Question
Your PriorityQueue will store Task objects, defined by the Task class below. Two member attributes in Task use enum types Status and Priority, as defined
Your PriorityQueue will store Task objects, defined by the Task class below.
Two member attributes in Task use enum types Status and Priority, as defined as enum "classes" that will be used in your Task class. Notice the order of the Priority enumerations. This is the sort order, your queue will use:
class Task implements Comparable{ int taskId; String subject; Priority priority; Status status; LocalDateTime startDate; LocalDateTime dueDate; }
enum Priority { URGENT, HIGH, NORMAL, LOW} enum Status{ NOT_STARTED, IN_PROGRESS, WAITING, DEFERRED }
You will create an interactive test driver as we did for the BinarySearch exercise. A text-based driver is fine but if you are feeling ambitious, you are welcome to create a simple graphical UI (definitely not required). Your interactive test driver loop will allow users to:
- Add a task. This will require you to capture the subject, priority, and dueDate. Here are some tips on using Java LocalDates. Use now() for the startDate. TaskId should start at 1 and increment each time a task is added.
- View the next task in the queue using the peek() method and then optionally complete the current task using the poll() method.
- View a list of all tasks. This will require the use of an iterator and the peek() method on each element. Use isEmpty() to check if there are items in your queue and if not, display "No tasks in queue."
- View a single task by id. This will require iterating the task list until the id is found and then displaying it.
- Remove a task by id. This will require you to iterate through the Task queue until you find the task with the matching id. Use remove(task) and confirm to the user that the task has been removed.
- For readability, create a public toString method so that you can easily print queue elements:
@Override public String toString() { return "Id:" + taskId + "; Subject: " + subject + "; Status: " + status + "; Priority: " + priority + "; StartDate: " + startDate.toString() + "; Due date: " + dueDate; }
- In order to force PriorityQueue to use Priority as your sorting criterion, you should override the default comparator for the sort by adding this method to your Task class. Task should implements Comparable
- @Override public int compareTo(Task task) { return this.getPriority().compareTo(task.getPriority()); } Note: If your PriorityQueue is just a list of numbers or simple strings, you won't need your own comparator, but in our case, we are using a more complex object-type, so we need to create our own.
- Here is a priority helper method for your test-driver if you want, as a freebie:
private Priority scanForPriority() { System.out.println("Enter priority abbreviation Normal=n,Low=l,High=h,Urgent=u): ");String abbrev = scanner.nextLine(); switch (abbrev) { case "n": priority = Priority.NORMAL; break; case "l": priority = Priority.LOW; break; case "h": priority = Priority.HIGH; break; case "u": priority = Priority.URGENT; break; } return priority; }
You should create your own scanForStatus() method that follows the pattern above. Use a Do-While loop to code up a test driver method for your PriorityQueue interactive testing. Here is a screenshot of a portion of one solution for your "menus" as a hint, in case you are confused. Try it before you look:
public void testPriorityQueue() { System.out.println("Welcome to My Task List "); System.out.println("Choose action (Add(a),Next(n),List(l),Detail(d),Edit(e),Remove(r),Quit(q): "); String menuItem = scanner.nextLine(); do { switch (menuItem) { case "a": addTask(); break; case "n": displayNextTask(); break; case "l": showTaskList(); break; case "e": System.out.println("Enter taskId: "); int taskId = parseInt(scanner.nextLine()); Task task = getTaskById(taskId); editTask(task); break; case "d": System.out.println("Enter taskId: "); taskId = parseInt(scanner.nextLine()); showTaskDetail(taskId); break; case "r": System.out.println("Enter taskId: "); taskId = parseInt(scanner.nextLine()); removeTask(taskId); case "q": break; } System.out.println("Choose action (Add(a),Next(n),List(l),Detail(d),Edit(e),Remove(r),Quit(q): "); menuItem = scanner.nextLine(); if (menuItem.equals("q")) break; } while (menuItem != "q"); System.out.println("Goodbye"); }
Template
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Iterator;
import java.util.PriorityQueue;
import java.util.Scanner;
import java.lang.Integer;
enum Priority {
URGENT,
HIGH,
NORMAL,
LOW
}
enum Status {
NOT_STARTED,
IN_PROGRESS,
WAITING,
DEFERRED
}
class Task implements Comparable
int taskId;
String subject;
Priority priority;
Status status;
LocalDate startDate;
LocalDate dueDate;
public Task(int taskId, String subject, Priority priority, Status status, LocalDate startDate, LocalDate dueDate) {
this.taskId = taskId;
this.subject = subject;
this.priority = priority;
this.status = status;
this.startDate = startDate;
this.dueDate = dueDate;
}
public int getTaskId() { return taskId;
}
@Override
public String toString() { return "Id:" + taskId + "; Subject: " + subject + "; Status: " + status + "; Priority: " + priority + "; StartDate: " + startDate.toString() + "; Due date: "+ dueDate;
}
public Priority getPriority() { return priority; } @Override public int compareTo(Task task) {
return this.getPriority().compareTo(task.getPriority()); }
}
public class ToDoListApplication {
PriorityQueue
Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
ToDoListApplication app = new ToDoListApplication();
app.testPriorityQueue();
}
public void displayNextTask() {
// add your code here
}
public void showTaskDetail(int taskId) {
Task task = getTaskById(taskId);
// add your code here to print the task.
}
public Task getTaskById(int taskId) {
Iterator it = taskPriorityQueue.iterator();
while (((Iterator) it).hasNext()) {
Task task = (Task) it.next(); if (task.getTaskId() == taskId) return task;
System.out.println("TaskId not found...");
}
return null;
}
public void removeTask(int taskId) {
// Add your code here
}
public void testPriorityQueue() {
System.out.println("Welcome to My Task List ");
do {
System.out.println("Choose action (Add(a), Next(n), List(l), Detail(d),Edit(e), Remove(r), Quit(q):");
String menuItem = scanner.nextLine();
switch (menuItem) {
case "a":
addTask();
break;
case "n":
displayNextTask();
break;
case "l":
showTaskSummaryList();
break;
case "d":
System.out.println("Enter taskId: ");
int taskId = Integer.parseInt(scanner.nextLine());
showTaskDetail(taskId);
break;
case "r":
System.out.println("Enter taskId: ");
taskId = Integer.parseInt(scanner.nextLine());
removeTask(taskId);
break;
case "q":
break;
}
} while (true);
}
void showTaskSummaryList() {
for (Task task: taskPriorityQueue)
System.out.println(task);
}
private Priority scanForPriority() {
Priority priority = Priority.NORMAL;
System.out.println("Enter priority abbreviation Normal=n,Low=l,High=h,Urgent=u): ");
String abbrev = scanner.nextLine();
switch (abbrev) {
case "n":
priority = Priority.NORMAL;
break;
case "l":
priority = Priority.LOW;
break;
case "h":
priority = Priority.HIGH;
break;
case "u":
priority = Priority.URGENT;
break;
}
return priority;
}
void addTask() {
System.out.println("Adding new Task...");
System.out.println("Enter subject:");
Scanner scanner = new Scanner(System.in);
String subject = scanner.nextLine();
System.out.println("Enter due date (yyyy-MM-dd):");
String input = scanner.nextLine();
DateTimeFormatter dateFormat = DateTimeFormatter.ofPattern("yyyy-MM-dd");
LocalDate dueDate = LocalDate.parse(input);
Priority priority = scanForPriority();
Status status = Status.NOT_STARTED;
LocalDate date = LocalDate.now();
LocalDate startDate = LocalDate.now();
Task task = new Task(taskPriorityQueue.size() + 1, subject, priority, status, startDate, dueDate);
taskPriorityQueue.add(task);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
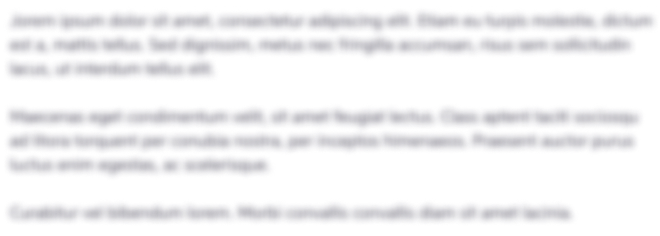
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started