a while loop is condition based.
Call the readDouble function to do this. You must catch all invalid data such as characters, negative numbers other than etc.
The variable count keeps track of the number of scores entered.
You must also do validation to make sure that the number is between and inclusive and nothing other than that. Use a while loop to do this.
void readDoublestring prompt, double &num
This function should be used any time you read any floats or doubles from the user. Use this function to read the numerical scores from the user.
It takes a string prompt, outputs it reads a number from the user, validates and returns the num by reference.
Write it exactly like the readInt from assignment but declare a double or float instead of an int. See Sampleacpp for the readInt function.
You must catch all invalid data such as characters, negative numbers etc.
void calcGradedouble scores char grade int count
Use a loop to process each array element and calculate the letter grade for each score.
Use the table below to assign grades A to F to the corresponding numerical score.
Letter Grade
Score
Level
A
Exceeds
B
Meets
C
Approaching
D
Not Yet
F
No Evidence
void printListdouble scores char grades int count
Go through a for loop and print the scores and the corresponding grades for each item.
void sortdouble scores char grade int count
Sort the arrays using the given sorting algorithm. This is called Selection Sort. Use only this algorithm to sort your list. To see how the Selection Card Sort Algorithm works, watch this video from Virginia Tech.
Be careful and make sure you sort based on the scores array and swap the corresponding element in the grade array to maintain the correspondence between the two arrays. Meaning if you swap the scores in index and you must also swap the corresponding grades in index and
Watch this Python Video to help you with the sorting algorithm. Try this Selection Sort Animation by Y Daniel Liang.
procedure selection sort
list : array of items
count : size of list
for i to count
set current element as minimum
min i
go through the list and find the smallest element
for j i to count
if listj listmin then
min j;
end if
end for
swap the minimum element with the current element
If they are not the same element
if min i then
swap listmin and listi
end if
end for
end procedure
double mediandouble scores int countnew to this level
After sorting, write this function to identify the median score. The median is located in the middle of the array if the arrays size is odd. Otherwise, the median is the average of the middle two values.
Return the median to main and output in main with two decimal places.
int main
Declare all variables needed. The arrays double scores and char grades must be declared in main
Call readScores and send scores and count to it This will fill the scores array from the user. You should call the readDouble function to read and validate the scores before adding them to the scores array.
count will have the number of values read.
Call calcGradefunction that takes the scores array and an empty grades array and fills the grades array with letter grades.
Call the printList function to print the lists.
Call the sort function to sort the list based on scores.
Call the printList function to print the lists.
Call the median function to find the median and print it in main
Assume the arrays will always contain fewer than values. You must not let the user enter more than values.
Open the Algorithmic Design Document, make a copy, and follow the steps to create your algorithm.
You must express your algorithm as pseudocode.
Check ou
Step by Step Solution
There are 3 Steps involved in it
Step: 1
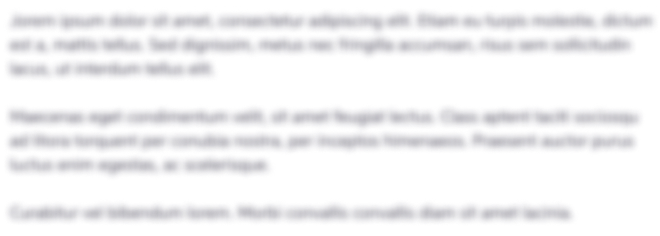
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started