Question
Your job is to develop a simple version of mathematics tool on linear algebra. Assuming you are the developer for supporting those APIs for other
Your job is to develop a simple version of mathematics tool on linear algebra. Assuming you are the developer for supporting those APIs for other programmers to use. Your role is responsible for designing a Matrix structure and provide some basic containers to hold these matrices, such as stack or queue. There are three class files involved, one is called "Matrix", which defines the matrix structure and some basic function about the matrix; the second class is called "StackContainer", which is used to store different matrices in a stack structure; the third class is called "QueueContainer", which is used to store different matrices in a queue structure. After finishing implementing these three classes, write a "Main" class file in the "main" package to test it. This class is pretty much a test class that has "main()" function inside it. The basic structure for these three files of the project is as the image blow:
Packages:
For this project, there are three packages you need to create: "linear.algebra.types", "linear.algebra.containers", and "test". For the "linear.algebra.types" package, it has a single "Matrix.java" file; for the "linear.algebra.containers", it has two files: "StackContainer.java" and "QueueContainer.java"; for the "test" package, it has a "Main.java", which holds the "main()" function in the Main class. The structure for these files are as the figure 1 shows below:
II.Your Three Tasks:
(1) Implement the functions of the three classes "Matrix", "StackContainer", and "QueueContainer".
(2) Put "import" in different files properly at the beginning of the associated file(s) to make sure the access is visible across different packages.
(3) Put some testing code into the "main" function of the "Main" class to evaluate your "Matrix", "StackContainer", "QueueContainer". This code for Main class is flexible. To save your time, you can use the provided "testing" code (check your email for the testing code)
III. Classes Details and Rubrics
"Matrix" class:
Field (data) Members (5%):
(1) "data" - use a 2D array to store the values for all the elements. The type for the array is "double".
(2) "rows" - defining the number of rows of the matrix, using "int".
(3) "cols" - defining the number of columns of the matrix, using "int".
Method (function) Members:
(1) Constructor 1 (2%) - empty arguments (default constructor). Assign 1 for both the row and column and 0.0 for all the elements.
(2) Constructor 2 (3%) - two arguments (int, int), assigning the row and column properties with all the elements equal to 0.
(3) Constructor 3 (5%) - three arguments (int, int, double), assigning the elements with the third argument "double", which is an 2D array. You need to do some checking to make sure the input 2D array should have at least the same number of rows and columns as the first two input arguments. It is fine if the passed 2D array supports more data than the rows and columns needed. You can simply ignore those extra numbers. (Hint: to check the size of the array, use the "length" data member of an array object)
(4) Constructor 4 (5%) -three arguments (int, int, double). This is very similar to the "constructor 3". The only difference is the third argument, which is a 1D array. It is a bit tricky here that you need to convert the 1D into 2D yourself. We assume the elements in the 1D array is in row wise. e.g. a[] = {5, 2, 3, 4, 3, 2}, if you store this array into a 2 x 3 matrix. It is like 5, 2, 3 for the first row, and 4, 3, 2 for the second row.
(5) void printMat() (5%) - print the matrix data row by row. e.g. if the matrix has data [2][3] = {5, 2, 3, 4, 3, 2}, then this function will print it out on the console:
5, 2, 3,
4, 3, 2
(6) void copyTo(Mat mat) (5%) - copy the value of current matrix to the input variable "mat". e.g. Mat a; Mat b; ...; a.copyTo(b);//b will be identical to a
(7) setters and getters (5%) - In Java, it is a convention that the data fields are "private". If you want to access or modify these data of the corresponding object, you should use the corresponding "public" functions to finish the job. Fortunately, Eclipse provide a way to automatically generate these piece of code for you. Click the "Source" in the menu, then choose something about "setters and getter". You will use the wizard to generate the corresponding functions.
"StackContainer" class:
This class is used to store Matrix elements in a stack structure (First-In-Last-Out).If you forget the way stack works, you are suggested to find online tutorials on stack structure. In fact, Java has already provided a well implemented class "java.util.Stack". So you can directly use this class as the data member for your "StackContainer" class. In other word, "StackContainer" is like a wrapper class. For all the member functions as described below, you can directly utilize the provided functions in "java.util.Stack" class.
Field (data) Members:
Stack
Method (function) Members:
- stackIsEmpty(); (5%)//Check whether the stack is empty, the return type should be "boolean". If the stack is empty, returns true; otherwise returns false.
- stackAddItem(Matrix item) (5%)//This function is to push input parameter "item" into the stack. No return value is needed.
- stackRemoveItem()(5%)//This function is to remove the top (youngest) element from the stack. No return value is needed.
- Matrix stackPeekItem()(5%)//Different from "stackRemoveItem()", this function is just retrieve the top element from the stack without removing it. So the return value is a Matrix.
- stackPrintAllItems()(5%)//This function is to display all the elements in the stack in the right order. No return value is needed.
"QueueContainer" class:
This class is used to store Matrix elements in a queue structure (First-In-First-Out).Similar to the "StackContainer" class, you will use it to store matrices. This class has a set of similar functions as "StackContainer" class. However, there is a slightly difference in the way of implementation. Instead of using Java provided queue class, you will use "LinkedList" class to hold the data as described below.
Field (data) Members:
LinkedList data;(5%)
//This is static and private data member, which is used to hold all the matrix data. "LinkedList" class is a well implemented Java class. So you need to look it up online and find out how this class works.
Method (function) Members:
All the function members are supposed to be public.
(1) queueIsEmpty()(5%)//Check whether the queue is empty, the return type should be "boolean". If the queue is empty, returns true; otherwise returns false.
(2) queueAddItem(Matrix item) (5%)//This function is to push input parameter "item" into the queue. No return value is needed.
(3) queueRemoveItem()(5%)//This function is to remove the oldest element from the queue. No return value is needed.
(4) Matrix queuePeekItem()(5%)//Different from "queueRemoveItem()", this function is just retrieve the oldest element from the queue without removing it. So the return value is a Matrix.
(5) queuePrintAllItems()(10%)//This function is to display all the elements in the queue in the right order. No return value is needed.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
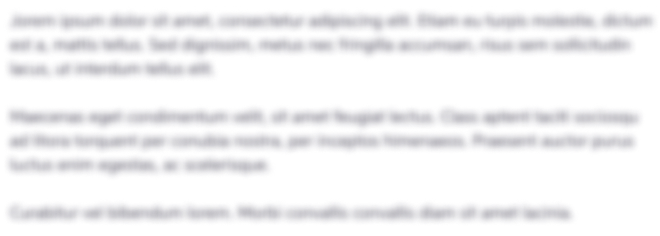
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started