Question
Your program will expect a file name to be on the commands line when the program is launched: C:> java Project2 dictionary.txt Your Project2.java defines
Your program will expect a file name to be on the commands line when the program is launched:
C:> java Project2 dictionary.txt
Your Project2.java defines a small array of String. As your loop in main reads in Strings from the args[0] file, the dictionary, it appends them to the end of the array. When the array gets full a call is made to upSize the array and then resume appending Strings into it until the file is exhausted and all Strings are loaded into the array. You must fill in the upSize() method. Each time an upsize is performed, a line will be printed to the terminal indicating the new .length of the array.
As each string is read in you will update a counter of how many vowels in total have been found so far in all the strings. A vowel is 'a', 'e', 'i', 'o', or 'u'.
After the args[0] file is closed, main will call downSize() to shrink-wrap the array around the data so there are no empty cells at the end. You must fill in this method also. After downsizing the array main will print out the .length after downsizing and the wordCount and the vowelCount.
The last method main will call is findFirstDupe() which you must fill in. This method will search the array looking for the first occurrence of a String that is a duplicate of a previous String. Do not sort the array or alter the ordering of any of the strings inside the array. The method must return the String that is duplicated in the array. This string will be printed in main.
Starter file
/* Project2.java */ import java.io.*; import java.util.*; public class Project2 { static final int INITIAL_CAPACITY = 10; public static void main (String[] args) throws Exception { // ALWAYS TEST FIRST TO VERIFY USER PUT REQUIRED INPUT FILE NAME ON THE COMMAND LINE if (args.length < 1 ) { System.out.println(" usage: C:\\> java Project2 "); // i.e. C:\> java Project2 dictionary.txt System.exit(0); } String[] words = new String[INITIAL_CAPACITY]; int wordCount = 0; int vowelCount = 0; BufferedReader infile = new BufferedReader( new FileReader(args[0]) ); while ( infile.ready() ) { String word = infile.readLine(); // # # # # # DO NOT WRITE/MODIFY ANYTHING ABOVE THIS LINE # # # # # /* if words array is full { upsize the array System.out.println( "words.length after upSize: " + words.length ); // use this line } now append the word onto the end of the array and increment wordCount now examine every char in the word and every time you detect an a e i o or u, increment vowelCount */ // # # # # # DO NOT WRITE/MODIFY BELOW THIS LINE IN MAIN # # # # # } //END WHILE INFILE READY infile.close(); words = downSize( words, wordCount ); System.out.println( "After downSize() words.length=" + words.length + " wordCount=" + wordCount + " vowelCount=" + vowelCount ); System.out.println( "The duplicate word is: " + findFirstDupe( words, wordCount ) ); } // END main // RETURN AN ARRAY OF STRING 2X AS BIG AS ONE YOU PASSED IN // BE SURE TO COPY ALL THE OLD OWRDS OVER TO THE NEW ARRAY FIRST static String[] upSize( String[] fullArr ) { return null; } static String[] downSize( String[] arr, int count ) { return null; } static String findFirstDupe( String[] array, int count ) { // write a pair of nested loops that compare every stinrg to every other string // as soon as you fond two that are .equals to each other, immediately return that string return "NO DUPE FOUND IN ARRAY"; // LEAVE THIS HERE } } // END CLASS PROJECT#2
Thank you
Step by Step Solution
There are 3 Steps involved in it
Step: 1
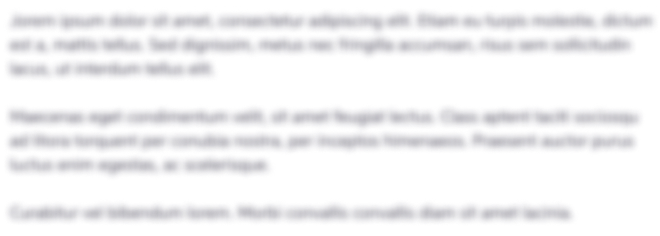
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started